How to Check Whether Your Code is Running on the GPU or CPU
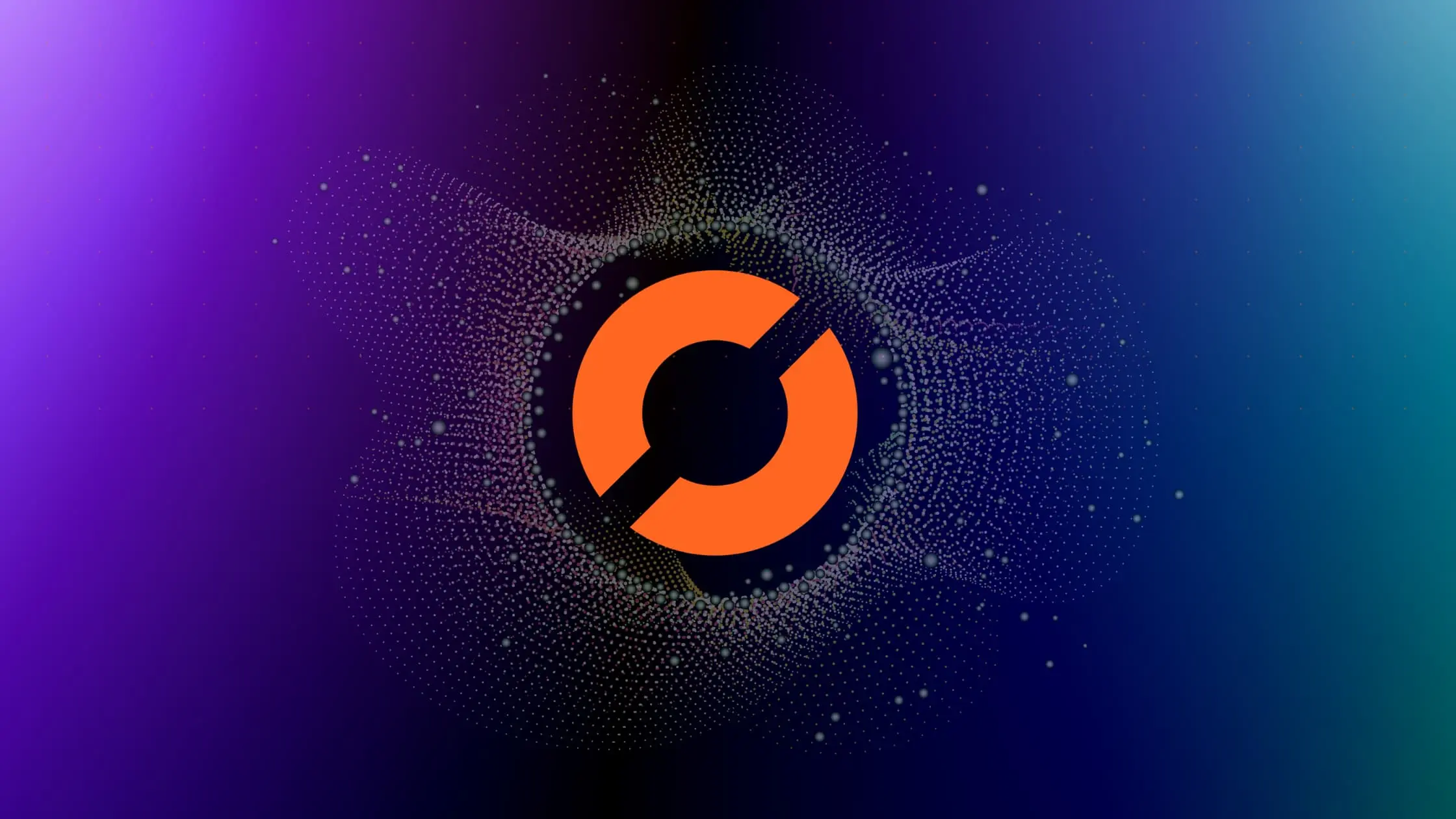
As a data scientist or software engineer, it’s important to know whether your code is running on the GPU or CPU. Running code on the GPU can significantly speed up computation times, but it’s not always clear whether your code is actually running on the GPU or not.
