How to Append a Row to Pandas DataFrame using pandasconcat
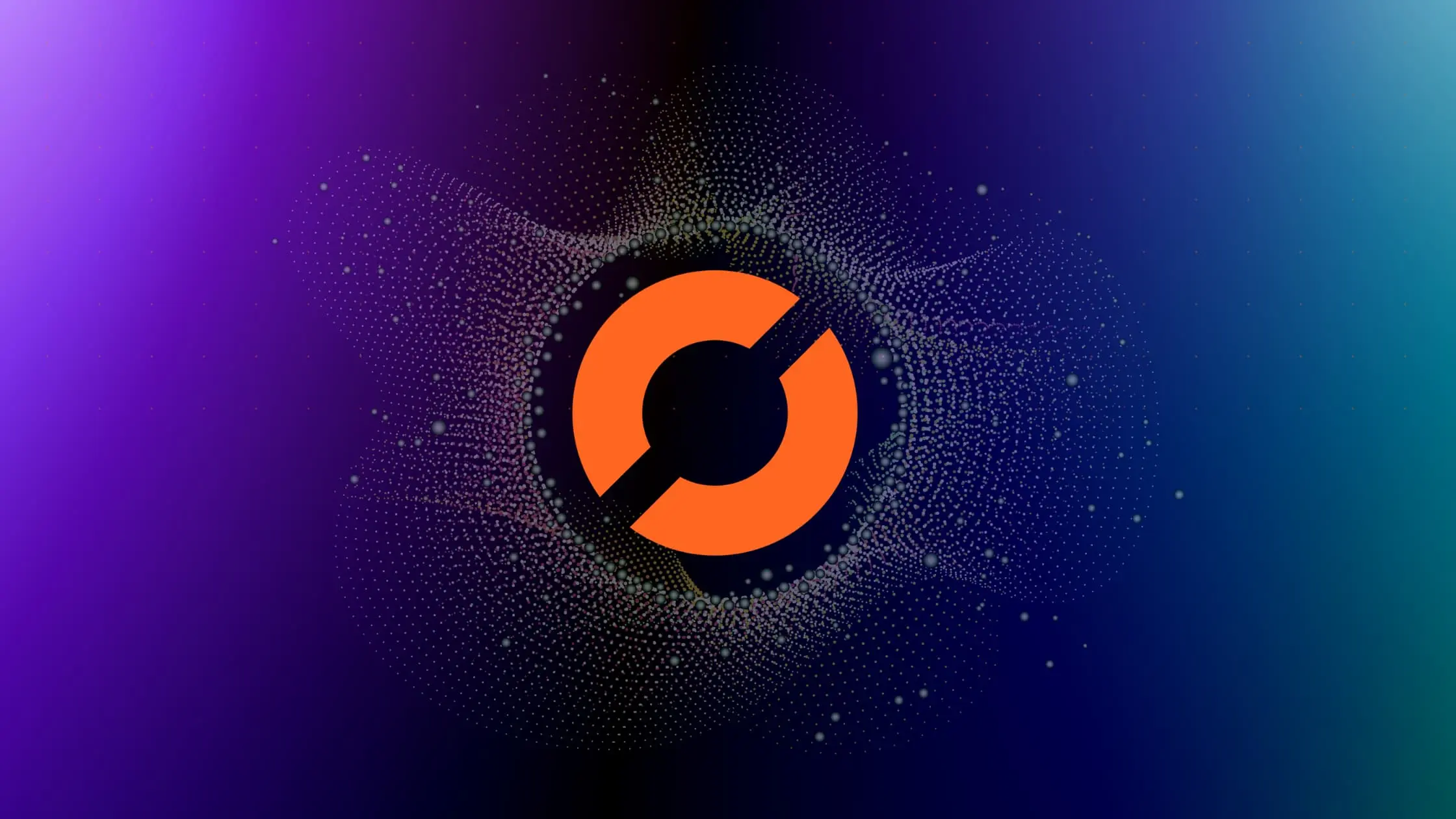
As a data scientist or software engineer, you are likely to come across a situation where you need to append a new row to an existing Pandas DataFrame. In such cases, the pandas.concat()
method can be used to concatenate two or more DataFrames along a particular axis. In this article, we will explore how to use pandas.concat()
to append a new row to an existing DataFrame.
Table of Contents
What is Pandas?
Pandas is a popular Python library used for data manipulation and analysis. It provides data structures for efficiently storing and manipulating large datasets, and a wide range of tools for cleaning, transforming, and analyzing data.
One of the most commonly used data structures in Pandas is the DataFrame. A DataFrame is a two-dimensional table-like data structure that organizes data into rows and columns. Each column in a DataFrame represents a variable, and each row represents a record or observation.
Appending a Row to a Pandas DataFrame
Using pandas.concat
Appending a row to an existing Pandas DataFrame can be achieved using the pandas.concat()
method. The pandas.concat()
method concatenates two or more DataFrames along a particular axis. By default, the method concatenates DataFrames along the vertical axis (axis=0), which means that the rows of the DataFrames are stacked on top of each other.
To append a new row to an existing DataFrame, we can create a new DataFrame with a single row containing the data we want to add, and then concatenate the new DataFrame with the original DataFrame using the pandas.concat()
method.
