How to Compare Multiple Column Values Using Pandas
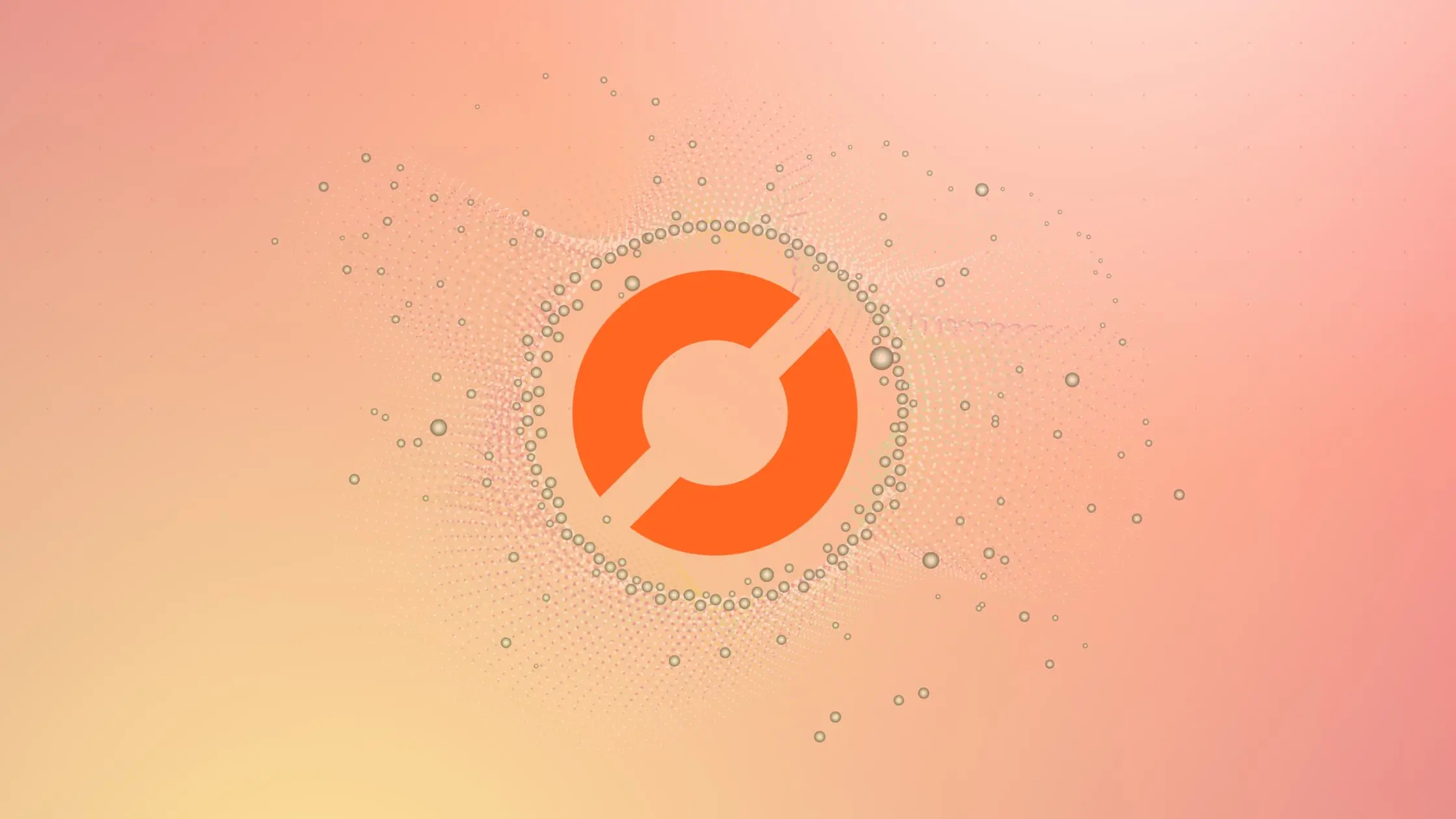
As a data scientist or software engineer, you may need to compare multiple column values in a dataset to gain insights into the data. This task can be achieved using Pandas, which is a popular data manipulation library in Python. In this article, we will explore how to compare multiple column values using Pandas.
