How to Confirm Equality of Two Pandas DataFrames
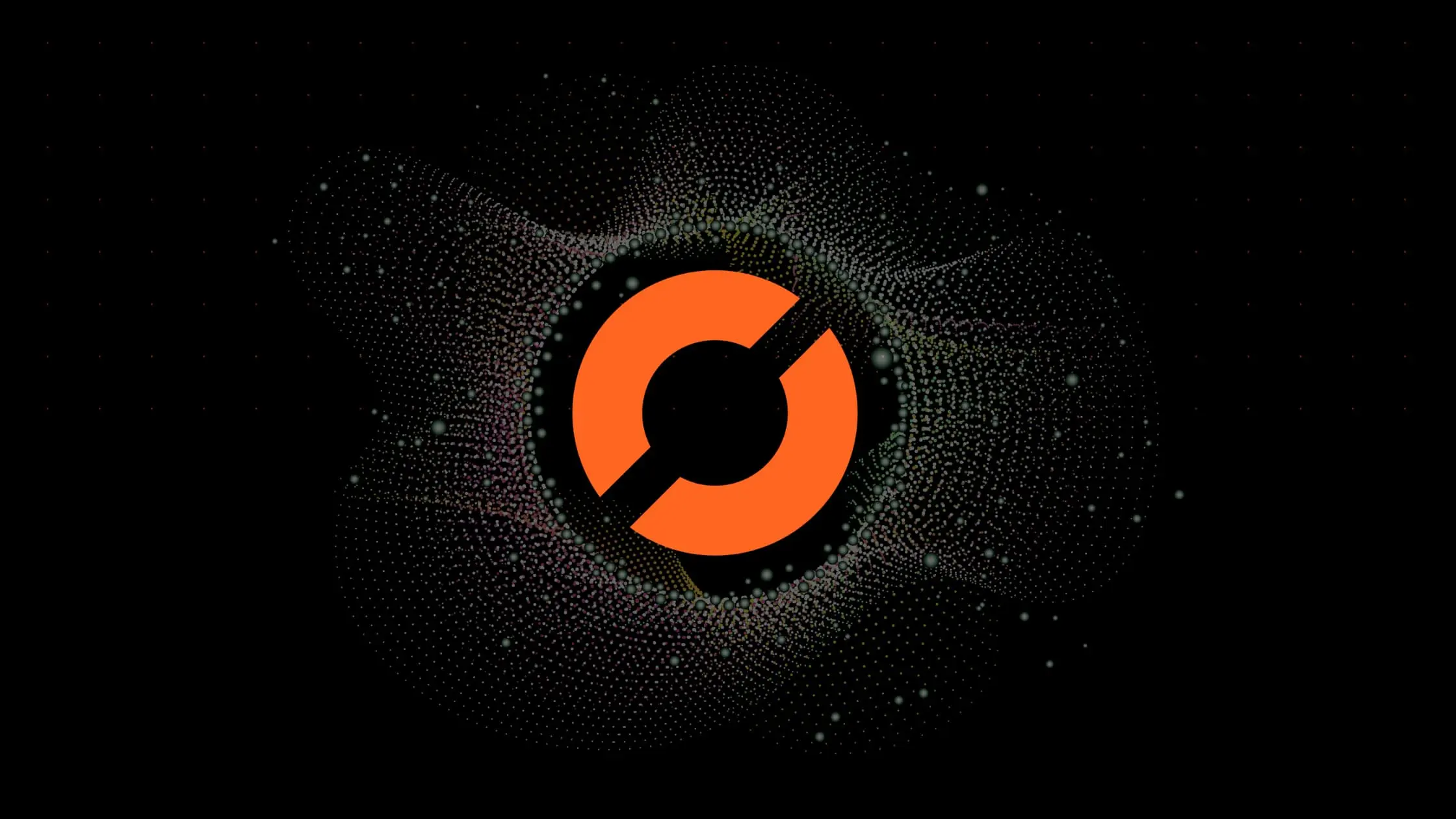
As a data scientist or software engineer, you may need to compare two pandas DataFrames to confirm their equality. This can be a common task when working with large datasets, and it’s important to ensure that the data is consistent between two different sources.
In this article, we will explore the various methods of confirming the equality of two pandas DataFrames. We will also discuss some of the potential issues that may arise during this process and how to avoid them.
Table of Contents
- What is a Pandas DataFrame?
- How to Compare Two Pandas DataFrames
- Potential Issues When Comparing Pandas DataFrames
- Conclusion
What is a Pandas DataFrame?
Before we dive into the specifics of comparing two pandas DataFrames, let’s first discuss what a pandas DataFrame is. A DataFrame is a two-dimensional, size-mutable, tabular data structure with rows and columns, similar to a spreadsheet or a SQL table. Pandas is a popular Python library used for data manipulation and analysis, and it provides a DataFrame class as one of its primary data structures.
