How to Access MultiIndex DataFrame in Pandas
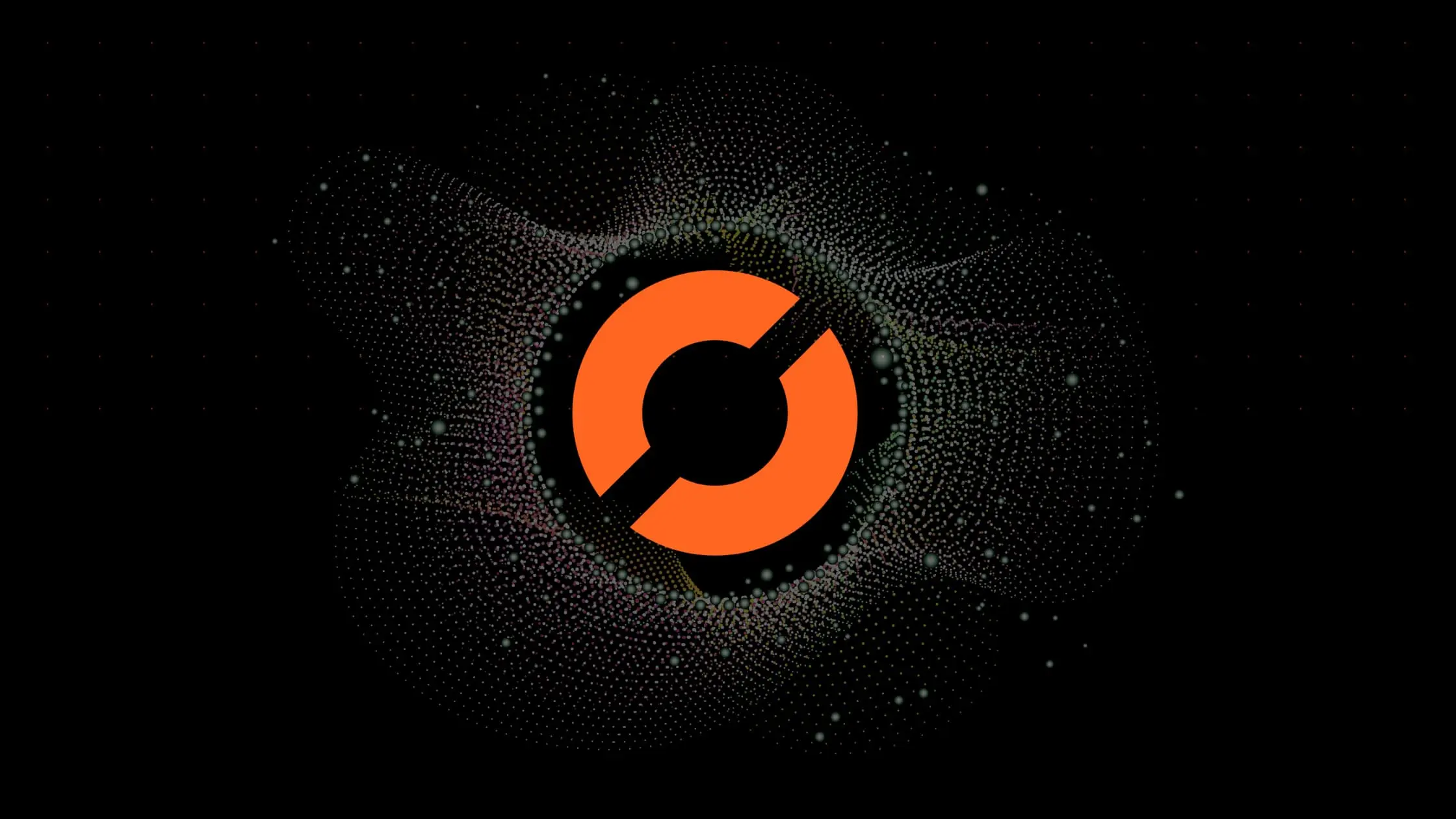
As a data scientist or software engineer, you might come across complex datasets with multiple levels of indexing. Pandas is a powerful library in Python that facilitates data manipulation and analysis. In this article, we will explore how to access a MultiIndex DataFrame in Pandas.
Table of Contents
- Introduction
- What is a MultiIndex DataFrame?
- How to Create a MultiIndex DataFrame
- How to Access MultiIndex DataFrame
- Pros and Cons of MultiIndex DataFrames
- Error Handling
- Conclusion
What is a MultiIndex DataFrame?
