How to Calculate Percentage with Pandas DataFrame
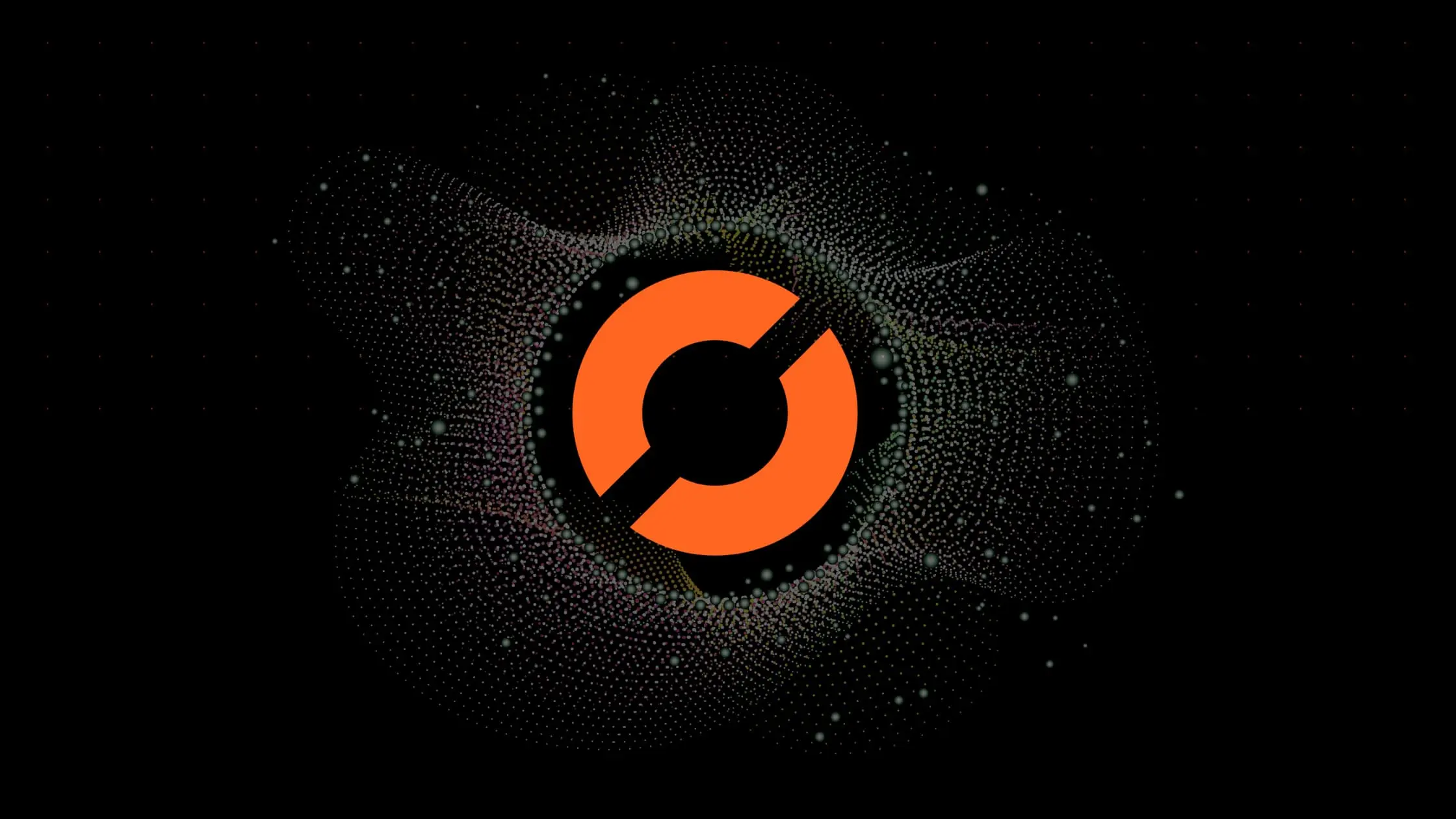
What is Pandas' DataFrame?