What is Pandas?
Pandas is an open-source data manipulation library in Python that provides easy-to-use data structures and data analysis tools. It is built on top of the NumPy library and is used for data cleaning, data analysis, and data visualization.
Comparing Multiple Column Values using Pandas
To compare multiple column values in Pandas, we can use the DataFrame
class, which is a two-dimensional table-like data structure with rows and columns. We can compare the values of two or more columns using various operators such as equality (==
), inequality (!=
), greater than (>
), less than (<
), greater than or equal to (>=
), and less than or equal to (<=
).
Let’s consider the following example dataset:
import pandas as pd
# Sample data for product prices in 2021, 2022, and 2023
data = {'Product': ['Laptop', 'Smartphone', 'Tablet', 'Smartwatch', 'Headphones'],
'Price_2021': [1300, 850, 420, 260, 150],
'Price_2022': [1200, 800, 350, 250, 150],
'Price_2023': [1100, 750, 380, 240, 150]}
product_prices = pd.DataFrame(data)
This creates a DataFrame with three columns (Porduct, Price_2022, and Price_2023) and five rows of data.
Comparing Two Columns
To compare two columns of a DataFrame, we can use the equality (==
) operator to check if the values in the two columns are the same. For example, if we want to compare the Price_2022 and Price_2023 columns, we can use the following code:
# Comparing two columns (Price_2022 and Price_2023)
price_comparison = product_prices['Price_2022'] > product_prices['Price_2023']
print(price_comparison)
This will return a Boolean Series with True
where the values in the Price_2022 and Price_2023 columns are the same, and False
where they are not.
Output:
0 True
1 True
2 False
3 True
4 False
dtype: bool
Comparing Multiple Columns
To compare multiple columns of a DataFrame, we can use the all()
method along with the equality eq()
method. For example, if we want to compare the Price_2021, Price_2022, and Price_2023 columns, we can use the following code:
# Comparing multiple columns
comparison_result = (product_prices[['Price_2021', 'Price_2022', 'Price_2023']].eq(product_prices['Price_2021'], axis=0)).all(axis=1)
# Print the result
print("Comparison Result:")
print(comparison_result)
Output:
Comparison Result:
0 False
1 False
2 False
3 False
4 True
dtype: bool
This will return True
if all the values in the Price_2021, Price_2022, and Price_2023 columns are the same, and False
otherwise.
Filtering Data based on Column Comparisons
We can also use the comparison operations to filter the rows of a DataFrame based on the values in multiple columns. For example, if we want to filter the rows where the Price_2022 is greater than Price_2023 and the Product column contains the word “Smart” , we can use the following code:
# Comparing prices and product names
price_comparison = product_prices['Price_2022'] > product_prices['Price_2023']
product_name_comparison = product_prices['Product'].str.contains('Smart')
# Filtering data based on column comparisons
filtered_data = product_prices[price_comparison & product_name_comparison]
print(filtered_data)
Output:
Product Price_2021 Price_2022 Price_2023
1 Smartphone 850 800 750
3 Smartwatch 260 250 240
This code snippet creates two conditions (price_comparison and product_name_comparison) to filter rows where ‘Price_2022’ is greater than ‘Price_2023’ and the ‘Product’ column contains the word “Smart”. The resulting DataFrame, filtered_data, will contain only the rows that satisfy both conditions.
Conclusion
Comparing multiple column values in a DataFrame is a common task in data analysis. In this article, we have explored how to compare column values using Pandas. We have seen how to compare two columns using the equality operator and how to compare multiple columns using the all()
method. We have also seen how to filter data based on column comparisons. With these techniques, you can gain insights into your data and make better decisions based on the results of your analysis.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.