A MultiIndex DataFrame is a type of Pandas DataFrame that has multiple levels of indexing. It is a way of representing data in a hierarchical or nested structure. The index can be thought of as a way of labeling the rows and columns of the DataFrame. In a MultiIndex DataFrame, the index is a combination of two or more levels of labels.
For example, consider a dataset that contains the stock prices of different companies on different dates. The DataFrame can be indexed by the company name and the date. This creates a MultiIndex DataFrame with two levels of indexing.
How to Create a MultiIndex DataFrame
Let’s create a sample MultiIndex DataFrame to demonstrate how to access it. We will use the pd.MultiIndex.from_product
method to create a MultiIndex and then pass it to the DataFrame constructor.
import pandas as pd
index = pd.MultiIndex.from_product([['A', 'B'], [1, 2]])
df = pd.DataFrame({'values': [10, 20, 30, 40]}, index=index)
This will create a DataFrame that looks like this:
values
A 1 10
2 20
B 1 30
2 40
As you can see, the DataFrame has two levels of indexing: ['A', 'B']
and [1, 2]
.
How to Access MultiIndex DataFrame
Accessing a MultiIndex DataFrame can be a bit tricky, but Pandas provides several ways to do it.
Accessing Rows
To access a row in a MultiIndex DataFrame, you need to specify the labels for each level of the index. You can do this using the .loc
accessor.
# Accessing row with index ('A', 1)
row = df.loc[('A', 1)]
print(row)
This will output:
values 10
Name: (A, 1), dtype: int64
Accessing Columns
To access a column in a MultiIndex DataFrame, you can use the .loc
accessor and specify the column name.
# Accessing column with name 'values' for company 'A'
column = df.loc['A', 'values']
print(column)
This will output:
1 10
2 20
Name: values, dtype: int64
Accessing Cells
To access a specific cell in a MultiIndex DataFrame, you can use the .loc
accessor and specify the labels for each level of the index and the column name.
# Accessing cell for company 'B' on date 2
cell = df.loc[('B', 2), 'values']
print(cell)
This will output:
40
Pros and cons of MultiIndex DataFrames
Pros
Hierarchical Indexing: MultiIndex allows for the representation of complex, hierarchical data in a structured and intuitive way, making it easier to organize and interpret.
Flexible Data Aggregation: They facilitate advanced data aggregation and grouping operations, allowing users to perform computations at various levels of the hierarchy.
Efficient Data Slicing: MultiIndexing supports efficient querying and data slicing, making it convenient to access subsets of data based on multiple keys.
Enhanced Data Representation: They are ideal for representing high-dimensional data in a two-dimensional table, which is particularly useful in time series and panel data analysis.
Cons
Complexity in Syntax: The syntax for manipulating MultiIndex DataFrames can be complex and unintuitive, especially for beginners or those used to working with single-index DataFrames.
Performance Overhead: MultiIndex DataFrames can have performance overhead, especially when dealing with large datasets and complex indexing operations.
Difficulty in Visualization: Visualizing data from MultiIndex DataFrames can be challenging as most plotting libraries are optimized for single-level indexing.
Data Manipulation Challenges: Simple tasks like sorting, merging, and reshaping can become complicated with multiple indices, requiring a deeper understanding of Pandas operations.
Error Handling
Invalid Index Errors: Always validate the existence of an index before accessing it. Using try-except blocks can prevent crashes due to invalid indices.
Ambiguous Indexing Errors: Be explicit in your indexing to avoid ambiguity. For example, use loc or iloc explicitly instead of direct indexing to clarify row vs. column access.
Handling Missing Data: Be cautious of missing data in MultiIndex DataFrames. Employ methods like
fillna()
ordropna()
to handle NaN values as per the data analysis requirements.Performance Optimization: For large datasets, consider optimizing performance by sorting indices using
sort_index()
or using the at and iat methods for faster access in certain scenarios.Type Errors in Indices: Ensure that the data types of the indices are consistent and appropriate for the operations being performed.
Updating MultiIndex Structure: When modifying the structure of a MultiIndex DataFrame (like adding or removing levels), ensure that the changes are consistent across the DataFrame to avoid alignment issues.
Conclusion
In this article, we explored how to access a MultiIndex DataFrame in Pandas. We created a sample MultiIndex DataFrame and demonstrated how to access rows, columns, and cells. MultiIndex DataFrame is a powerful way to represent complex datasets with multiple levels of indexing. With the techniques discussed in this article, you can easily access and manipulate data in a MultiIndex DataFrame.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.