Pandas is a popular open-source library for data manipulation and analysis in Python. It provides data structures for efficiently storing and manipulating large datasets. Pandas' DataFrame is a two-dimensional table-like data structure, where each column can have a different data type. It is similar to a spreadsheet or SQL table, where each row represents a record or observation, and each column represents a feature or variable.
How to Calculate Percentage with Pandas' DataFrame
Calculating percentage with Pandas' DataFrame is a straightforward process. We can use the following steps:
- Calculate the numerator
- Calculate the denominator
- Divide the numerator by the denominator
- Multiply the result by 100 to get the percentage
Example 1: Calculating Percentage of a Single Column
Suppose we have a Pandas' DataFrame df
with a single column A
representing the number of apples sold in a store. We want to calculate the percentage of apples sold per day. We can use the following code:
import pandas as pd
df = pd.DataFrame({'A': [10, 20, 30, 40, 50]})
total = df['A'].sum()
percentage = (df['A'] / total) * 100
print(percentage)
Output:
0 6.666667
1 13.333333
2 20.000000
3 26.666667
4 33.333333
Name: A, dtype: float64
In this example, we first calculate the total number of apples sold using the sum()
method of Pandas' DataFrame. We then divide each value in column A
by the total and multiply by 100 to get the percentage. The result is a Pandas' Series with the percentage for each row.
Example 2: Calculating Percentage of Multiple Columns
Suppose we have a Pandas' DataFrame df
with multiple columns A
, B
, and C
representing the number of apples, bananas, and cherries sold in a store. We want to calculate the percentage of each fruit sold per day. We can use the following code:
import pandas as pd
df = pd.DataFrame({'A': [10, 20, 30, 40, 50], 'B': [5, 15, 25, 35, 45], 'C': [15, 25, 35, 45, 55]})
total = df.sum()
percentage = (df / total) * 100
print(percentage)
Output:
A B C
0 6.666667 4.0 8.571429
1 13.333333 12.0 14.285714
2 20.000000 20.0 20.000000
3 26.666667 28.0 25.714286
4 33.333333 36.0 31.428571
In this example, we first calculate the total number of each fruit sold using the sum()
method of Pandas' DataFrame. We then divide each value in each column by the total and multiply by 100 to get the percentage. The result is a Pandas' DataFrame with the percentage for each row and column.
Common Errors
While calculating percentages with Pandas DataFrame is simple, there are some common errors that data scientists and software engineers may encounter. Here are some of the most frequent ones:
- Using incorrect denominator:
- Dividing by zero: This can occur when the denominator (total or reference value) is zero. Always ensure the denominator is positive and non-zero before calculating the percentage.
- Dividing by a wrong column: Double-check that you are dividing by the intended column or total value when calculating the percentage.
- Data type mismatch:
- Incompatible data types: Ensure the data types of the numerator and denominator are compatible with division. In some cases, you might need to convert data types before calculating the percentage.
- NaN values: NaN (Not a Number) values can lead to errors or unexpected results. Consider handling NaN values appropriately, such as filling them with appropriate values or excluding them from the calculation.
Calculating percentage in place: Modifying original data: While convenient, calculating percentages directly in the original DataFrame can unintentionally modify the data. Consider creating a new column or variable to store the calculated percentages.
Precision and rounding: Loss of information: By default, Pandas displays limited decimal places. This can lead to loss of information when dealing with small percentages. Consider specifying the desired number of decimal places for accurate representation.
Conclusion
Calculating percentage with Pandas' DataFrame is a simple and powerful tool for data analysis. We can easily calculate the percentage of a single column or multiple columns using a few lines of code. By understanding how to calculate percentages with Pandas' DataFrame, we can gain insights into our data and make informed decisions.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.