How to Convert CSV File to Pandas DataFrame
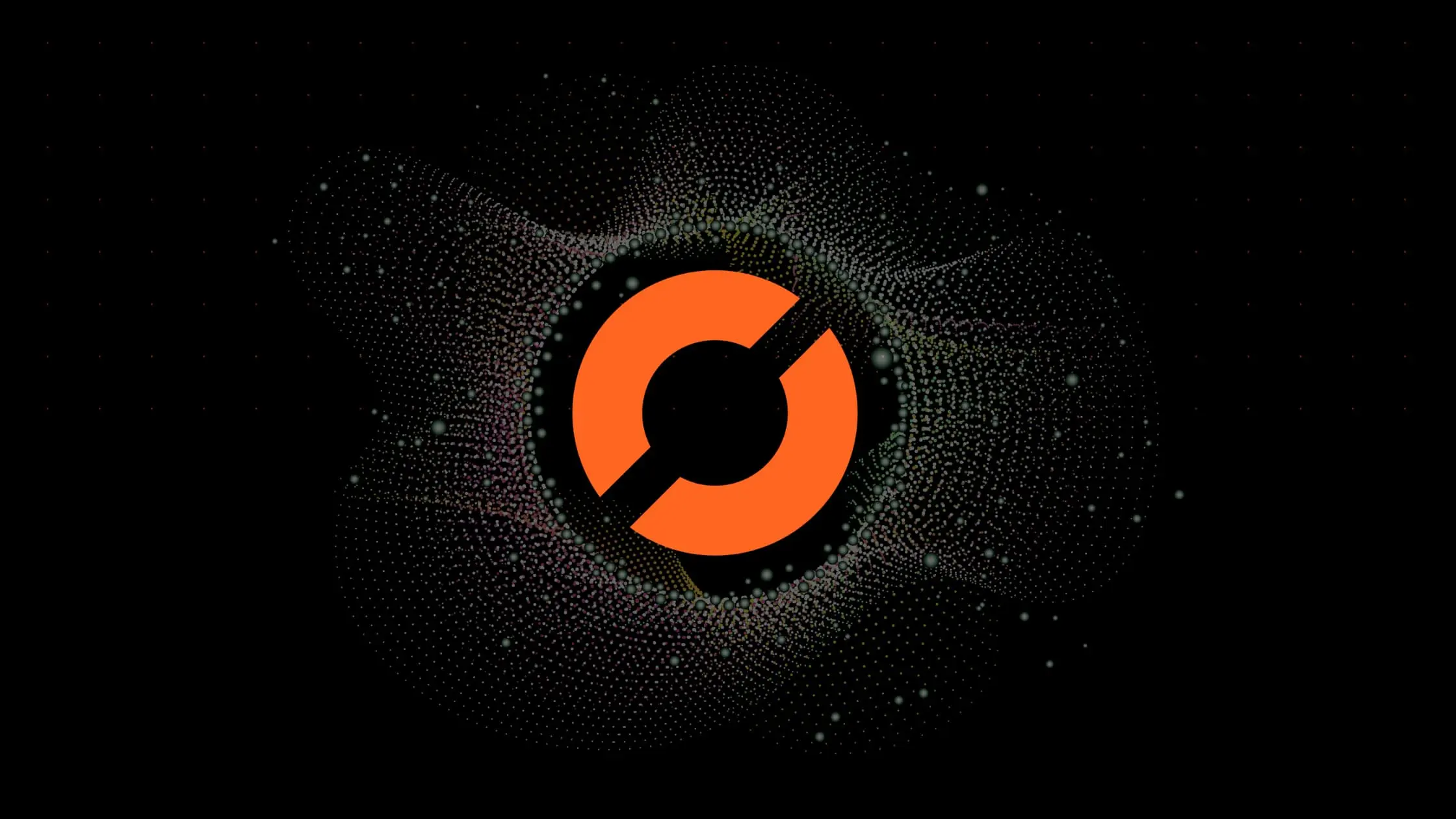
As a data scientist or software engineer, you might frequently encounter the need to work with data stored in CSV (Comma-Separated Values) files. CSV files are a popular file format for storing and exchanging tabular data, as they are easy to read and write, and can be easily imported into various tools and applications. One of the most powerful tools for working with tabular data in Python is the Pandas library. In this article, we will explain how to convert a CSV file to a Pandas DataFrame, step-by-step.
