How to Convert DataFrame to Dictionary in Pandas Without Index
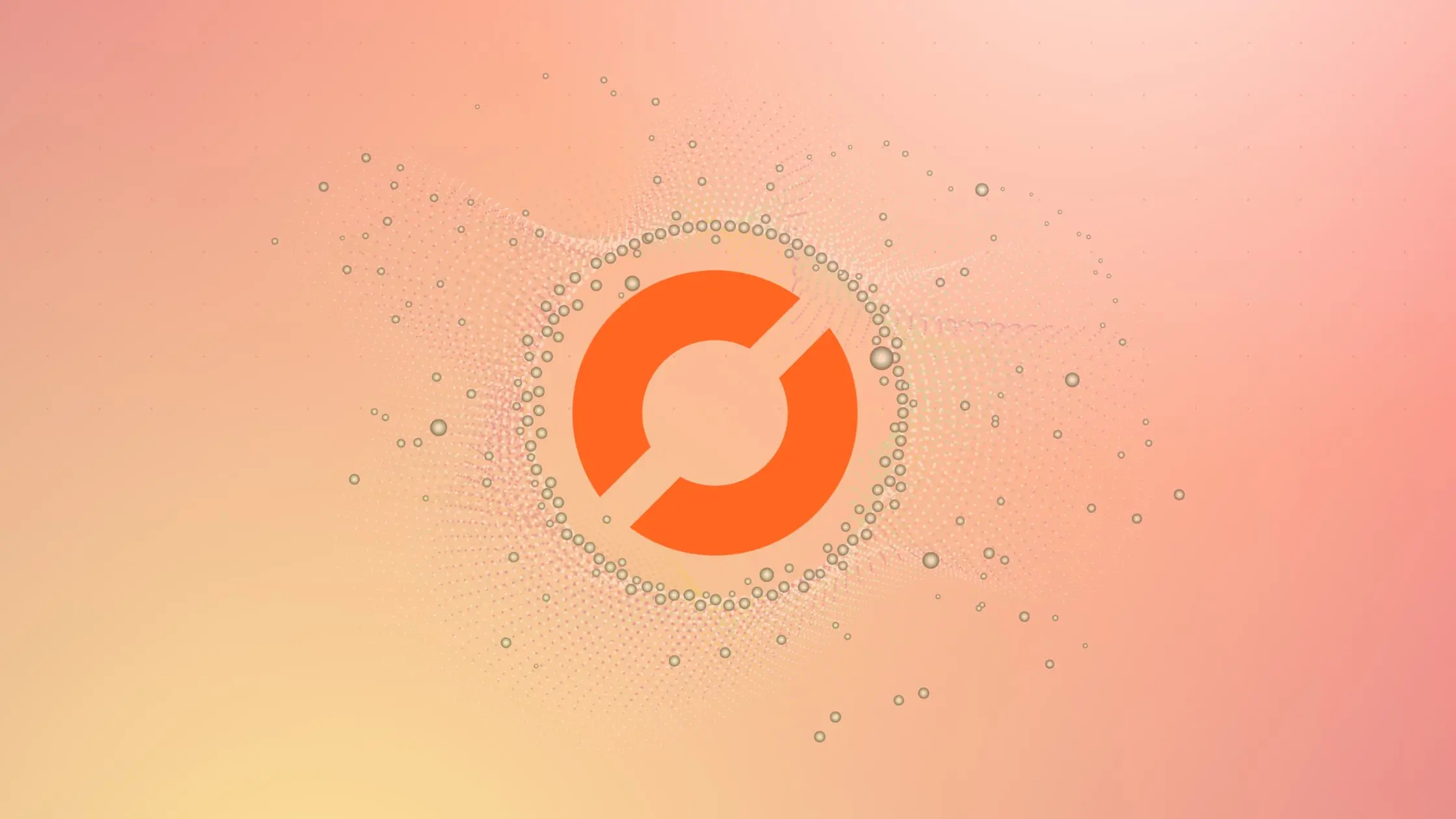
Pandas is a powerful data manipulation library in Python, widely used by data scientists for its robust and flexible data structures. One of these structures is the DataFrame, a two-dimensional tabular data structure with labeled axes. However, there are times when you might need to convert this DataFrame into a dictionary for easier manipulation or to feed into certain algorithms. In this blog post, we’ll explore how to convert a DataFrame to a dictionary without including the index.
