How to calculate Pandas Correlation of One Column against All Others
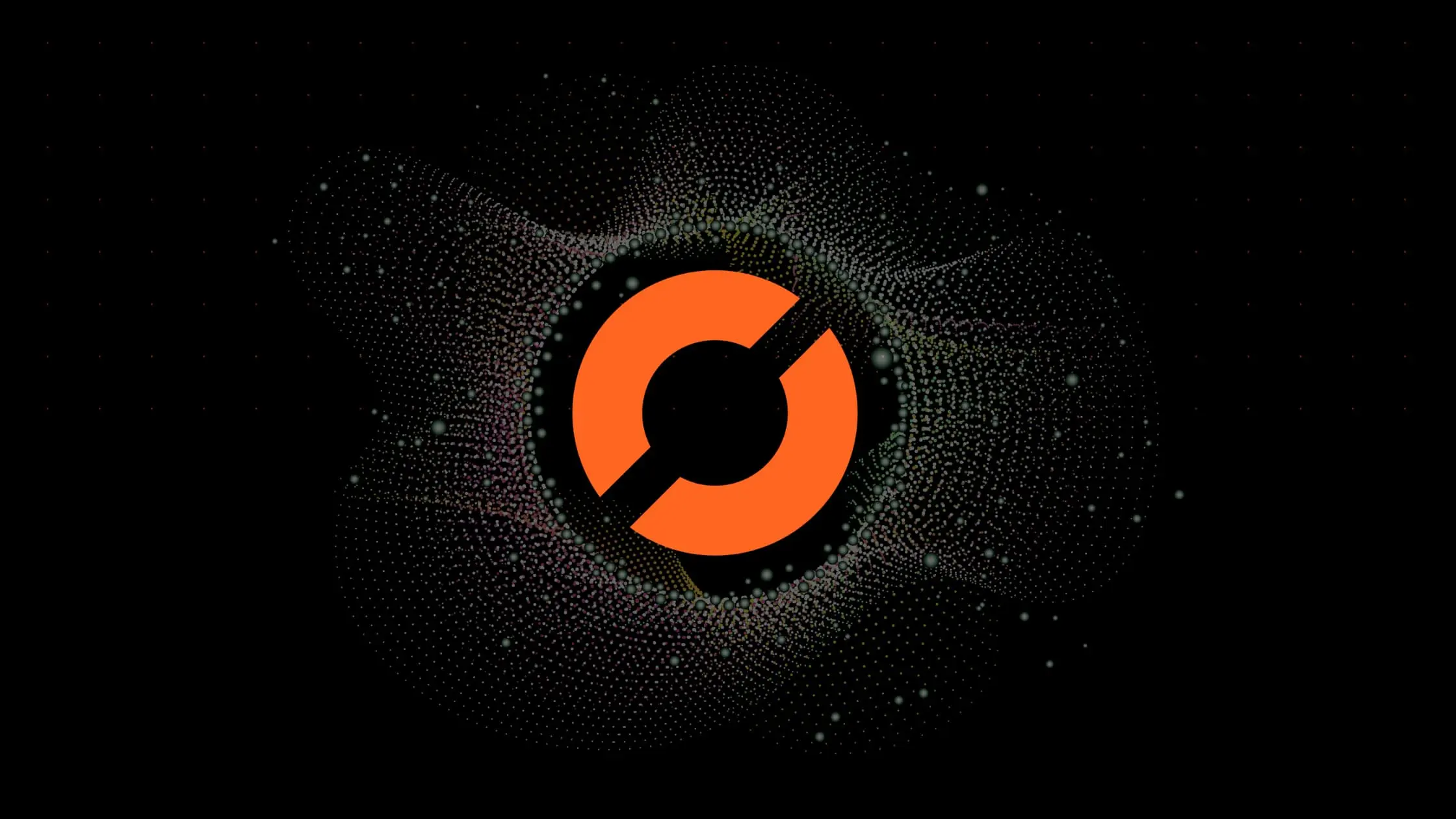
How to calculate Pandas Correlation of One Column against All Others
As a data scientist or software engineer, you are often tasked with analyzing large datasets to gain insights into the underlying trends and patterns. One of the most common techniques used in data analysis is correlation analysis. Correlation analysis is a statistical technique that measures the strength of the relationship between two variables. In this blog post, I will explain how to calculate the Pandas correlation of one column against all others.
What is Pandas?
Pandas is an open-source data analysis and manipulation library for Python. It is built on top of the NumPy library and provides easy-to-use data structures and data analysis tools for Python. Pandas is widely used in the data science community for data cleaning, data exploration, and data analysis.