A DataFrame can be created from a variety of sources, including CSV files, Excel spreadsheets, SQL databases, and other pandas DataFrames. Once you have a DataFrame, you can perform various operations on it, such as filtering, sorting, grouping, and aggregating data.
How to Compare Two Pandas DataFrames
When comparing two pandas DataFrames, there are several methods that you can use to confirm their equality. Let’s explore each of these methods in detail.
Method 1: Using the equals() Method
The simplest method of comparing two pandas DataFrames is to use the equals() method. This method returns True if the two DataFrames are equal and False otherwise. Here’s an example:
import pandas as pd
df1 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df2 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
if df1.equals(df2):
print("The two DataFrames are equal")
else:
print("The two DataFrames are not equal")
In this example, we create two identical DataFrames, df1 and df2, and compare them using the equals() method. Since the DataFrames are identical, the output will be "The two DataFrames are equal"
.
Method 2: Using the compare() Method
Another method of comparing two pandas DataFrames is to use the compare() method. This method returns a DataFrame containing the differences between the two DataFrames. If the DataFrames are equal, the resulting DataFrame will be empty.
Here’s an example:
import pandas as pd
df1 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df2 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 7]})
diff = df1.compare(df2)
if diff.empty:
print("The two DataFrames are equal")
else:
print("The two DataFrames are not equal")
print(diff)
In this example, we create two DataFrames, df1 and df2, with a single difference in the B column of df2. We compare the DataFrames using the compare() method, which returns a DataFrame containing the differences between the two DataFrames. Since the DataFrames are not equal, the output will be "The two DataFrames are not equal"
, followed by the differences between the two DataFrames.
Output:
The two DataFrames are not equal
B
self other
2 6.0 7.0
Method 3: Using the all() Method
The all() method can also be used to compare two pandas DataFrames. This method returns True if all the elements in the two DataFrames are equal and False otherwise.
Here’s an example:
import pandas as pd
df1 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df2 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
if (df1 == df2).all().all():
print("The two DataFrames are equal")
else:
print("The two DataFrames are not equal")
In this example, we create two identical DataFrames, df1 and df2, and compare them using the all() method. The expression (df1 == df2) returns a DataFrame of True and False values, indicating whether each element in the two DataFrames is equal. The all() method is then called twice to check whether all the elements in the resulting DataFrame are True. Since all the elements are True, the output will be "The two DataFrames are equal"
.
Method 4: Using the assert_frame_equal() Function
Finally, we can use the assert_frame_equal() function from the pandas.testing module to compare two pandas DataFrames. This function raises an AssertionError if the two DataFrames are not equal.
Here’s an example:
import pandas as pd
from pandas.testing import assert_frame_equal
df1 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df2 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 7]})
assert_frame_equal(df1, df2)
In this example, we create two DataFrames, df1 and df2, with a single difference in the B column of df2. We compare the DataFrames using the assert_frame_equal() function, which raises an AssertionError since the DataFrames are not equal.
Output:
AssertionError: DataFrame.iloc[:, 1] (column name="B") are different
DataFrame.iloc[:, 1] (column name="B") values are different (33.33333 %)
[index]: [0, 1, 2]
[left]: [4, 5, 6]
[right]: [4, 5, 7]
Potential Issues When Comparing Pandas DataFrames
When comparing two pandas DataFrames, there are some potential issues that you should be aware of. These issues can arise due to differences in the data types, column names, or row indexes of the two DataFrames.
Issue 1: Different Data Types
If the two DataFrames have columns with different data types, the equals() method may return False even if the actual values in the columns are the same. The compare() method and the all() method may also return unexpected results in this case.
To avoid this issue, it’s important to ensure that the data types of the columns in the two DataFrames are the same before comparing them.
Issue 2: Different Column Names
If the two DataFrames have columns with different names, the equals() method and the all() method may return False even if the actual values in the columns are the same. The compare() method may also return unexpected results in this case.
To avoid this issue, you can use the rename() method to rename the columns in one of the DataFrames to match the column names in the other DataFrame before comparing them.
Issue 3: Different Row Indexes
If the two DataFrames have different row indexes, the equals() method and the all() method may return False even if the actual values in the columns are the same. The compare() method may also return unexpected results in this case.
To avoid this issue, you can use the reset_index() method to reset the row indexes of both DataFrames before comparing them.
Conclusion
In this article, we explored the various methods of confirming the equality of two pandas DataFrames. We also discussed some of the potential issues that may arise during this process and how to avoid them.
By using the methods outlined in this article, you can ensure that your data is consistent between two different sources. This can be particularly important when working with large datasets, where small discrepancies can have a significant impact on your analysis.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.