How to Add a Worksheet to an Existing Excel File with Pandas
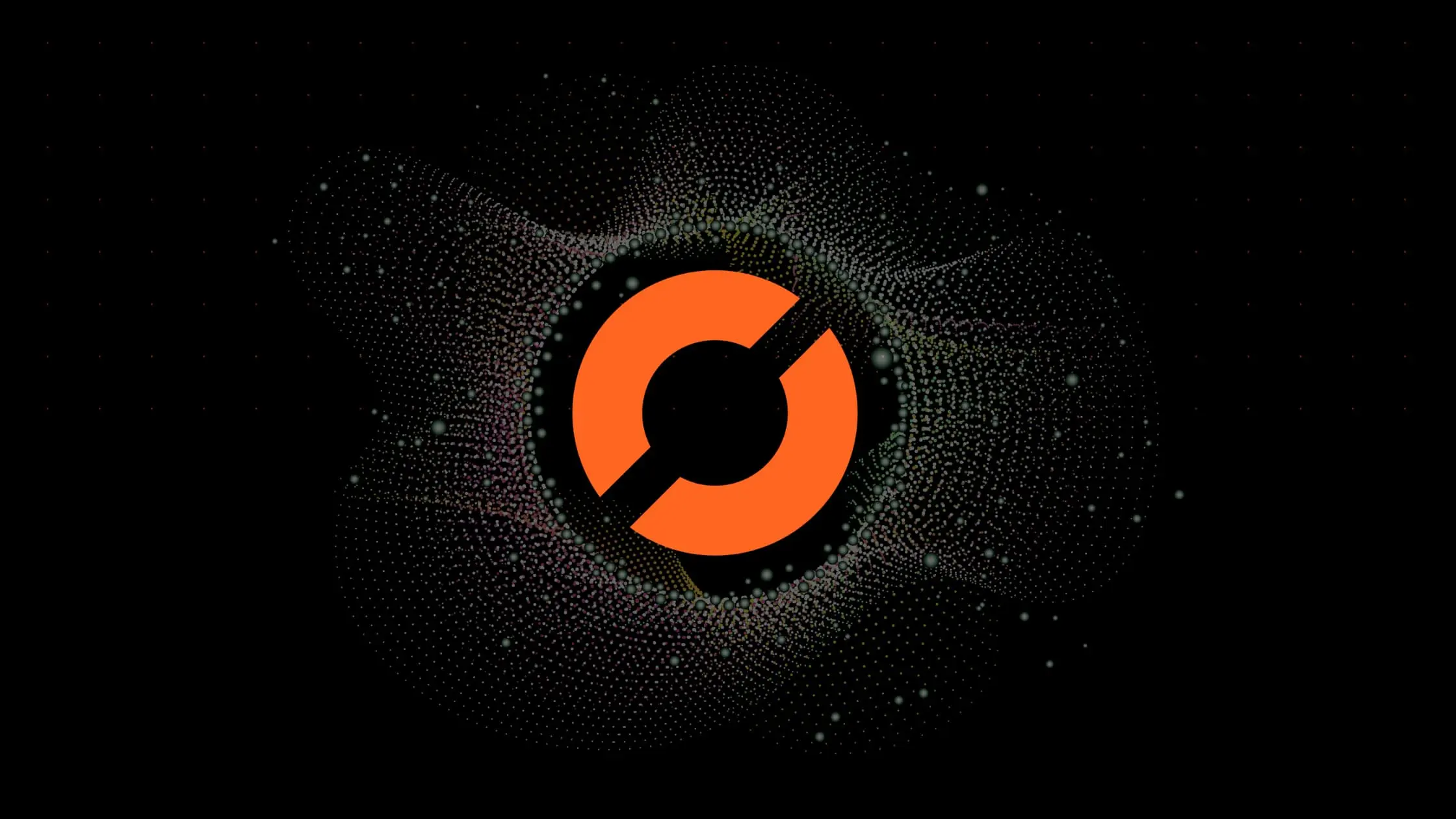
As a data scientist or software engineer, you may often find yourself working with data in various file formats, including Excel files. Excel is a popular choice for storing and analyzing data due to its versatility and ease of use. However, as you work with data, you may need to add new worksheets to an existing Excel file. Fortunately, with the help of Pandas, adding a worksheet to an existing Excel file is a straightforward process.
