How to Convert DataFrameGroupBy Object to DataFrame in Pandas
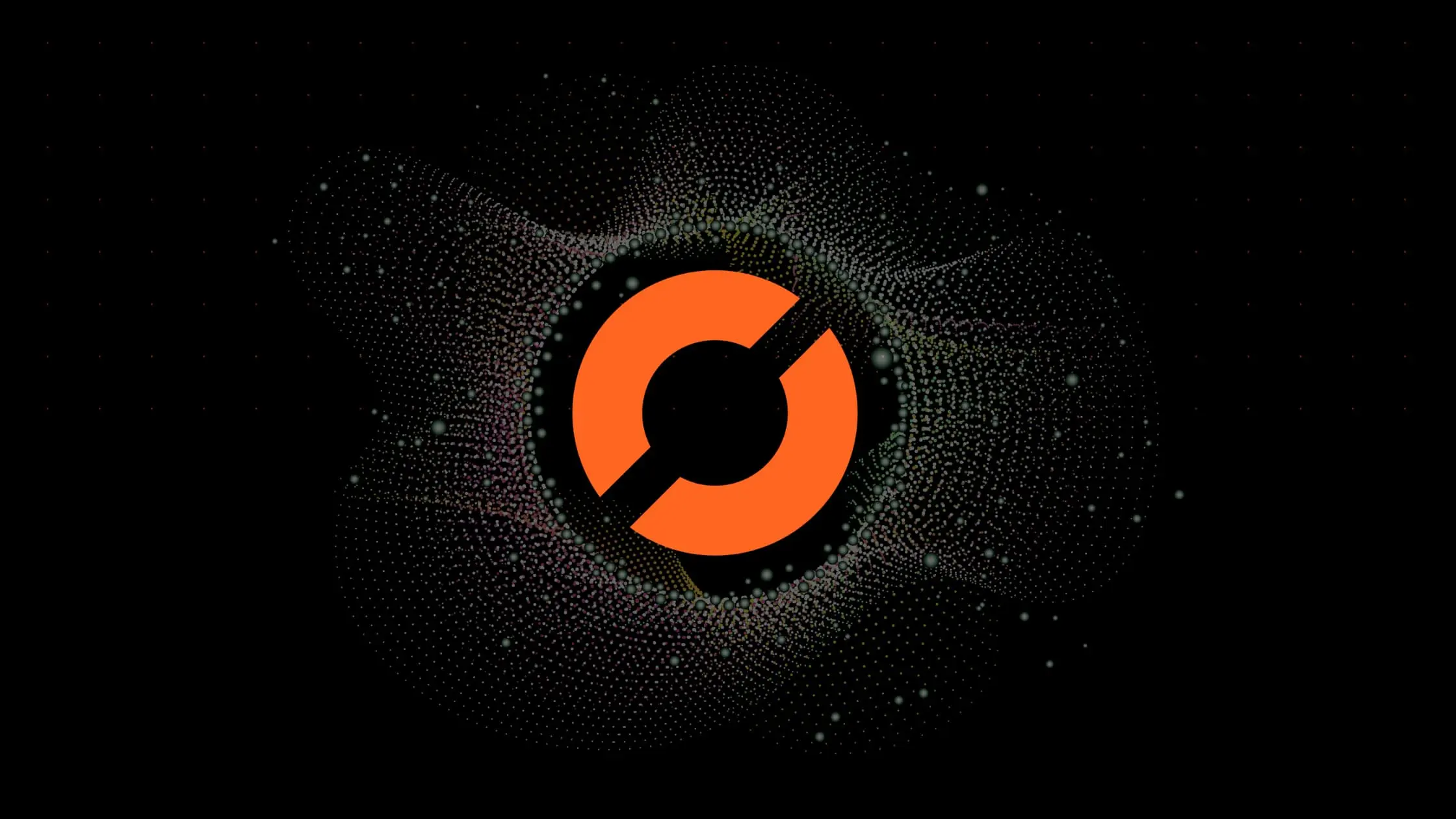
As a data scientist or software engineer, working with data is a crucial part of your job. Pandas is one of the most popular Python libraries for data manipulation and analysis. It provides a powerful DataFrame object that allows you to manipulate and analyze structured data easily. In some cases, you may need to group your data by certain columns and perform some operations on the groups. Pandas provides a handy groupby
function that allows you to do this. However, the resulting object is a DataFrameGroupBy
object, which may not be suitable for further analysis. In this blog post, we will show you how to convert a DataFrameGroupBy
object to a regular DataFrame
object in Pandas.
Table of Contents
- What is a DataFrameGroupBy Object?
- How to Convert a DataFrameGroupBy Object to DataFrame
- Common Errors and Solutions
- Conclusion
What is a DataFrameGroupBy Object?
Before we dive into the conversion process, let’s first understand what a DataFrameGroupBy
object is. When you apply the groupby
function on a DataFrame
object, Pandas returns a DataFrameGroupBy
object. This object has grouped the data based on one or more columns and is ready for further operations.
For example, let’s say you have a DataFrame
object that contains information about customers, their purchases, and the amount spent:
import pandas as pd
data = {
'customer': ['A', 'B', 'C', 'A', 'B', 'C'],
'purchase': ['book', 'pen', 'book', 'pen', 'book', 'pen'],
'amount': [10, 5, 15, 7, 12, 9]
}
df = pd.DataFrame(data)
If you want to group the data by the customer
column and get the total amount spent by each customer, you can use the groupby
function as follows:
grouped = df.groupby('customer')['amount'].sum()
print(grouped)
Output:
customer
A 17
B 17
C 24
Name: amount, dtype: int64
How to Convert a DataFrameGroupBy Object to DataFrame
To convert a DataFrameGroupBy
object to a regular DataFrame
object, you can use the reset_index
function. This function resets the index of the DataFrame
and returns a new DataFrame
object.
In our example above, we grouped the data by the customer
column and got the total amount spent by each customer. To convert the resulting DataFrameGroupBy
object to a regular DataFrame
, you can use the reset_index
function as follows:
df_new = grouped.reset_index()
The resulting df_new
object is a regular DataFrame
object that you can use for further analysis. You can confirm this by printing its type:
print(type(df_new))
Output:
pandas.core.frame.DataFrame
You can also print the df_new
object to see its contents:
print(df_new)
Output:
customer amount
0 A 17
1 B 17
2 C 24
As you can see, the df_new
object is a regular DataFrame
object that contains the grouped data.
Common Errors and Solutions
Error 1: Attempting to Access Columns Directly on DataFrameGroupBy Object
# Error
grouped = df.groupby('customer')['amount']
grouped['amount'].sum()
Error Explanation: Directly accessing a column on a DataFrameGroupBy
object will result in an error.
IndexError: Column(s) amount already selected
Solution:
# Solution
grouped = df.groupby('customer')['amount'].sum()
Error 2: Resetting Index Without Aggregation Function
# Error
df_new = df.groupby('customer').reset_index()
Error Explanation: Attempting to reset the index without an aggregation function will result in an error.
AttributeError: 'DataFrameGroupBy' object has no attribute 'reset_index'
Solution:
# Solution
df_new = df.groupby('customer')['amount'].sum().reset_index()
Conclusion
In this blog post, we have shown you how to convert a DataFrameGroupBy
object to a regular DataFrame
object in Pandas. The DataFrameGroupBy
object is created when you group your data using the groupby
function. It is a useful object for performing operations on groups of data. However, in some cases, you may need to convert this object to a regular DataFrame
object for further analysis. You can do this using the reset_index
function. We hope this blog post helps you in your data analysis tasks using Pandas.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.