How to Check if Column Value is in Other Columns in Pandas
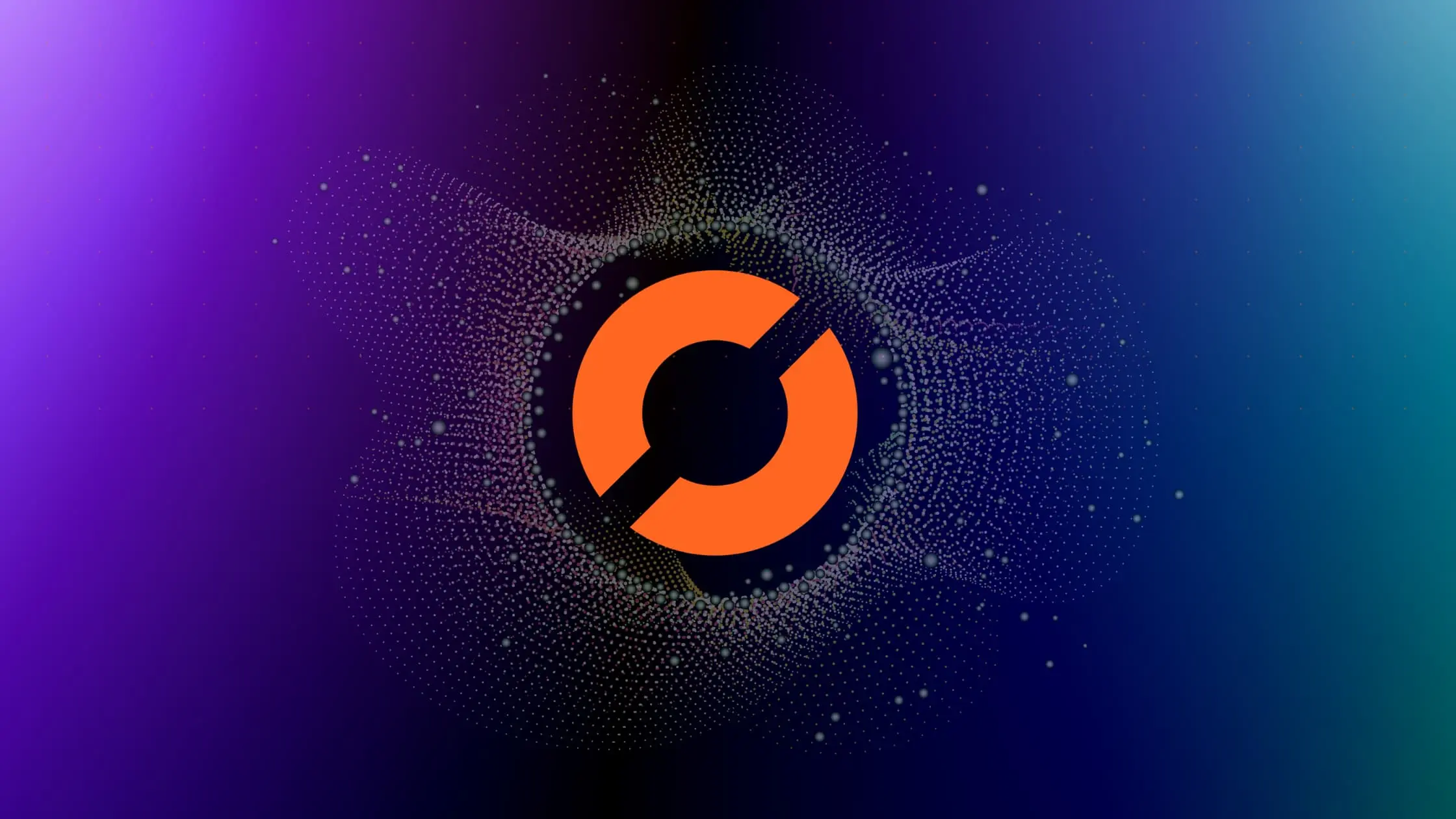
As a data scientist or software engineer, you may come across a situation where you need to check if a column value exists in other columns of the same dataframe. This can be a useful technique when you need to filter or manipulate your data based on certain conditions.
In this blog post, we will explore different ways to check if a column value exists in other columns of a pandas dataframe. We will also discuss the advantages and disadvantages of each method.
Table of Contents
Understanding the Data
Before we dive into the methods, let’s first understand the data we will be working with. For this example, we will use a dataframe that contains information about movies.
import pandas as pd
data = {'Title': ['The Shawshank Redemption', 'The Godfather', 'The Dark Knight', 'Pulp Fiction', 'The Lord of the Rings: The Return of the King'],
'Director': ['Frank Darabont', 'Francis Ford Coppola', 'Christopher Nolan', 'Quentin Tarantino', 'Peter Jackson'],
'Year': [1994, 1972, 2008, 1994, 2003],
'Rating': [9.3, 9.2, 9.0, 8.9, 8.9],
'Genre': ['Drama', 'Crime', 'Action', 'Crime', 'Adventure']}
df = pd.DataFrame(data)
This dataframe contains information about the title, director, year, rating, and genre of each movie. Our goal is to check if the movie genre is also present in the title or director columns.