What is Correlation?
Correlation is a statistical technique that measures the strength of the relationship between two variables. The correlation coefficient, denoted by “r”, ranges from -1 to +1. A value of -1 indicates a perfect negative correlation, a value of 0 indicates no correlation, and a value of +1 indicates a perfect positive correlation.
Calculating Correlation in Pandas
To calculate the correlation of one column against all others in Pandas, we can use the corr()
function. The corr()
function calculates the correlation between columns in a Pandas DataFrame. By default, it calculates the Pearson correlation coefficient, which is the most commonly used correlation coefficient.
Let’s start by importing the Pandas library and creating a sample DataFrame.
import pandas as pd
import numpy as np
# Creating a sample DataFrame with random values
np.random.seed(42) # Setting a seed for reproducibility
df = pd.DataFrame({'A': np.random.rand(5),
'B': np.random.rand(5),
'C': np.random.rand(5)})
We have created a DataFrame with three columns - A, B, and C. Now let’s calculate the correlation of column A against all others using the corr()
function.
# Calculating the correlation of column A against all others
corr_matrix = df.corr()['A']
The corr()
function returns a correlation matrix, which is a square matrix that shows the correlation between all pairs of columns in a DataFrame. In our case, we are interested in the correlation of column A against all others. Therefore, we extract the first column of the correlation matrix by using the ['A']
syntax.
The output of the above code is a Pandas Series that shows the correlation of column A against all others.
A 1.000000
B -0.288389
C 0.849226
Name: A, dtype: float64
We can see that column A has a perfect positive correlation with itself, as expected. Column A also has a very strong positive correlation with columns B and C.
Pros:
Ease of Use: Pandas provides a simple and intuitive interface for data analysis, making it easy for data scientists and software engineers to perform correlation analysis without extensive coding.
Integration with NumPy: Being built on top of the NumPy library, Pandas leverages the efficient numerical operations of NumPy, enhancing performance and computation speed.
Versatility:** The
corr()
function in Pandas is versatile, allowing users to calculate various correlation coefficients, including the widely used Pearson correlation coefficient by default. This flexibility accommodates different analytical needs.Visualization Support: Pandas seamlessly integrates with visualization libraries such as Matplotlib and Seaborn, enabling users to visualize correlation matrices and patterns easily.
Community and Documentation: Pandas has a large and active community, resulting in extensive documentation and a wealth of online resources. This support makes it easier for users to find solutions to common issues and challenges.
Cons:
Memory Usage: For very large datasets, Pandas may consume a significant amount of memory, potentially leading to performance issues on machines with limited resources.
Limited Scalability: While Pandas is suitable for small to medium-sized datasets, it may face scalability challenges when handling extremely large datasets. This can impact the speed and efficiency of correlation calculations.
Single Machine Limitations: Pandas operates on a single machine, which means it may not be the ideal choice for distributed computing and parallel processing. This limitation can be a constraint when dealing with massive datasets that require distributed computing.
Learning Curve: Despite its user-friendly interface, mastering all of Pandas' capabilities, including advanced features for correlation analysis, may have a learning curve for beginners.
Error Handling:
Data Type Compatibility: Ensure that the data types of the columns being correlated are appropriate for correlation analysis. Non-numeric data or missing values can result in errors or inaccurate correlation calculations.
DataFrame Structure: Verify that the DataFrame structure is consistent, with the same set of columns across the dataset. A mismatch in column names or missing columns can lead to unexpected errors.
Outliers: Be cautious of outliers in the data, as extreme values can disproportionately influence correlation coefficients. Consider handling outliers appropriately, such as through data preprocessing or using robust correlation measures.
Contextual Understanding: Always interpret correlation results in the context of the specific data and problem domain. Correlation does not imply causation, and misinterpretation of results can lead to erroneous conclusions.
Library Dependencies: Confirm that the required libraries, including Pandas and NumPy, are correctly installed and up to date. Incompatibility between library versions could lead to function failures or unexpected behavior.
Conclusion
In this blog post, we have learned how to calculate the Pandas correlation of one column against all others. Correlation analysis is a powerful technique that can be used to gain insights into the underlying relationships between variables in a dataset. Pandas provides easy-to-use tools for calculating correlations, making it a popular choice for data scientists and software engineers.
Remember to always carefully consider the context and assumptions of your data when interpreting correlation results. Correlation does not imply causation, and other factors may be at play in the relationship between variables.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.