How to Append an Empty Row in a DataFrame Using Pandas
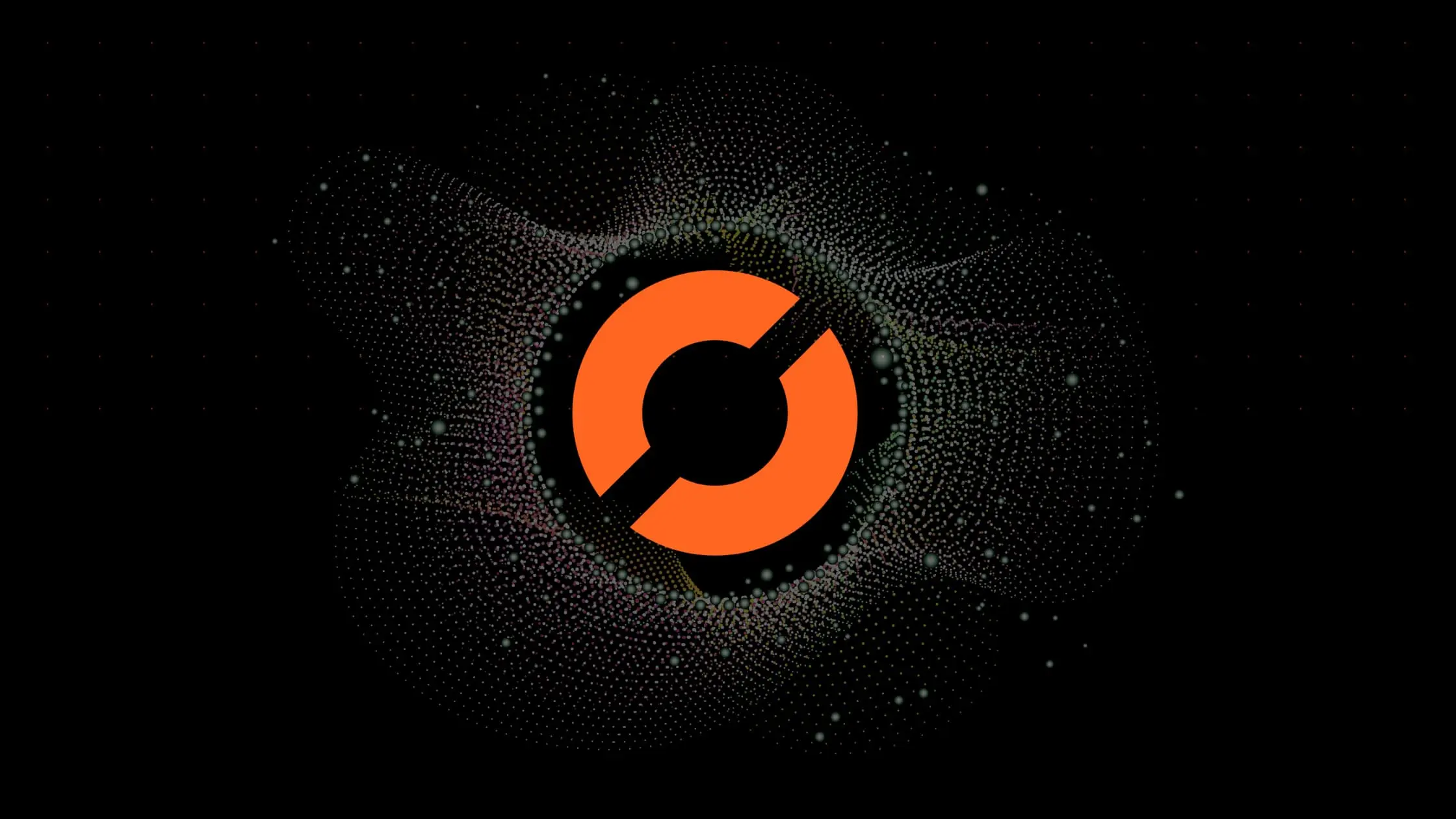
Data analysis is an essential part of any software engineering or data science project. One of the most commonly used libraries for data analysis in Python is Pandas. Pandas provides a variety of methods to manipulate and analyze data, and in this article, we will discuss one of them: appending an empty row to a DataFrame.
Table of Contents
What is a DataFrame?
A DataFrame is a two-dimensional labeled data structure that is used to store and manipulate data in Python. It is a primary data structure in Pandas that is similar to a spreadsheet or a SQL table. A DataFrame consists of rows and columns, where each row represents an observation or a record, and each column represents a variable or a feature.
Why Append an Empty Row to a DataFrame?
Appending an empty row to a DataFrame is useful in several scenarios. For instance, it can be used to add a new record to the DataFrame, which is useful when you are collecting data in real-time. It can also be used to initialize a DataFrame with a specific shape or to create a DataFrame with a header and no data.
How to Append an Empty Row to a DataFrame Using Pandas
To append an empty row to a DataFrame using Pandas, we can use the .loc
accessor and the square bracket notation. Here is an example:
import pandas as pd
# create a DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
# append an empty row
df.loc[len(df)] = pd.Series(dtype='float64')
Output:
A B
0 1.0 4.0
1 2.0 5.0
2 3.0 6.0
3 NaN NaN
In this example, we first create a DataFrame df
with two columns A
and B
and three rows. Then, we use the .loc
accessor to access the last row of the DataFrame and assign it an empty pd.Series
object. The pd.Series
object is initialized with the dtype
parameter set to float64
, which specifies the data type of the Series.
We can also assign values to the columns of the empty row by specifying the column names as keys of the pd.Series
object. For example, to assign a value of 0
to the A
column of the empty row, we can use the following code:
df.loc[len(df), 'A'] = 0
Output:
A B
0 1.0 4.0
1 2.0 5.0
2 3.0 6.0
3 0.0 NaN
In this code, we use the .loc
accessor to access the last row of the DataFrame and the A
column of that row, and assign it a value of 0
.
Append Multiple Empty Rows to a DataFrame Using a Loop
Appending a single empty row to a DataFrame is useful, but there are situations where you may need to append multiple empty rows. This can be achieved efficiently using a loop. Below is an extension to the article demonstrating how to append multiple empty rows to a DataFrame:
import pandas as pd
# Function to append n empty rows to a DataFrame
def append_empty_rows(dataframe, n):
for _ in range(n):
dataframe.loc[len(dataframe)] = pd.Series(dtype='float64')
# Create a DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
# Append three empty rows
append_empty_rows(df, 3)
# Display the updated DataFrame
print(df)
Output:
A B
0 1.0 4.0
1 2.0 5.0
2 3.0 6.0
3 NaN NaN
4 NaN NaN
5 NaN NaN
In this example, the append_empty_rows
function takes a DataFrame and the number of empty rows to append (n
) as parameters. It uses a loop to append the specified number of empty rows to the DataFrame using the same approach as in the original article.
This method is particularly helpful when you need to initialize a DataFrame with a certain number of empty rows or when you want to add a specific number of blank records to accommodate future data.
Conclusion
In this article, we learned how to append an empty row to a DataFrame using Pandas. We discussed what a DataFrame is and why appending an empty row is useful. We also provided an example of how to append an empty row to a DataFrame and how to assign values to the columns of the empty row. By following the steps outlined in this article, you can easily append an empty row to a DataFrame using Pandas and manipulate your data as needed.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.