Finding the Column Name Corresponding to the Largest Value in a Pandas DataFrame
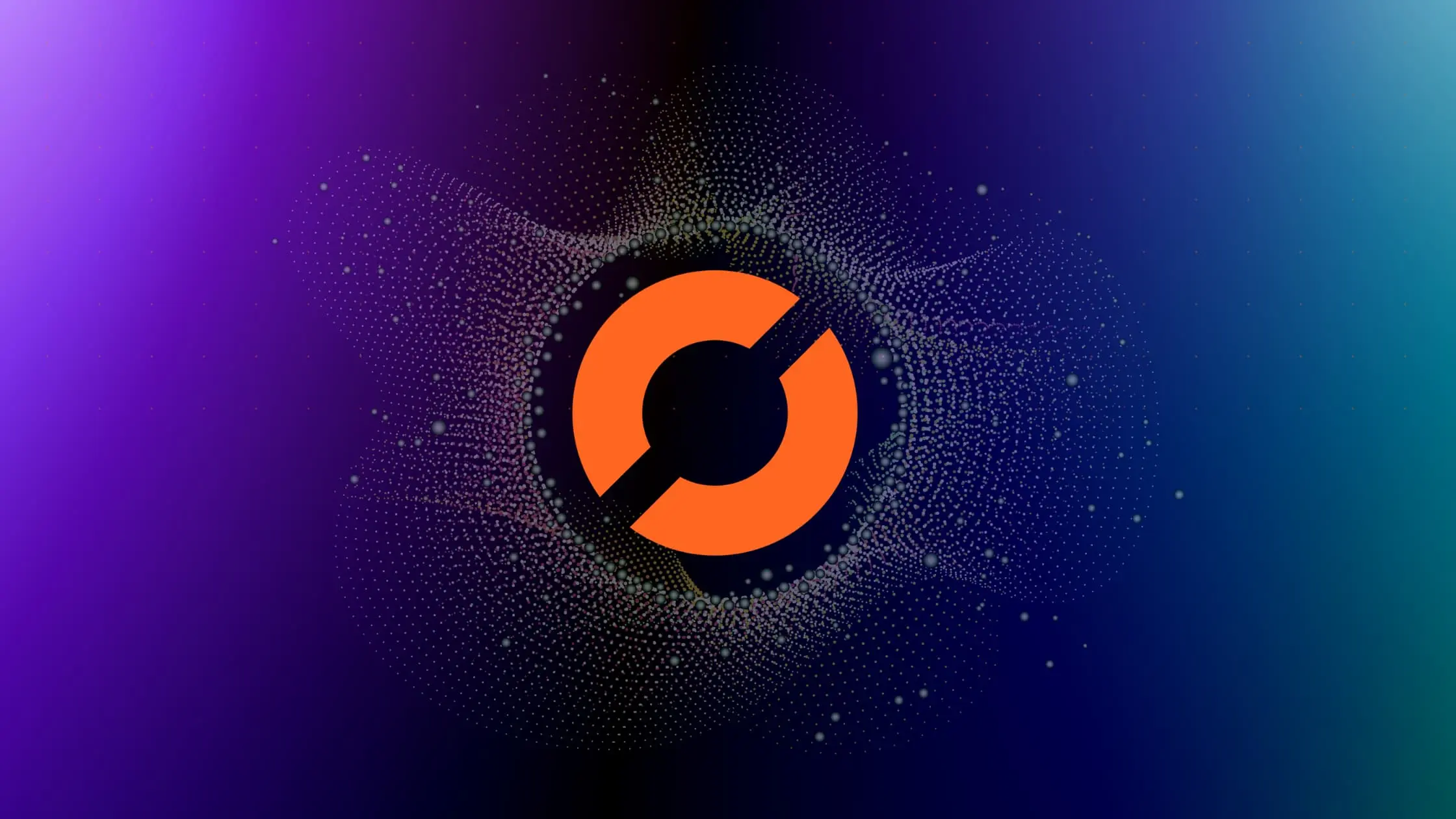
Pandas is a powerful Python library that provides flexible data structures to manipulate and analyze data. It’s a go-to tool for data scientists due to its ease of use and versatility. In this blog post, we’ll explore how to find the column name corresponding to the largest value in a Pandas DataFrame. This is a common task in data analysis, especially when dealing with large datasets where manual inspection is not feasible.
