How to Change the Default Threshold for Classification in sklearn LogisticRegression
LogisticRegression
algorithm from the widely used Python library scikit-learn
(sklearn) proves inadequate for specific use cases. Delving into the significance of thresholds and their impact on model performance, we will explore why it matters. Additionally, we will discuss practical techniques to modify these thresholds, offering insights into enhancing the effectiveness of machine learning models.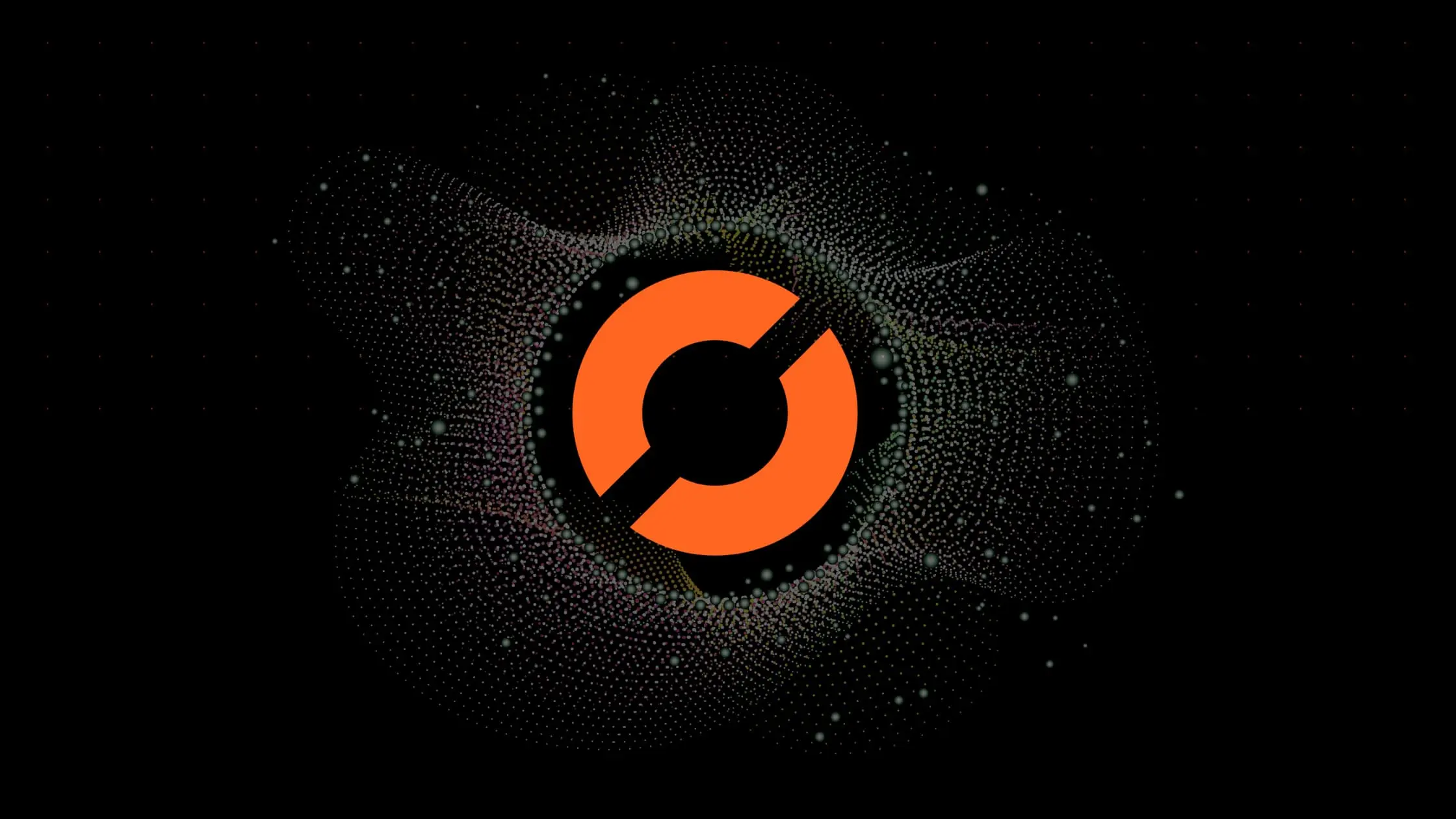
As a data scientist or software engineer, you may have encountered situations where the default threshold for classification in the LogisticRegression
algorithm provided by the popular Python library scikit-learn
(sklearn) doesn’t fit your specific use case. In this blog post, we will explore what the threshold is, why it matters, and how to change it to improve the performance of your machine learning models.
Table of Contents
- What is a Threshold in Logistic Regression?
- Why Change the Threshold?
- How to Change the Threshold in Logistic Regression
- Common Errors and How to Handle Them
- Conclusion
What is a Threshold in Logistic Regression?
In binary classification problems, the goal is to predict whether a given sample belongs to one of two classes. The LogisticRegression
algorithm in sklearn works by calculating the probability of a sample belonging to the positive class, and then comparing it to a threshold to make the final prediction.
