How to Convert Columns to String in Pandas
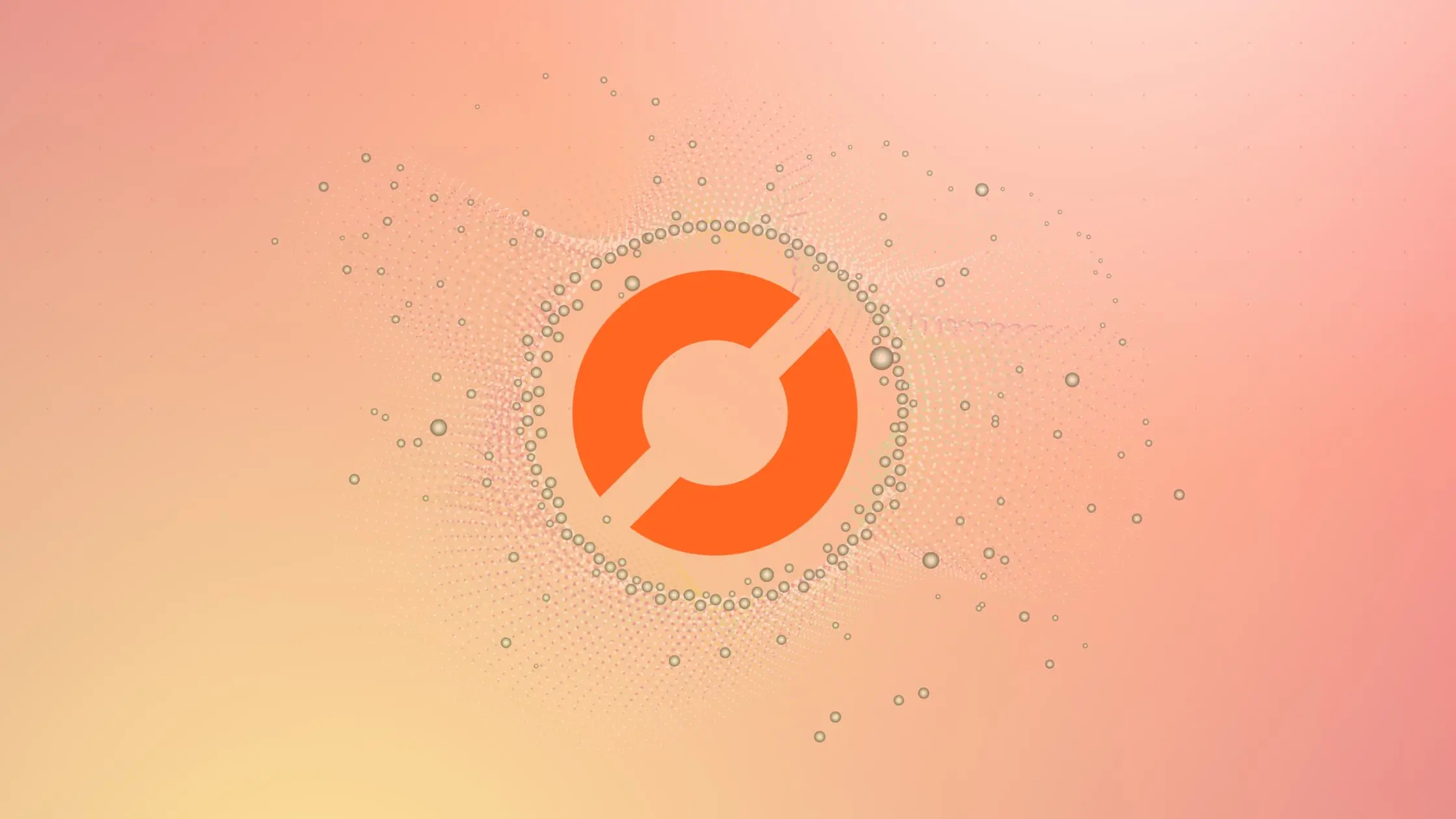
As a data scientist or software engineer, you may come across many situations where you need to convert columns to string in Pandas. In this article, we will explain how to do this with Python and Pandas.
What is Pandas?
Pandas is an open-source data manipulation library for Python. It provides data structures for efficiently storing and manipulating large datasets. Pandas is built on top of NumPy and provides easy-to-use data analysis tools.
Why do we need to convert columns to string in Pandas?
There are many reasons why we might need to convert columns to string in Pandas. One of the most common reasons is when we are working with data that has mixed data types. For example, we might have a column that contains both numeric and string data types. In this case, it can be difficult to perform certain operations on the data, such as sorting or grouping.
Another reason why we might need to convert columns to string in Pandas is when we want to concatenate two or more columns. In this case, we need to convert each column to a string before we can concatenate them.
How to convert columns to string in Pandas
Let assume that we have the following DataFrame.
import pandas as pd
# Creating a DataFrame
data = {'employee_id': [101, 102, 103],
'name': ['Alice', 'Bob', 'Charlie'],
'age': [28, 35, 42],
'salary':[50000, 60000, 75000],
'experience':[2, 5, 8]}
df = pd.DataFrame(data)
# Displaying the DataFrame
print(df)
OUTPUT:
employee_id name age salary experience
0 101 Alice 28 50000 2
1 102 Bob 35 60000 5
2 103 Charlie 42 75000 8
To convert columns to string in Pandas, we can use the astype()
method. This method allows us to convert a column to a specified data type.
Let’s say we have a Pandas DataFrame df
that contains a column named employee_id
that we want to convert to a string. We can use the following code to do this:
# Converting 'employee_id' to string
df['employee_id'] = df['employee_id'].astype(str)
# Displaying the types of data after conversion
print("\nTypes of data after conversion:\n", df.dtypes)
OUTPUT:
Types of data after conversion:
employee_id object
name object
age int64
salary int64
experience int64
dtype: object
This code will convert the employee_id
column to a string data type. We can then use this column for further analysis or manipulation.
We can also convert multiple columns to string at once by passing a list of column names to the astype()
method. For example, if we have two columns named salary
and experience
, we can convert them to string data types using the following code:
# Converting 'salary' and 'experience' to string
df[['salary', 'experience']] = df[['salary', 'experience']].astype(str)
# Displaying the types of data after conversion
print("\nTypes of data after conversion:\n", df.dtypes)
This code will convert both salary
and experience
to string data types:
Types of data after conversion
employee_id object
name object
age int64
salary object
experience object
dtype: object
Conclusion
In this article, we have explained how to convert columns to string in Pandas using Python. We have also discussed why we might need to do this and provided examples of how to convert single and multiple columns to string data types.
By converting columns to string in Pandas, we can perform certain operations on the data that would otherwise be difficult or impossible. We hope that this article has been helpful in explaining how to do this.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.