How to Clear GPU Memory After PyTorch Model Training Without Restarting Kernel
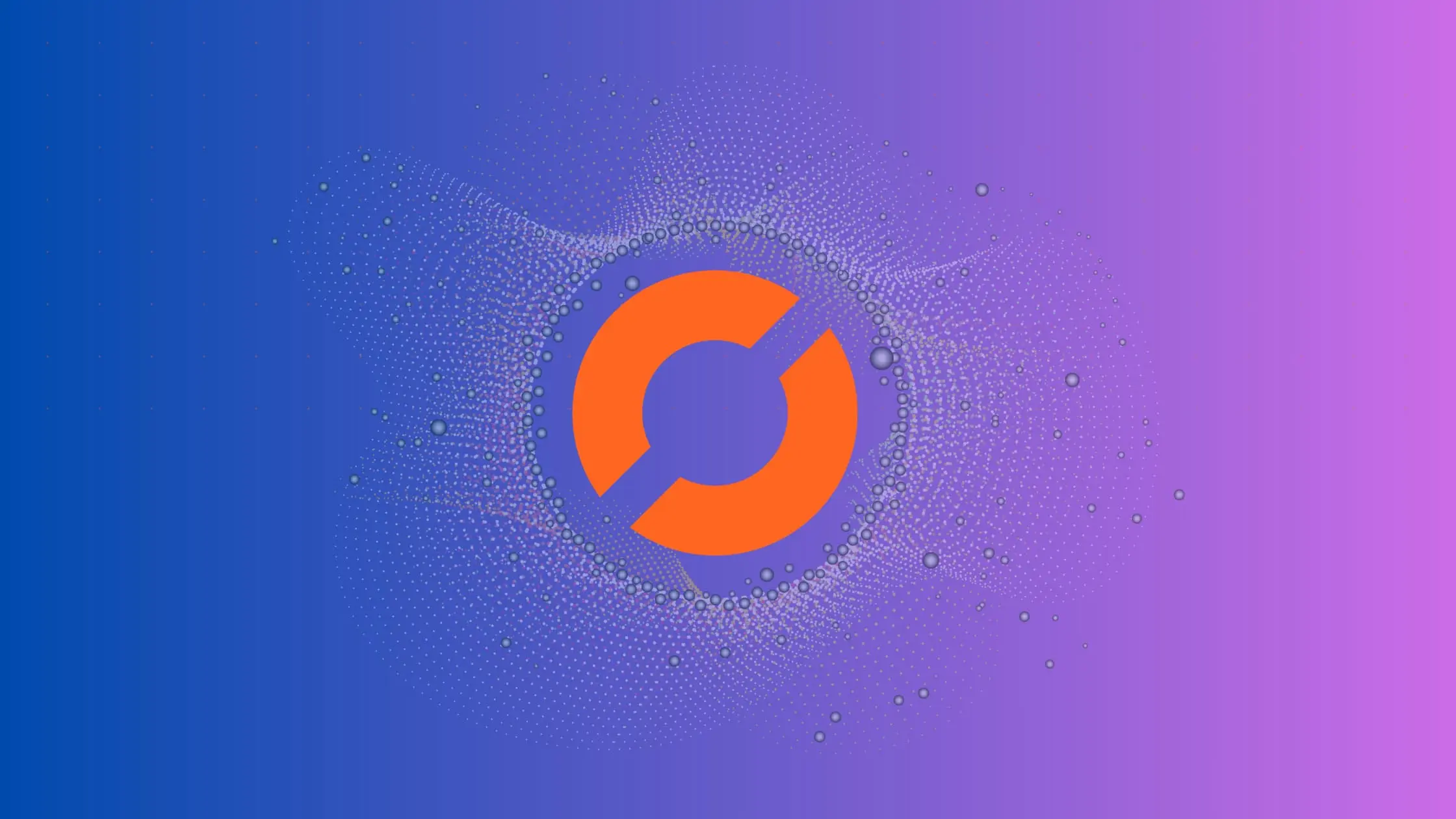
As a data scientist or software engineer, you may have encountered situations where you need to train PyTorch models on large datasets using a GPU. GPUs are ideal for deep learning tasks as they can perform parallel computations faster than CPUs. However, training models on a GPU can quickly fill up its memory, leading to memory errors and reduced performance. In this post, we’ll explore how to clear GPU memory after PyTorch model training without restarting the kernel.
Table of Contents
The Problem with GPU Memory
Before we dive into the solution, let’s first understand why GPU memory can become an issue during PyTorch model training. GPUs have limited memory resources, and as such, they can only hold a certain amount of data at a time. When training deep learning models, the model’s parameters, activations, and gradients are stored in the GPU memory. As the model trains, the memory usage increases, and if it reaches the limit, the GPU will run out of memory, leading to memory errors.
The Solution: Clearing GPU Memory
To prevent memory errors and optimize GPU usage during PyTorch model training, we need to clear the GPU memory periodically. There are several ways to clear GPU memory, and we’ll explore them below.
Method 1: Empty Cache
PyTorch provides a built-in function called empty_cache()
that releases all the GPU memory that can be freed. This function is useful when you want to release all the memory that is no longer needed but still held by the cache.
Here’s how to use empty_cache()
:
import torch
torch.cuda.empty_cache()
This function releases all the memory that can be freed. However, it doesn’t guarantee that all the memory will be freed since some memory may still be held by references. Therefore, you may need to call this function multiple times to ensure that all the memory is released.
Method 2: Del Variables
Another way to clear GPU memory is by deleting the variables that are no longer needed. When a variable is deleted, its memory is freed and can be used by other variables.
Here’s an example:
import torch
# Define a tensor
x = torch.randn(1000, 1000).cuda()
# Use the tensor
y = x * 2
# Delete the tensor
del x
# Use the GPU memory for other variables
z = y * 3
In this example, we defined a tensor x
and used it to compute y
. After using x
, we deleted it using the del
keyword, which freed its memory. We then used the freed memory to compute z
.
Method 3: Set Variables to None
Similar to deleting variables, setting variables to None
can also release their memory. However, unlike deleting variables, setting variables to None
doesn’t immediately free their memory. Instead, the memory is freed by Python’s garbage collector, which runs periodically.
Here’s an example:
import torch
# Define a tensor
x = torch.randn(1000, 1000).cuda()
# Use the tensor
y = x * 2
# Set the tensor to None
x = None
# Use the GPU memory for other variables
z = y * 3
In this example, we defined a tensor x
and used it to compute y
. After using x
, we set it to None
, which marked it for garbage collection. We then used the freed memory to compute z
.
Method 4: Use Context Manager
Finally, we can use a context manager to clear GPU memory automatically. A context manager is a Python construct that allows you to define a block of code that is executed before and after another block of code. In this case, we can define a context manager that clears the GPU memory before and after PyTorch model training.
Here’s an example:
import torch
class ClearCache:
def __enter__(self):
torch.cuda.empty_cache()
def __exit__(self, exc_type, exc_val, exc_tb):
torch.cuda.empty_cache()
# Use the context manager
with ClearCache():
# Define and train the PyTorch model
...
In this example, we defined a context manager called ClearCache
that calls empty_cache()
before and after the block of code it surrounds. We then used the context manager to define and train a PyTorch model.
Conclusion
In this post, we explored how to clear GPU memory after PyTorch model training without restarting the kernel. We discussed why GPU memory can become an issue during PyTorch model training and explored four methods to clear GPU memory: empty_cache()
, deleting variables, setting variables to None
, and using a context manager. By using these methods, you can prevent memory errors and optimize GPU usage during PyTorch model training.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.