Method 1: Using the isin Method
The first method we will explore is using the isin
method of the pandas dataframe. This method returns a boolean mask indicating whether each element of the dataframe is contained in the specified sequence.
df['Genre'].isin(df['Title']) | df['Genre'].isin(df['Director'])
This code creates a boolean mask that checks if the genre column is present in either the title or director columns. The |
operator is used to combine the two boolean masks using logical OR.
The advantage of using the isin
method is that it is a very simple and straightforward way to check if a column value exists in other columns. However, this method can only check if the column value exists in one other column at a time. If you need to check if the column value exists in multiple columns, you will need to use another method.
Pros
- Simple and straightforward.
- Easy to implement.
- Suitable for checking if a column value exists in one other column at a time.
Cons
- Limited to checking in one other column at a time.
Method 2: Using the apply Method
The second method we will explore is using the apply
method of the pandas dataframe. This method applies a function along an axis of the dataframe.
df.apply(lambda x: x['Genre'] in [x['Title'], x['Director']], axis=1)
This code applies a lambda function to each row of the dataframe. The lambda function checks if the genre column is present in either the title or director columns. The axis=1
parameter specifies that the function should be applied to each row.
The advantage of using the apply
method is that it can check if the column value exists in multiple columns at the same time. However, this method can be slower than the isin
method for large dataframes.
Pros
- Can check if a column value exists in multiple columns simultaneously.
Cons
- May be slower for large dataframes.
- Code may be less readable compared to the isin method.
Method 3: Using the any Method
The third method we will explore is using the any
method of the pandas dataframe. This method returns a boolean mask indicating whether any element of the dataframe is True along a specified axis.
df[['Title', 'Director']].apply(lambda x: x.str.contains(df['Genre'].iloc[0], regex=False).any(), axis=1)
This code first selects the title and director columns of the dataframe using df[['Title', 'Director']]
. The lambda function then applies the str.contains
method to each element of the title and director columns to check if the genre column is present in either column. The any
method is then used to check if any element of the resulting boolean mask is True.
The advantage of using the any
method is that it is a fast and efficient way to check if a column value exists in multiple columns at the same time. However, this method can be more complex to implement than the previous methods.
Pros
- Efficient for checking if a column value exists in multiple columns simultaneously.
Cons
- Code complexity might be higher than the isin method.
General Considerations
Size of Dataframe:
- For small dataframes, simplicity may be prioritized, favoring the isin method.
- For large dataframes, efficiency becomes more critical, and the any method might be preferred despite its complexity.
Code Complexity:
- If code readability is crucial, the isin method might be preferred.
- If performance is a higher priority, the any method could be chosen.
Error Handling
- Handling NaN Values: When dealing with NaN values, it’s crucial to handle them appropriately to avoid unexpected behavior during comparisons.
# Example: Fill NaN values with a placeholder value
df.fillna('NA', inplace=True)
- Axis Specification in Apply Method: When using the apply method, it’s essential to specify the correct axis to ensure the function is applied row-wise.
# Example: Applying a function row-wise using axis=1
df.apply(lambda x: your_function(x), axis=1)
- Case Sensitivity in String Comparison: String comparison using the str.contains method is case-sensitive by default. Specify case=False for case-insensitive matching if needed.
# Example: Using case-insensitive string comparison
df['Column'].str.contains('value', case=False)
- Bitwise OR for Combining Boolean Masks: When combining boolean masks, use the bitwise OR (|) instead of the logical OR (or) to avoid errors.
# Example: Using bitwise OR to combine boolean masks
df['Column'].isin(another_df['AnotherColumn']) | df['Column'].isin(yet_another_df['YetAnotherColumn'])
- Data Types Mismatch: Ensure that the data types of the columns being compared are compatible. Convert columns to the desired data type if needed.
# Example: Convert column to the desired data type
df['Column'] = df['Column'].astype('desired_type')
- Memory Usage for Large Dataframes: For large dataframes, consider selecting specific columns instead of working with the entire dataframe to reduce memory usage.
# Example: Selecting specific columns to reduce memory usage
df_subset = df[['Column1', 'Column2']]
Conclusion
In this blog post, we explored three different methods to check if a column value exists in other columns of a pandas dataframe. The isin
method is a simple and straightforward way to check if a column value exists in one other column at a time. The apply
method can check if a column value exists in multiple columns at the same time, but can be slower for large dataframes. The any
method is a fast and efficient way to check if a column value exists in multiple columns at the same time, but can be more complex to implement.
When choosing a method, it is important to consider the size of your dataframe and the complexity of your code. By understanding the advantages and disadvantages of each method, you can choose the one that best fits your needs.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.