Getting min and max Dates from a pandas dataframe
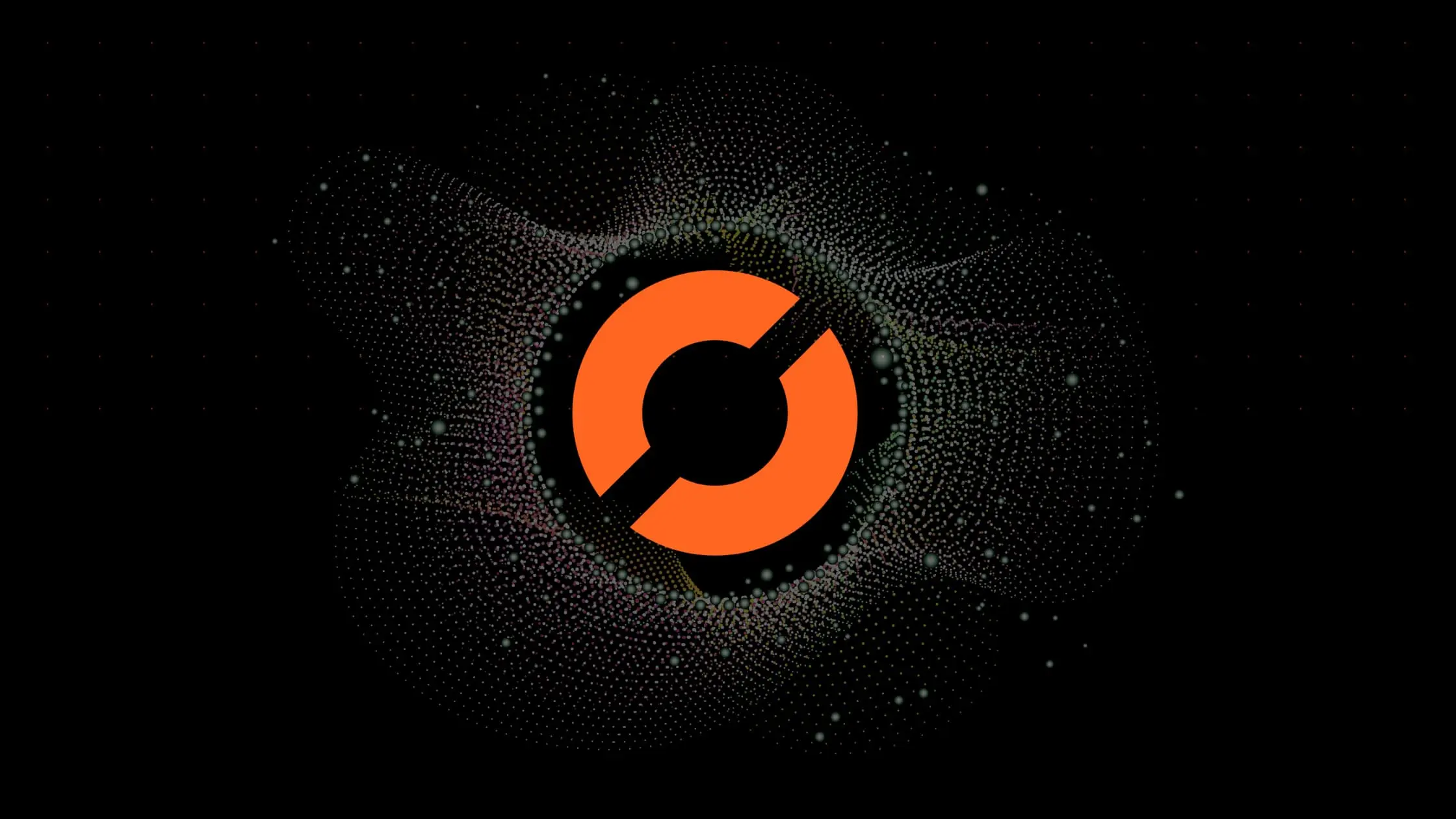
As a data scientist or software engineer, you might often find yourself working with large datasets that contain date and time information. One of the most common tasks in this domain is to find the minimum and maximum dates present in a pandas dataframe. In this article, we will explore how to do this using Python’s pandas library.
Table of Contents
What is pandas?
Pandas is a popular data analysis library for Python. It provides data structures and functions for manipulating and analyzing structured data. One of the key data structures in pandas is the DataFrame. A DataFrame is a two-dimensional table-like data structure where each column can have a different data type. It is similar to a spreadsheet or a SQL table.
Pandas provides a wide range of functions for manipulating and analyzing data in a DataFrame. In this article, we will focus on how to get the min and max dates from a pandas DataFrame.
How to get the min and max dates from a pandas DataFrame?
Method 1: Using min()
and max()
Suppose we have a pandas DataFrame with a column of dates. We want to find the earliest and latest dates in that column. We can do this using the min()
and max()
functions provided by pandas.
import pandas as pd
# Create a sample DataFrame
df = pd.DataFrame({'Dates': ['2022-01-01', '2022-01-02', '2022-01-03', '2022-01-04']})
# Convert the dates column to datetime format
df['Dates'] = pd.to_datetime(df['Dates'])
# Find the minimum and maximum dates
min_date = df['Dates'].min()
max_date = df['Dates'].max()
print('Minimum date:', min_date)
print('Maximum date:', max_date)
In the above code, we first create a sample DataFrame with a column of dates. We then convert the dates column to datetime format using the pd.to_datetime()
function. This is necessary because pandas provides specialized functions for manipulating datetime data. Finally, we use the min()
and max()
functions to find the earliest and latest dates in the Dates column.
Output:
Minimum date: 2022-01-01 00:00:00
Maximum date: 2022-01-04 00:00:00
Method 2: Using nlargest
and nsmallest
functions
Apart from using min()
and max()
functions, pandas also provides the nlargest
and nsmallest
functions, which allow you to retrieve the top N largest or smallest values from a DataFrame. In this case, you can use these functions to get the maximum and minimum dates:
import pandas as pd
# Create a sample DataFrame
df = pd.DataFrame({'Dates': ['2022-01-01', '2022-01-02', '2022-01-03', '2022-01-04']})
# Convert the dates column to datetime format
df['Dates'] = pd.to_datetime(df['Dates'])
# Find the minimum and maximum dates using nlargest and nsmallest
min_date = df.nsmallest(1, 'Dates')['Dates'].iloc[0]
max_date = df.nlargest(1, 'Dates')['Dates'].iloc[0]
print('Minimum date:', min_date)
print('Maximum date:', max_date)
Output:
Minimum date: 2022-01-01 00:00:00
Maximum date: 2022-01-04 00:00:00
Conclusion
In this article, we explored how to get the min and max dates from a pandas DataFrame using Python’s pandas library. We first introduced pandas and explained its key data structure, the DataFrame. We then showed how to use the min()
and max()
functions to find the earliest and latest dates in a DataFrame column. This is a common task in data analysis and pandas provides a convenient way to accomplish it.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.