Here’s an example of how to append a new row to a Pandas DataFrame using pandas.concat()
:
import pandas as pd
# create the original DataFrame
data = {'name': ['John', 'Doe'], 'age': [35, 40]}
df = pd.DataFrame(data)
# create a new row to append to the DataFrame
new_row = pd.DataFrame({'name': ['Jane'], 'age': [28]})
# append the new row to the original DataFrame
df = pd.concat([df, new_row], ignore_index=True)
print(df)
Output:
name age
0 John 35
1 Doe 40
2 Jane 28
In this example, we first create the original DataFrame df
with two rows and two columns. Next, we create a new DataFrame new_row
with a single row containing the data we want to add to the original DataFrame. Finally, we use the pandas.concat()
method to concatenate the two DataFrames along the vertical axis, and assign the result back to the original DataFrame df
.
The ignore_index=True
parameter is used to reset the index of the resulting DataFrame. If this parameter is not set to True, the resulting DataFrame will have the index values of the original DataFrame and the new row.
Pros
Versatility:
concat
is versatile and can concatenate DataFrames along both rows and columns. It can be used for more complex concatenation scenarios.Multiple Concatenation: It allows concatenating multiple DataFrames in a single call.
Fine-grained Control: Offers options like
ignore_index
to reset the index and axis to specify the axis along which concatenation should happen.
Cons
Syntax Complexity: The syntax might be more complex than other methods for simple row appending tasks.
Additional Configuration: May require additional parameters and settings for specific use cases.
Using loc
Another method to append a row to a Pandas DataFrame is by using the loc accessor. The loc
accessor is a label-based indexer that allows you to modify a DataFrame by accessing a group of rows and columns by labels or a boolean array. Here’s an example of how to use the loc
accessor to append a new row:
import pandas as pd
# create the original DataFrame
data = {'name': ['John', 'Doe'], 'age': [35, 40]}
df = pd.DataFrame(data)
# create a new row to append to the DataFrame
new_row = pd.Series({'name': 'Jane', 'age': 28})
# append the new row using the loc accessor
df.loc[len(df)] = new_row
print(df)
Output:
name age
0 John 35
1 Doe 40
2 Jane 28
In this example, we use the loc
accessor to access the last index of the DataFrame (len(df)
) and assign the values from the new_row
Series to that index. This effectively appends the new row to the original DataFrame.
Pros
Label-Based Indexing: Using
loc
allows label-based indexing, making it easy to append a row with a specific index.Direct Modification: Enables direct modification of the DataFrame by assigning values to a specific location.
Cons
Indexing Knowledge: Requires understanding of DataFrame indexing to use effectively.
Specific Use Case: More suitable for appending a single row or a small number of rows, may not be as convenient for multiple-row additions.
Using append
Another way to append a row to a Pandas DataFrame is by using the append()
method. The append()
method is specifically designed for concatenating rows from another DataFrame or Series. Here’s an example:
import pandas as pd
# create the original DataFrame
data = {'name': ['John', 'Doe'], 'age': [35, 40]}
df = pd.DataFrame(data)
# create a new row to append to the DataFrame
new_row = pd.DataFrame({'name': ['Jane'], 'age': [28]})
# append the new row using the append() method
df = df._append(new_row, ignore_index=True)
print(df)
Output:
name age
0 John 35
1 Doe 40
2 Jane 28
In this example, the append()
method is used to concatenate the new_row
DataFrame to the original DataFrame df
. The ignore_index=True parameter is used to reset the index of the resulting DataFrame.
The append()
method provides a concise way to add rows to a DataFrame, and it’s particularly useful when dealing with smaller datasets or when appending a single row.
Pros
Conciseness:
append
provides a concise and readable way to append rows to a DataFrame.Readability: The code is often more readable and straightforward compared to some other methods.
Cons
Limited Functionality: It may not be as powerful as
concat
for more complex concatenation scenarios.Potentially Less Efficient: In certain scenarios, it may be less efficient for appending multiple rows compared to other methods.
Considerations
Task Complexity: For simple row appending tasks,
df.append()
might be the most straightforward. For more complex concatenation scenarios,pandas.concat()
may be preferred.Performance: Depending on the size of the dataset, the performance of each method can vary. It’s worth considering the efficiency of the chosen method for large datasets.
Code Readability: Choose the method that makes the code most readable and maintainable for your specific use case.
Error Handling
Column Mismatch: When the columns in the DataFrames being combined (concatenated, appended, etc.) do not match. Check and ensure that the columns are in the correct order and have the same names. Print an informative error message if a column mismatch is detected.
Incompatible Data Types: When the data types of columns in the DataFrames being combined are not compatible. Check and ensure that the data types of corresponding columns are compatible. Print an informative error message guiding the user to check and convert data types if needed.
Index Out of Range: When trying to append a row using an index that is out of the DataFrame’s range. Check if the index provided for appending is within the valid range. Print an error message if the index is out of range and suggest using a valid index.
Conclusion
In this article, we have explored how to append a row to an existing Pandas DataFrame using the pandas.concat()
method. Pandas is a powerful library for data manipulation and analysis, and the pandas.concat()
method is just one of the many tools it provides for working with DataFrames. By mastering these tools, you can efficiently clean, transform, and analyze large datasets, and make more informed decisions based on your data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.