By default, the threshold value in sklearn is set to 0.5, which means that a sample is classified as positive if its predicted probability is greater than or equal to 0.5, and negative otherwise. However, this threshold may not be appropriate for all use cases. For example, in a medical diagnosis scenario, a false negative (classifying a sick patient as healthy) may be more severe than a false positive (classifying a healthy patient as sick). In this case, we may want to decrease the threshold to increase the sensitivity of the model.
Why Change the Threshold?
Changing the threshold can impact the performance of your machine learning model in several ways. By increasing the threshold, you can improve the specificity of the model (the ability to correctly classify negative samples), while decreasing the sensitivity (the ability to correctly classify positive samples). Conversely, decreasing the threshold can improve the sensitivity of the model, while decreasing the specificity.
In practice, the choice of threshold depends on the specific context of the problem. For example, in a spam email detection system, we may want to prioritize specificity (avoiding false positives) over sensitivity (avoiding false negatives), while in a fraud detection system, we may prioritize sensitivity (avoiding false negatives) over specificity (avoiding false positives).
How to Change the Threshold in Logistic Regression
Fortunately, changing the threshold in sklearn is relatively easy, you can either use predict_proba
or predict
and threshold
.
Method 1: predict_proba
and Manual Thresholding
You can use the predict_proba
method, which returns the probability estimates for each class. By manually setting a threshold, you can control the classification outcome.
# Example code for Method 1
probs = model.predict_proba(X_test)
custom_threshold = 0.3 # Set your desired threshold
predictions = (probs[:, 1] >= custom_threshold).astype(int)
The predict_proba
method of the LogisticRegression
class returns the probability estimates for each sample, which can be used to calculate the predicted class based on a custom threshold value.
Here is the code snippet that demonstrates how to change the threshold value:
from sklearn.linear_model import LogisticRegression
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
# Load the iris dataset
iris = load_iris()
# Split the data into training and test sets
X_train, X_test, y_train, y_test = train_test_split(iris.data, iris.target, test_size=0.3, random_state=42)
# Train a logistic regression model
lr = LogisticRegression()
lr.fit(X_train, y_train)
# Calculate the predicted probabilities for the test set
probs = lr.predict_proba(X_test)
# Set a custom threshold value
custom_threshold = 0.3
# Generate the predicted classes based on the custom threshold
preds = (probs[:,1] >= custom_threshold).astype(int)
# Evaluate the performance of the model using the custom threshold
accuracy = (preds == y_test).mean()
print(f"Accuracy with custom threshold: {accuracy:.2f}")
In this example, we first load the iris dataset and split it into training and test sets. Then we train a logistic regression model using the default threshold value of 0.5. Next, we use the predict_proba
method to calculate the probability estimates for the test set. We then set a custom threshold value of 0.3 and generate the predicted classes based on this threshold. Finally, we evaluate the performance of the model using the custom threshold by calculating the accuracy of the predictions.
Method 2: predict
and threshold
Parameter
In scikit-learn version 0.24 and later, the predict
method for Logistic Regression has an optional threshold
parameter, allowing you to directly set the decision threshold for classification. This parameter enables you to control the balance between precision and recall based on your specific use case.
Example Code:
from sklearn.linear_model import LogisticRegression
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.datasets import make_classification
# Load the iris dataset
iris = load_iris()
# Split the data into training and test sets
X_train, X_test, y_train, y_test = train_test_split(iris.data, iris.target, test_size=0.3, random_state=42)
# Create a Logistic Regression model
model = LogisticRegression()
# Fit the model on the training data
model.fit(X_train, y_train)
# Set your desired threshold
custom_threshold = 0.3
# Use the predict method with the threshold parameter
predictions = (model.predict(X_test, threshold=custom_threshold)).astype(int)
In this example, the predict
method is used with the threshold
parameter set to custom_threshold
, which is a value between 0 and 1. The predict
method then applies this threshold to the predicted probabilities and classifies instances accordingly.
This method provides a straightforward way to adjust the decision threshold without the need for manual thresholding based on the predict_proba
output. It simplifies the process of customizing the classification threshold directly within the model’s prediction step.
Common Errors and How to Handle Them
Error 1: Threshold Out of Range
Error Message: “ValueError: threshold should be in [0, 1]”
Solution: Ensure that your custom threshold is within the valid range [0, 1].
Error 2: Invalid Input to threshold
Parameter
Error Message: “TypeError: predict() got an unexpected keyword argument ‘threshold’”
Solution: Make sure you are using scikit-learn version 0.24 or later, as the threshold
parameter was introduced in this version.
Conclusion
Customizing the threshold for Logistic Regression in scikit-learn can be beneficial for improving model performance in certain situations. By understanding the methods for changing the threshold, handling common errors, and exploring examples, you can effectively leverage these techniques in your classification tasks. Adjusting the threshold allows you to strike the right balance between precision and recall, tailoring the model to meet the specific needs of your application.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.