How to Calculate Error for a Neural Network
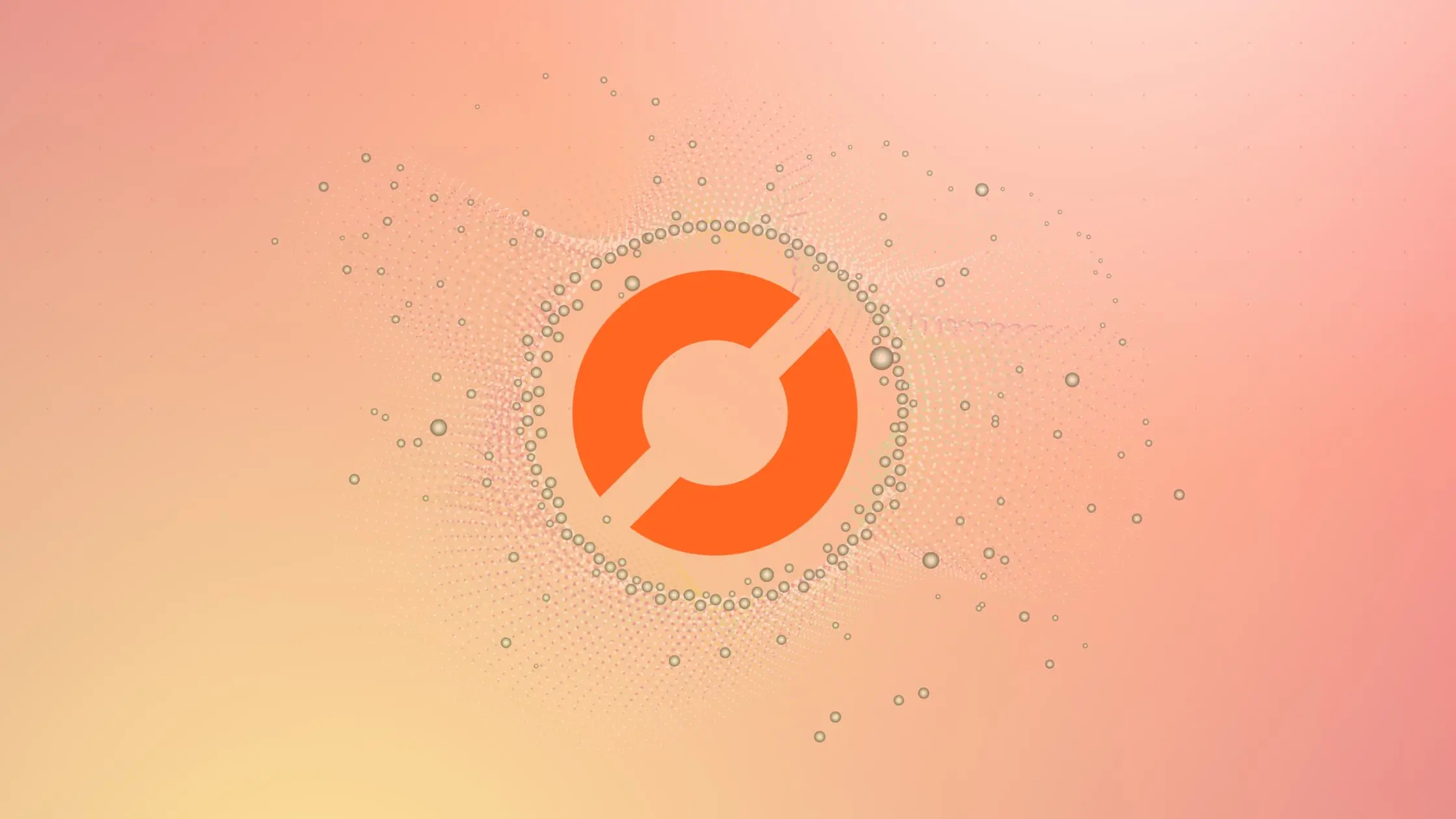
As a data scientist or software engineer, building and training neural networks is a crucial part of your job. However, after training a neural network, it is important to assess its accuracy and performance. This is where calculating error comes into play. In this article, we will discuss different types of errors in neural networks and how to calculate them.
Table of Contents
- Types of Errors in Neural Networks
- Calculating Error in Neural Networks
- Common Errors and Solutions
- Conclusion
Types of Errors in Neural Networks
Before diving into the calculation of errors, let’s first understand the different types of errors that can occur in a neural network. The three most common types of errors in neural networks are:
Training Error: This is the difference between the predicted output and the actual output during the training phase. The goal of training is to minimize this error by adjusting the weights and biases of the network.
Validation Error: This is the difference between the predicted output and the actual output on a validation set. The validation set is a separate set of data that is not used during training. The goal of validation is to prevent overfitting and ensure that the network is generalizing well.
Test Error: This is the difference between the predicted output and the actual output on a test set. The test set is a completely independent set of data that is not used during training or validation. The goal of testing is to evaluate the performance of the network on unseen data.
Calculating Error in Neural Networks
Now that we understand the different types of errors, let’s discuss how to calculate them.
Training Error
The training error is calculated during the training phase. It is the difference between the predicted output and the actual output for each training example. The most common way to calculate the training error is by using a loss function.
A loss function measures how well the network is predicting the output for a given input. There are many different types of loss functions. A loss function, such as Mean Squared Error (MSE), is commonly used.