Table of Contents
- Prerequisites
- Methods for Converting CSV to Pandas DataFrame
- Pros and Cons Comparison
- Common Errors and How to Handle Them
- Conclusion
Prerequisites
Before we dive into the actual process of converting a CSV file to a Pandas DataFrame, you need to ensure that you have the following prerequisites:
- Python 3.x installed on your system
- Pandas library installed on your system
- A CSV file containing the data that you want to convert to a Pandas DataFrame
Methods for Converting CSV to Pandas DataFrame
Using pd.read_csv()
import pandas as pd
# Method 1: Using pd.read_csv()
df = pd.read_csv('your_file.csv')
Using csv.reader
and Lists
import csv
import pandas as pd
# Method 2: Using csv.reader and Lists
with open('your_file.csv', 'r') as file:
reader = csv.reader(file)
data = list(reader)
df = pd.DataFrame(data, columns=data[0])
Using numpy
and Arrays
import numpy as np
import pandas as pd
# Method 3: Using numpy and Arrays
data = np.genfromtxt('your_file.csv', delimiter=',', dtype=None, names=True)
df = pd.DataFrame(data)
Pros and Cons Comparison
Method | Pros | Cons |
---|---|---|
pd.read_csv() | - Simple and concise | - May not handle all edge cases |
csv.reader and Lists | - Provides fine-grained control over the conversion | - Requires additional code for data cleanup |
numpy and Arrays | - Efficient for large datasets | - Limited flexibility in data types |
Common Errors and How to Handle Them
Missing Values
Dealing with missing values is a common challenge when working with real-world datasets. The pd.read_csv()
method provides a convenient way to handle missing values during the conversion process. You can use the na_values
parameter to specify which values should be treated as missing. Here’s an example:
import pandas as pd
# Specify missing values using na_values
df = pd.read_csv('your_file.csv', na_values=['NA', 'N/A', '-'])
# Alternatively, handle missing values explicitly after reading the CSV
df = pd.read_csv('your_file.csv')
df.dropna(inplace=True)
In this example, the na_values
parameter is set to a list of strings that should be treated as missing values. You can customize this list based on the specific representations of missing values in your CSV file.
Delimiter Mismatch
Mismatched delimiters can lead to incorrect parsing of CSV files. It’s crucial to ensure that the delimiter specified in your code matches the actual delimiter used in the CSV file. The pd.read_csv()
method allows you to explicitly set the delimiter using the delimiter
or sep
parameter. Here’s an example:
import pandas as pd
# Specify the delimiter using the delimiter parameter
df = pd.read_csv('your_file.csv', delimiter=';')
# Alternatively, use the sep parameter
df = pd.read_csv('your_file.csv', sep=';')
In this example, the delimiter is set to a semicolon (;). Adjust the delimiter according to the structure of your CSV file.
Encoding Issues
Encoding issues can arise when the default encoding used by pd.read_csv()
doesn’t match the encoding of your CSV file. To address encoding problems, use the encoding
parameter to explicitly specify the encoding. Common encodings include ‘utf-8’, ‘latin-1’, and ‘ISO-8859-1’. Here’s an example:
import pandas as pd
# Specify the encoding using the encoding parameter
df = pd.read_csv('your_file.csv', encoding='utf-8')
# Alternatively, try different encodings based on your file
df = pd.read_csv('your_file.csv', encoding='latin-1')
Conclusion
In this article, we have explained how to convert a CSV file to a Pandas DataFrame in Python. We have covered the basic steps involved in the process, including importing the Pandas library, reading the CSV file, exploring the data, and saving the DataFrame back to a CSV file. By following these steps, you can easily work with tabular data stored in CSV files using the powerful features provided by the Pandas library.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.