How to Convert a List to a Pandas Dataframe Column
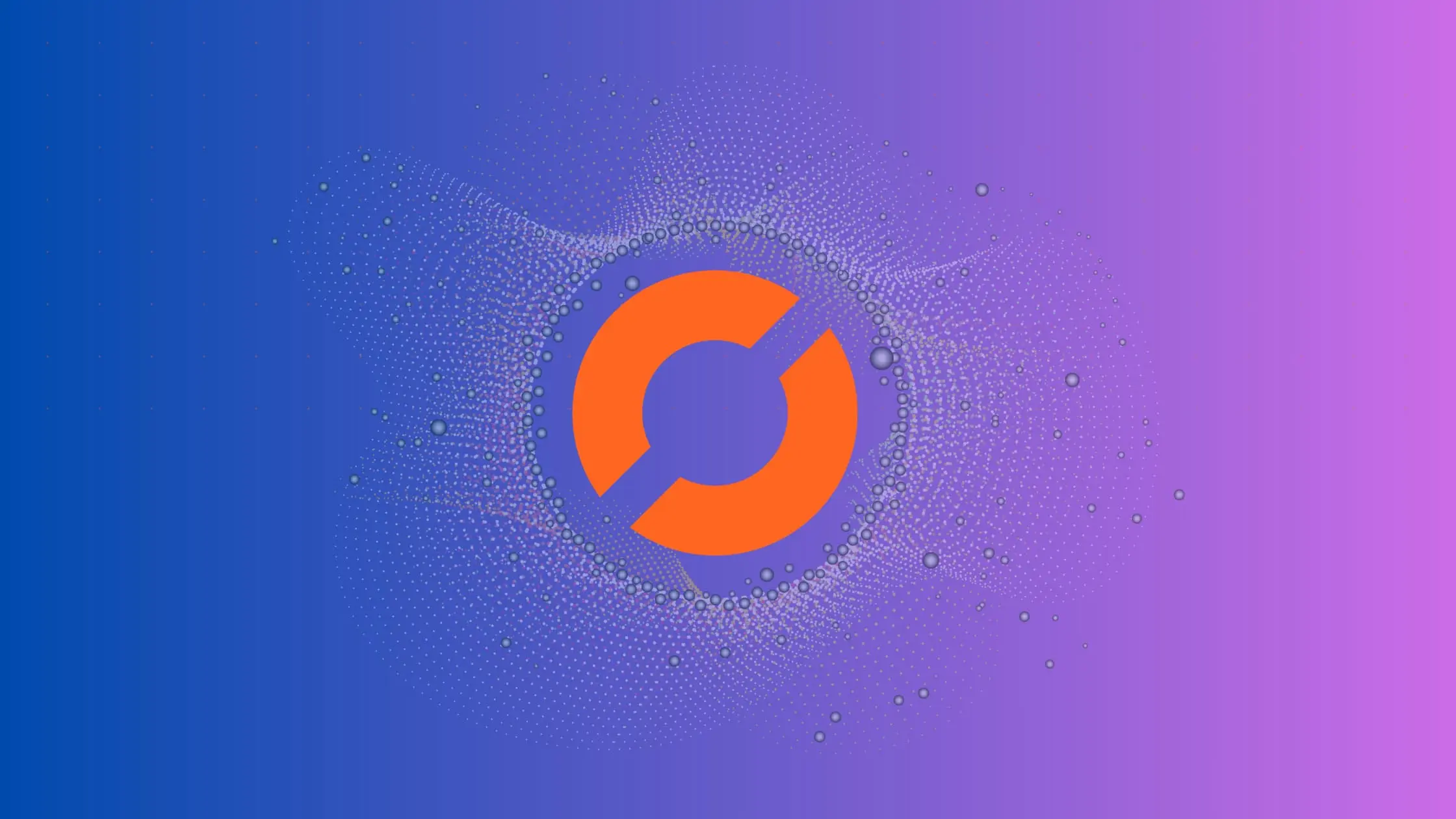
As a data scientist or software engineer, you may find yourself working with data in a variety of formats, including lists. While lists are a useful way to store data, they can be difficult to work with and analyze. One popular tool for data analysis is the Pandas library, which provides a range of powerful data manipulation and analysis functions. In this article, we will explore how to convert a list to a Pandas dataframe column, a common task in data analysis.