# Example for Mean Squared Error
mse = ((predictions - targets) ** 2).mean()
The goal of training is to minimize the loss by adjusting the weights and biases of the network. This is done through a process called backpropagation.
Validation Error
The validation error is calculated on a separate validation set. It is the difference between the predicted output and the actual output for each validation example. The goal of validation is to prevent overfitting.
To calculate the validation error, we use the same loss function as the training error. However, we do not adjust the weights and biases of the network during validation.
Test Error
The test error is calculated on a completely independent test set. It is the difference between the predicted output and the actual output for each test example. The goal of testing is to evaluate the performance of the network on unseen data.
To calculate the test error, we use the same loss function as the training and validation error. However, we do not adjust the weights and biases of the network during testing.
Loss Functions
Mean Squared Error (MSE):
def mean_squared_error(predictions, targets):
return ((predictions - targets) ** 2).mean()
# Example usage:
predictions = model.predict(X)
mse = mean_squared_error(predictions, y_true)
print(f"Mean Squared Error: {mse}")
Cross-Entropy Loss:
import numpy as np
def cross_entropy_loss(predictions, targets):
epsilon = 1e-15
predictions = np.clip(predictions, epsilon, 1 - epsilon)
return -np.mean(targets * np.log(predictions) + (1 - targets) * np.log(1 - predictions))
# Example usage:
predictions = model.predict(X)
ce_loss = cross_entropy_loss(predictions, y_true)
print(f"Cross-Entropy Loss: {ce_loss}")
Mean Absolute Error (MAE):
Mean Absolute Error is a metric that calculates the average absolute differences between the predicted and true values.
Copy code
def mean_absolute_error(predictions, targets):
return np.abs(predictions - targets).mean()
# Example usage:
predictions = model.predict(X)
mae = mean_absolute_error(predictions, y_true)
print(f"Mean Absolute Error: {mae}")
Huber Loss:
Huber Loss combines the best of both Mean Squared Error and Mean Absolute Error. It is less sensitive to outliers than Mean Squared Error and provides a compromise between Mean Squared Error and Mean Absolute Error.
def huber_loss(predictions, targets, delta=1.0):
errors = predictions - targets
huber_condition = np.abs(errors) < delta
squared_loss = 0.5 * (errors ** 2)
linear_loss = delta * (np.abs(errors) - 0.5 * delta)
return np.where(huber_condition, squared_loss, linear_loss).mean()
# Example usage:
predictions = model.predict(X)
huber_loss_value = huber_loss(predictions, y_true)
print(f"Huber Loss: {huber_loss_value}")
Hinge Loss (for Classification):
Hinge Loss is commonly used for support vector machine (SVM) models but can also be applied to neural networks for binary classification tasks.
def hinge_loss(predictions, targets):
return np.maximum(0, 1 - predictions * targets).mean()
# Example usage:
predictions = model.predict(X)
# Assuming targets are -1 for one class and 1 for the other
hinge_loss_value = hinge_loss(predictions, y_true)
print(f"Hinge Loss: {hinge_loss_value}")
Common Errors and Solutions:
Vanishing Gradient:
The vanishing gradient problem occurs when the gradients become extremely small during backpropagation, hindering the training process. Using activation functions like ReLU can mitigate this issue.
from keras.layers import Dense, Activation
model.add(Dense(units=64, input_dim=100))
model.add(Activation('relu'))
Exploding Gradient:
Conversely, exploding gradients happen when gradients become too large, leading to instability during training. Gradient clipping is a common technique to address this issue.
from keras.optimizers import SGD
sgd = SGD(clipvalue=0.5)
model.compile(optimizer=sgd, loss='mse')
Overfitting:
Overfitting occurs when a neural network learns the training data too well, capturing noise or irrelevant patterns. As a result, the model performs poorly on new, unseen data.
Solution:
Use techniques such as dropout and regularization to prevent overfitting.
- Dropout:
from keras.layers import Dropout
model.add(Dense(units=64, input_dim=100, activation='relu'))
model.add(Dropout(0.5))
model.add(Dense(units=1, activation='sigmoid'))
- Regularization:
from keras.regularizers import l2
model.add(Dense(units=64, input_dim=100, activation='relu', kernel_regularizer=l2(0.01)))
model.add(Dense(units=1, activation='sigmoid'))
Underfitting:
Underfitting occurs when a model is too simple to capture the underlying patterns in the data, resulting in poor performance on both training and test sets.
Solution:
Increase model complexity and adjust hyperparameters to address underfitting.
Copy code
model.add(Dense(units=128, input_dim=100, activation='relu'))
model.add(Dense(units=64, activation='relu'))
model.add(Dense(units=1, activation='sigmoid'))
Learning Rate Issues:
Choosing an inappropriate learning rate can lead to slow convergence or instability during training.
Solution:
Experiment with different learning rates or use adaptive optimization algorithms like Adam.
from keras.optimizers import Adam
model.compile(optimizer=Adam(lr=0.001), loss='mse')
Conclusion
Calculating error for a neural network is an important step in assessing its accuracy and performance. There are three types of errors that can occur in a neural network: training error, validation error, and test error. The most common way to calculate these errors is by using a loss function, such as the mean squared error function.
As a data scientist or software engineer, it is important to understand how to calculate these errors and how to interpret them. By doing so, you can improve the accuracy and performance of your neural network and ensure that it is generalizing well to unseen data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.