In this tutorial, we will walk you through the steps to add a worksheet to an existing Excel file using Pandas. We will assume that you have some basic knowledge of Python and Pandas and that you have an existing Excel file that you want to add a worksheet to.
Table of Contents
- Introduction
- Step 1: Import Required Packages
- Step 2: Load the Excel File
- Step 3: Write Data to the New Worksheet
- Error Handling
- Conclusion
Step 1: Import Required Packages
The first step is to import the required packages, which in this case are Pandas and openpyxl. Openpyxl is a Python library for reading and writing Excel files. You can install both packages using pip as follows:
pip install pandas openpyxl
Once you have installed these packages, you can import them in your Python script as follows:
import pandas as pd
Step 2: Load the Excel File
The next step is to load the existing Excel file into a Pandas DataFrame. You can do this using the Pandas read_excel()
function as follows:
df = pd.read_excel('existing_file.xlsx', sheet_name='Sheet1')
The read_excel()
function reads the existing Excel file into a Pandas DataFrame, with the specified sheet name. If you don’t specify the sheet name, Pandas will read the first sheet by default.
Step 3: Write Data to the New Worksheet
Finally, you can write data to the new worksheet using the Pandas DataFrame that you loaded earlier. To write data to the new worksheet, you need to specify the sheet name when you write the DataFrame to Excel using the to_excel()
function.
import pandas as pd
data = {'EmployeeID': [101, 102, 103],
'EmployeeName': ['Alice', 'Bob', 'Charlie'],
'Salary': [60000, 70000, 80000]}
df_new = pd.DataFrame(data)
with pd.ExcelWriter('existing_file.xlsx', engine='openpyxl', mode='a') as writer:
# Write the new DataFrame to a new sheet
df_new.to_excel(writer, sheet_name='New Sheet', index=False)
In this example, the ExcelWriter
is used with the openpyxl
engine in append mode (mode='a'
). This allows you to add a new sheet ('New Sheet'
) to the existing Excel file without deleting the previously existing sheets. The ExcelWriter provides a convenient and efficient way to manage Excel files, especially when dealing with multiple sheets or when you want to preserve existing data.
The to_excel()
function writes the Pandas DataFrame to Excel with the specified sheet name and index. Setting index=False
prevents Pandas from writing the DataFrame index to Excel.
Error Handling
Load the Excel File
# Specify the file path
file_path = 'existing_file.xlsx'
try:
# Load the existing Excel file into a Pandas DataFrame
df = pd.read_excel(file_path, sheet_name='Sheet1')
except FileNotFoundError:
print(f"Error: File '{file_path}' not found. Please provide the correct file path.")
exit()
except pd.errors.EmptyDataError:
print(f"Error: File '{file_path}' is empty. Please make sure it contains data.")
exit()
except pd.errors.SheetNameNotFound as e:
print(f"Error: {e}. Please provide a valid sheet name.")
exit()
This snippet handles potential errors that may occur when loading the existing Excel file. It checks for file not found, empty file, and invalid sheet name.
Write Data to the New Worksheet
# Create a new DataFrame (for illustration purposes)
df_new = pd.DataFrame({'Column1': [1, 2, 3], 'Column2': ['A', 'B', 'C']})
# Write data to the new worksheet
try:
with pd.ExcelWriter(file_path, engine='openpyxl', mode='a') as writer:
# Write the new DataFrame to a new sheet
df_new.to_excel(writer, sheet_name='New Sheet', index=False)
except PermissionError:
print(f"Error: Permission denied. Please make sure you have write access to the file '{file_path}'.")
exit()
except Exception as e:
print(f"An unexpected error occurred: {e}")
exit()
This snippet handles errors that may occur when writing data to the new worksheet. It specifically checks for permission issues and catches any unexpected errors during the execution.
Conclusion
In this tutorial, we have shown you how to add a worksheet to an existing Excel file using Pandas. The process involves loading the existing Excel file into a Pandas DataFrame, creating a new worksheet using openpyxl
, and writing data to the new worksheet using the Pandas to_excel()
function. With these steps, you can easily add new worksheets to existing Excel files, making it easier to organize and analyze your data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.