How to Combine Two Columns in a Pandas DataFrame
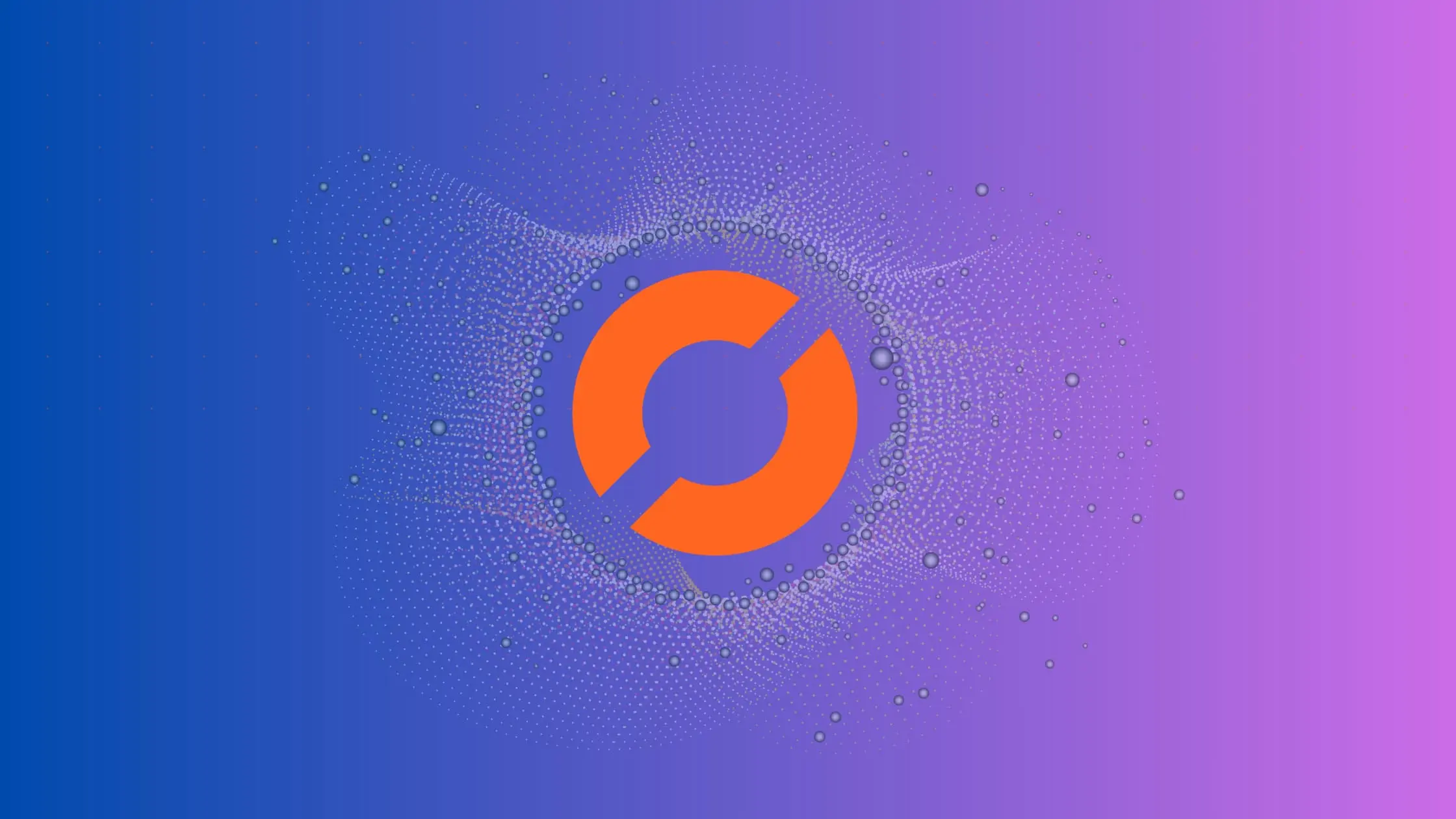
As a data scientist or software engineer, you are likely familiar with the powerful data manipulation library, pandas. One common task that arises when working with pandas is the need to combine two columns in a DataFrame. In this article, we will explore several methods for combining columns in pandas and discuss the pros and cons of each approach.
What is pandas?
Before we dive into the specifics of combining columns in pandas, let’s first discuss what pandas is and why it is such a valuable tool for data scientists and software engineers. Pandas is an open-source data manipulation library for Python that provides a wide range of functions for working with structured data. It is built on top of NumPy, another popular Python library for scientific computing, and provides several key data structures, including the Series and DataFrame objects.
How to Combine Two Columns in a Pandas DataFrame
There are several methods for combining two columns in a pandas DataFrame, each with its own advantages and disadvantages. Let’s explore some of the most common approaches.
Method 1: Using the + operator
One simple way to combine two columns in a pandas DataFrame is to use the +
operator. This approach is straightforward and easy to implement, but it has some limitations. Here’s an example:
import pandas as pd
# Create a sample DataFrame
data = {'Column1': [1, 2, 3],
'Column2': ['A', 'B', 'C']}
df = pd.DataFrame(data)
# Combine 'Column1' and 'Column2' into a new column 'Combined'
df['Combined'] = df['Column1'].astype(str) + df['Column2']
This will produce the following output:
Column1 Column2 Combined
0 1 A 1A
1 2 B 2B
2 3 C 3C
As you can see, the +
operator simply adds together the values in the two columns and creates a new column with the combined values. However, this approach has some limitations. For example, if either column contains missing values (NaN)
, the resulting column will also contain missing values. Additionally, if either column contains non-numeric data, the +
operator will raise an error.
Method 2: Using the .apply()
method
Another approach to combining columns in pandas is to use the .apply()
method. This method allows you to apply a custom function to each row or column of a DataFrame, which can be useful for more complex data manipulation tasks. Here’s an example:
import pandas as pd
# Create a sample DataFrame
data = {'Column1': [1, 2, 3],
'Column2': ['A', 'B', 'C']}
df = pd.DataFrame(data)
# Define a custom function to combine columns
def combine_columns(row):
return str(row['Column1']) + row['Column2']
# Apply the custom function to create a new column 'Combined'
df['Combined'] = df.apply(combine_columns, axis=1)
This will produce the same output as the previous example:
Column1 Column2 Combined
0 1 A 1A
1 2 B 2B
2 3 C 3C
While this approach requires a bit more code than the previous example, it is more flexible and can handle missing or non-numeric data more gracefully.
Method 3: Using agg() to Concat String Columns of DataFrame
You can achieve the concatenation of multiple string columns by utilizing the DataFrame.agg() method. As shown in the previous example, you can provide a list of the columns you wish to concatenate as the argument.
import pandas as pd
# Create a sample DataFrame
data = {'Column1': [1, 2, 3],
'Column2': ['A', 'B', 'C']}
df = pd.DataFrame(data)
df["Column1"] = df["Column1"].astype(str)
# Apply the custom function to create a new column 'Combined'
df['Combined'] = df[['Column1', 'Column2']].agg(''.join, axis=1)
This will generate an output identical to the previous example, but it’s crucial to ensure that the two columns on which this function is applied have consistent data types.:
Column1 Column2 Combined
0 1 A 1A
1 2 B 2B
2 3 C 3C
Conclusion
In this article, we explored several methods for combining two columns in a pandas DataFrame, including using the +
operator, the .apply()
method, and the .agg()
method. While each approach has its own advantages and disadvantages, the method you choose will depend on the specific requirements of your data manipulation task. By understanding these different approaches, you can become a more effective data scientist or software engineer and take full advantage of the powerful pandas library.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.