In this post, we’ll go over how to check whether your code is running on the GPU or CPU, and how to make sure it’s running on the GPU if it’s not.
Table of Contents
- What is the GPU?
- Checking Whether Your Code is Running on the GPU
- Common Errors and Solutions
- Conclusion
What is the GPU?
Before we delve into checking whether your code is running on the GPU or CPU, let’s briefly discuss what the GPU is. The GPU, or Graphics Processing Unit, is a specialized processor designed to handle the complex calculations required for rendering graphics. In recent years, GPUs have also become popular for general-purpose computing tasks due to their ability to perform many calculations in parallel.
Checking Whether Your Code is Running on the GPU
There are a few different ways to check whether your code is running on the GPU or CPU, depending on what programming language and libraries you’re using.
Python
If you’re using Python and the PyTorch library, you can check whether your code is running on the GPU by using the torch.cuda.is_available()
function. This function returns True
if a GPU is available and False
otherwise.
import torch
if torch.cuda.is_available():
print("GPU is available")
else:
print("GPU is not available")
To ensure that your PyTorch code is running on the GPU, you can use the .to()
method to move your tensors to the GPU.
import torch
if torch.cuda.is_available():
device = torch.device("cuda")
else:
device = torch.device("cpu")
x = torch.randn(10, 10).to(device)
This will create a tensor x
that is located on the GPU if one is available, and on the CPU otherwise.
R
If you’re using R and the tensorflow
library, you can check whether your code is running on the GPU by using the tf$test_gpu_available()
function. This function returns TRUE
if a GPU is available and FALSE
otherwise.
library(tensorflow)
if (tf$test_gpu_available()) {
print("GPU is available")
} else {
print("GPU is not available")
}
If you want to make sure that your TensorFlow code is actually running on the GPU, you can use the tf$device()
function to specify the device.
library(tensorflow)
if (tf$test_gpu_available()) {
device <- "/gpu:0"
} else {
device <- "/cpu:0"
}
with(tf$device(device), {
# Your TensorFlow code here
})
This will run your TensorFlow code on the GPU if one is available, and on the CPU otherwise.
MATLAB
If you’re using MATLAB and the Parallel Computing Toolbox, you can check whether your code is running on the GPU by using the gpuDeviceCount
function. This function returns the number of available GPUs.
num_gpus = gpuDeviceCount;
if num_gpus > 0
disp("GPU is available")
else
disp("GPU is not available")
end
If you want to make sure that your MATLAB code is actually running on the GPU, you can use the gpuArray()
function to create a GPU array.
if gpuDeviceCount > 0
x = gpuArray(rand(10, 10));
else
x = rand(10, 10);
end
This will create a matrix x
that is located on the GPU if one is available, and on the CPU otherwise.
Common Errors and Solutions
Error: No CUDA-capable device is detected.
Solution: Ensure that you have a compatible NVIDIA GPU and have installed the appropriate CUDA toolkit.
Error: TensorFlow not installed in R
Solution: Install TensorFlow in R using install.packages("tensorflow")
.
Error: GPU not found
Solution: Ensure your machine has a compatible GPU and TensorFlow can access it.
Error: Error using gpuDevice: No supported GPU device was found.
Solution: Ensure that you have a compatible GPU and have installed the necessary drivers.
Conclusion
In this post, we’ve gone over how to check whether your code is running on the GPU or CPU, and how to make sure it’s running on the GPU if it’s not. Depending on what programming language and libraries you’re using, you may need to use different functions or methods to check for the availability of the GPU and to move your data to the GPU. By following these steps, you can ensure that your code is running as efficiently as possible, and take advantage of the power of the GPU to speed up your computations.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.