Table of Contents
- What is a Pandas Dataframe?
- Converting a List to a Pandas Dataframe Column
- Converting Multiple Lists to Pandas Dataframe Columns
- Common Errors and Solutions
- Conclusion
What is a Pandas Dataframe?
Before we dive into the details of converting a list to a Pandas dataframe column, let’s first define what a Pandas dataframe is. A Pandas dataframe is a two-dimensional, size-mutable, and heterogeneous tabular data structure with labeled axes (rows and columns). It is similar to a spreadsheet or SQL table, but with more powerful processing capabilities.
Pandas dataframes are widely used in data analysis because they provide a flexible and efficient way to manipulate and analyze data. They can handle data in a variety of formats, including CSV, Excel, SQL databases, and more. In addition, Pandas provides a wide range of functions for data cleaning, transformation, and analysis, making it a powerful tool for data scientists and analysts alike.
Converting a List to a Pandas Dataframe Column
Now that we have a basic understanding of what a Pandas dataframe is, let’s look at how to convert a list to a Pandas dataframe column. This is a common task in data analysis, as you may have data stored in a list that you want to analyze using Pandas.
The easiest way to convert a list to a Pandas dataframe column is to use the pandas.DataFrame()
function. This function takes a dictionary as input, where the keys are the column names and the values are the data for each column. We can create a dictionary with a single key-value pair, where the key is the column name and the value is the list we want to convert to a dataframe column.
Here’s an example:
import pandas as pd
my_list = [1, 2, 3, 4, 5]
df = pd.DataFrame({'my_column': my_list})
print(df)
This will output:
my_column
0 1
1 2
2 3
3 4
4 5
As you can see, we have successfully converted our list to a Pandas dataframe column named “my_column”. This approach works well when you have a single list of data to convert.
Converting Multiple Lists to Pandas Dataframe Columns
In some cases, you may have multiple lists of data that you want to convert to a Pandas dataframe. In this case, you can create a dictionary with multiple key-value pairs, where each key is the column name and each value is a list of data for that column.
Here’s an example:
import pandas as pd
my_list1 = [1, 2, 3, 4, 5]
my_list2 = ['a', 'b', 'c', 'd', 'e']
df = pd.DataFrame({'column1': my_list1, 'column2': my_list2})
print(df)
This will output:
column1 column2
0 1 a
1 2 b
2 3 c
3 4 d
4 5 e
As you can see, we have successfully converted two lists to Pandas dataframe columns named “column1” and “column2”. This approach works well when you have multiple lists of data to convert.
Common Errors and Solutions
Error 1: List and Dictionary Length Mismatch
Error Code:
import pandas as pd
my_list = [1, 2, 3, 4, 5]
df = pd.DataFrame({'column1': my_list, 'column2': [10, 20, 30]})
Solution:
Ensure that all lists used in the dictionary have the same length. In the above example, make sure both my_list
and the list provided for ‘column2’ have the same number of elements.
Error 2: Undefined Column Name
Error Code:
import pandas as pd
my_list = [1, 2, 3, 4, 5]
df = pd.DataFrame({my_list: [10, 20, 30, 40, 50]})
Solution: Ensure that the column name provided in the dictionary is a valid string. In the above example, use a string as the column name.
Conclusion
In this article, we have explored how to convert a list to a Pandas dataframe column. We have seen that this is a common task in data analysis, and that it can be easily accomplished using the pandas.DataFrame()
function. We have also seen how to convert multiple lists to Pandas dataframe columns using a dictionary with multiple key-value pairs.
Pandas dataframes are a powerful tool for data analysis, and being able to convert data from various formats, including lists, is an essential skill for any data scientist or software engineer. By following the steps outlined in this article, you should now be able to easily convert lists to Pandas dataframe columns and start analyzing your data with Pandas.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.