Prerequisites
Before we dive in, ensure you have the following:
- Python installed (preferably Python 3.6 or later)
- Pandas library installed. If not, you can install it using pip:
pip install pandas
Creating a DataFrame
First, let’s create a simple DataFrame for our demonstration. We’ll use the pandas.DataFrame
function:
import pandas as pd
data = {'Name': ['John', 'Anna', 'Peter', 'Linda'],
'Age': [28, 24, 35, 32],
'City': ['New York', 'Paris', 'Berlin', 'London']}
df = pd.DataFrame(data)
This will create a DataFrame with ‘Name’, ‘Age’, and ‘City’ as columns.
Converting DataFrame to Dictionary Without Index
Pandas provides the to_dict()
function to convert a DataFrame into a dictionary. By default, this function includes the DataFrame’s index. However, we can modify this behavior by passing different arguments to the orient
parameter.
The orient
parameter determines the format of the resulting dictionary. Here are the possible values for orient
:
- ‘dict’ (default): keys are column names, values are dictionaries with index keys and corresponding values
- ‘list’: keys are column names, values are lists of column values
- ‘series’: keys are column names, values are Series of column values
- ‘split’: keys are ‘index’, ‘columns’, and ‘data’, values are corresponding arrays
- ‘records’: list of dictionaries with keys as column names and values as column values
- ‘index’: keys are index values, values are dictionaries with column names as keys and column values as values
To convert the DataFrame to a dictionary without the index, we can use the ‘list’ or ‘records’ option.
Using ‘list’ Orient
dict_list_orient = df.to_dict('list')
print(dict_list_orient)
This will output:
{'Name': ['John', 'Anna', 'Peter', 'Linda'],
'Age': [28, 24, 35, 32],
'City': ['New York', 'Paris', 'Berlin', 'London']}
Using ‘records’ Orient
dict_records_orient = df.to_dict('records')
print(dict_records_orient)
This will output:
[{'Name': 'John', 'Age': 28, 'City': 'New York'},
{'Name': 'Anna', 'Age': 24, 'City': 'Paris'},
{'Name': 'Peter', 'Age': 35, 'City': 'Berlin'},
{'Name': 'Linda', 'Age': 32, 'City': 'London'}]
Pros and Cons of Converting DataFrame to Dictionary Without Index:
Pros
Simplified Data Structure: The conversion allows for a more straightforward representation of tabular data, which can be advantageous for certain algorithms or data manipulation tasks that are more suited to dictionary structures.
Ease of Manipulation: Dictionaries in Python offer convenient methods for data manipulation and extraction. Converting a DataFrame to a dictionary without the index provides a format that is easy to work with programmatically.
Compatibility with Algorithms: Some algorithms and libraries may prefer or require input in dictionary format rather than a DataFrame. This conversion facilitates seamless integration with such algorithms, enhancing the compatibility of your data.
Reduced Memory Usage: In certain scenarios, dictionaries can be more memory-efficient than DataFrames. If memory constraints are a concern, converting to a dictionary without the index might be a favorable option.
Cons
Loss of Index Information: The index of a DataFrame contains valuable positional information. When converting to a dictionary without the index, this information is lost, which might be a drawback if the index is crucial for analysis or interpretation.
Limited Labeling: While dictionaries are effective for storing and retrieving data, they lack the column labels and data type information that DataFrames provide. This can make it less descriptive and potentially lead to ambiguity.
Potential Data Redundancy: Depending on the chosen orient parameter (‘list’ or ‘records’), there might be some redundancy in the generated dictionary, especially if multiple records share the same values for all columns.
Error Handling in Converting DataFrame to Dictionary:
Invalid Orient Parameter: Passing an invalid or unsupported value to the orient parameter can result in an error. Users should ensure that the chosen orient value (‘list’ or ‘records’) is appropriate for their use case.
Data Type Mismatch: The
to_dict()
function may encounter issues if the DataFrame contains mixed data types in a column. Ensuring consistent data types can help prevent unexpected errors during the conversion.Missing Data: If the DataFrame has missing or NaN values, the conversion to a dictionary might introduce complexities. Addressing missing data through preprocessing or handling is essential to maintain the integrity of the resulting dictionary.
Conclusion
Converting a DataFrame to a dictionary without the index is a straightforward process in Pandas, thanks to the to_dict()
function. Depending on your specific use case, you can choose the ‘list’ or ‘records’ orient to get a dictionary without the index.
Remember, the power of Pandas lies in its flexibility. So, don’t hesitate to explore different options and find the one that best suits your needs. Happy data wrangling!
References
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.