Table of Contents
- Prerequisites
- Creating a DataFrame
- Finding the Column with the Largest Value
- Handling Multiple Columns with the Same Maximum Value
- Common Errors and Handling Strategies
- Conclusion
Prerequisites
Before we dive in, make sure you have the following:
- Python installed (preferably Python 3.6 or later)
- Pandas library installed (you can install it using pip:
pip install pandas
)
Creating a DataFrame
First, let’s create a DataFrame to work with. We’ll use the pandas.DataFrame
function to create a DataFrame from a dictionary:
import pandas as pd
data = {
'A': [1, 2, 3, 4, 6],
'B': [5, 4, 3, 2, 1],
'C': [3, 3, 3, 3, 3]
}
df = pd.DataFrame(data)
Our DataFrame df
looks like this:
A B C
0 1 5 3
1 2 4 3
2 3 3 3
3 4 2 3
4 6 1 3
Finding the Column with the Largest Value
Method 1: Using idmax()
To find the column name corresponding to the largest value in the DataFrame, we can use the max()
function along with the idxmax()
function. The max()
function returns the highest value in each column, and idxmax()
returns the index of the first occurrence of the maximum value.
max_column = df.max().idxmax()
print(f"The column with the largest value is: {max_column}")
This will output: A
, as column ‘A’ contains the highest value in the DataFrame.
Method 2: Numpy’s argmax()
Function
Numpy’s argmax()
function can be utilized for finding the column index with the largest value. Here’s an example:
import pandas as pd
import numpy as np
# Finding the column with the largest value
max_column_index = np.argmax(df.values)
max_column = df.columns[max_column_index % len(df.columns)]
print(f"The column with the largest value is: {max_column}")
Handling Multiple Columns with the Same Maximum Value
What if multiple columns have the same maximum value? In this case, idxmax()
will return the first column name with the maximum value. If you want to get all column names with the maximum value, you can use a list comprehension:
import pandas as pd
data = {
'A': [1, 2, 3, 4, 5],
'B': [5, 4, 3, 2, 1],
'C': [3, 3, 3, 3, 3]
}
df = pd.DataFrame(data)
max_value = df.max().max()
max_value_columns = [col for col in df.columns if df[col].max() == max_value]
print(max_value_columns)
This will output: ['A', 'B']
, as both columns ‘A’ and ‘B’ contain the maximum value of 5.
Common Errors and Handling Strategies
Error 1: Non-Numeric Data in DataFrame
Error: If the DataFrame contains non-numeric data, the idxmax()
and argmax()
functions may raise an error.
Handling Strategy: Ensure the DataFrame only contains numeric data, or use appropriate data conversion techniques.
Error 2: Missing Values
Error: Presence of missing values (NaN) in the DataFrame can lead to unexpected results.
Handling Strategy: Clean the data by handling or removing missing values before applying any of the methods.
Conclusion
Pandas provides a robust set of tools for data manipulation and analysis. Finding the column name corresponding to the largest value in a DataFrame is a common task that can be accomplished easily using built-in Pandas functions. Whether you’re dealing with a small dataset or a large one, these techniques can help you quickly identify key features of your data.
Remember, the power of data science lies in the ability to extract meaningful insights from data. By mastering these fundamental operations in Pandas, you’re one step closer to becoming a proficient data scientist.
Further Reading
If you want to dive deeper into Pandas and its functionalities, here are some resources:
- Pandas Documentation
- Python for Data Analysis by Wes McKinney
- Data Wrangling with Pandas, NumPy, and IPython by J. VanderPlas
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.