How to Convert a PyTorch Tensor into a NumPy Array: A Comprehensive Guide
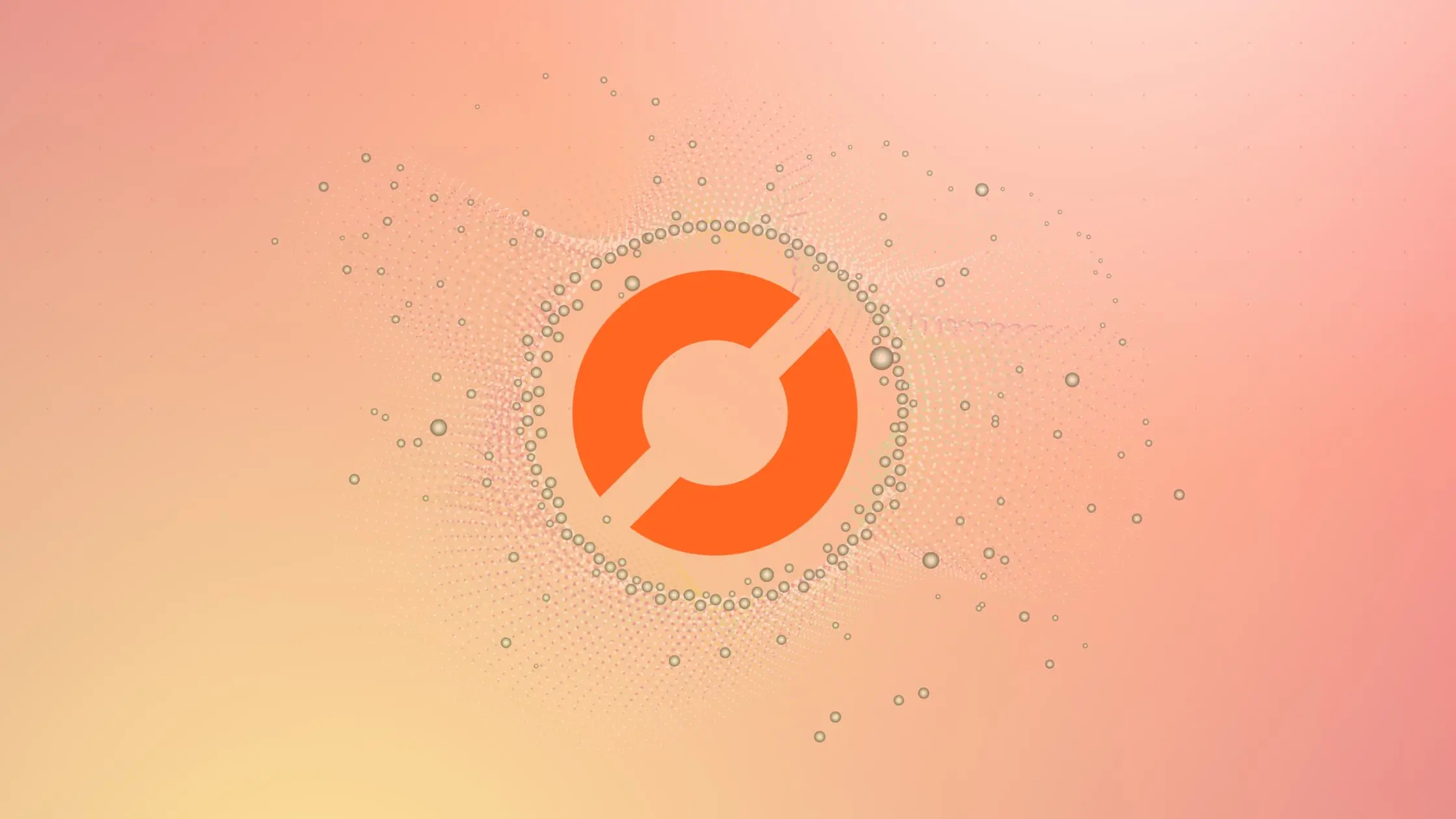
Data scientists often need to switch between different data types and formats. One common conversion is from PyTorch tensors to NumPy arrays. This blog post will guide you through the process, step by step.
Introduction
PyTorch and NumPy are two powerful libraries for data scientists. PyTorch is a popular open-source machine learning library, while NumPy is a library for the Python programming language, adding support for large, multi-dimensional arrays and matrices, along with a large collection of high-level mathematical functions to operate on these arrays.
There are times when you might need to convert a PyTorch tensor into a NumPy array. For instance, you might want to leverage the computational capabilities of PyTorch while using the versatility and functionality of NumPy for data manipulation and analysis. This comprehensive guide will walk you through the process of converting a PyTorch tensor into a NumPy array, providing insights into the advantages, potential challenges, and common errors encountered by data scientists. Optimize your data flow and elevate your workflow with this step-by-step conversion guide.
Bridging PyTorch and NumPy:
Converting PyTorch Tensors to NumPy Arrays: A Simple Recipe
Step 1: Import the Necessary Libraries
The first step is to import the necessary libraries. You will need both PyTorch and NumPy for this task.
import torch
import numpy as np
Step 2: Create a PyTorch Tensor
Next, you need to create a PyTorch tensor. This can be done using the torch.tensor()
function. Here’s an example:
# Create a PyTorch tensor
tensor = torch.tensor([[1, 2, 3], [4, 5, 6]])
print(tensor)
Step 3: Convert the PyTorch Tensor to a NumPy Array
Now that you have a PyTorch tensor, you can convert it into a NumPy array using the .numpy()
method. This method returns the tensor as a NumPy ndarray object.
# Convert the tensor to a NumPy array
numpy_array = tensor.numpy()
print(numpy_array)
Step 4: Verify the Conversion
Finally, you should verify that the conversion was successful. You can do this by checking the type of the resulting object.
# Check the type of the resulting object
print(type(numpy_array))
If the conversion was successful, the output should be <class 'numpy.ndarray'>
.
Common Errors and Troubleshooting:
Data Type Compatibility:
To prevent potential issues, confirm that the data types of your PyTorch tensor and NumPy array align perfectly. If necessary, explicitly set the data type to ensure a smooth conversion process.
GPU to CPU Transfer:
When dealing with GPU tensors, optimize your workflow by transferring data to the CPU using the to('cpu')
method before initiating the conversion to a NumPy array. This proactive step minimizes potential hiccups and ensures a seamless transition.
Empty Tensors:
Avoid the conversion of empty tensors, as this practice may result in unpredictable behavior and errors. Prioritize working with tensors containing meaningful data for a smoother conversion experience.
Conclusion
Converting a PyTorch tensor into a NumPy array is a straightforward process. It involves creating a PyTorch tensor, converting the tensor to a NumPy array using the .numpy()
method, and then verifying the conversion.
This conversion is useful in many scenarios, such as when you want to leverage the computational capabilities of PyTorch while using the versatility and functionality of NumPy for data manipulation and analysis.
Remember that the .numpy()
method returns a NumPy array that shares the same memory as the PyTorch tensor. Therefore, changes to the original tensor will affect the NumPy array and vice versa.
We hope this guide has been helpful. Stay tuned for more posts on data science and machine learning!
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.