Fastest way to copy columns from one DataFrame to another using pandas
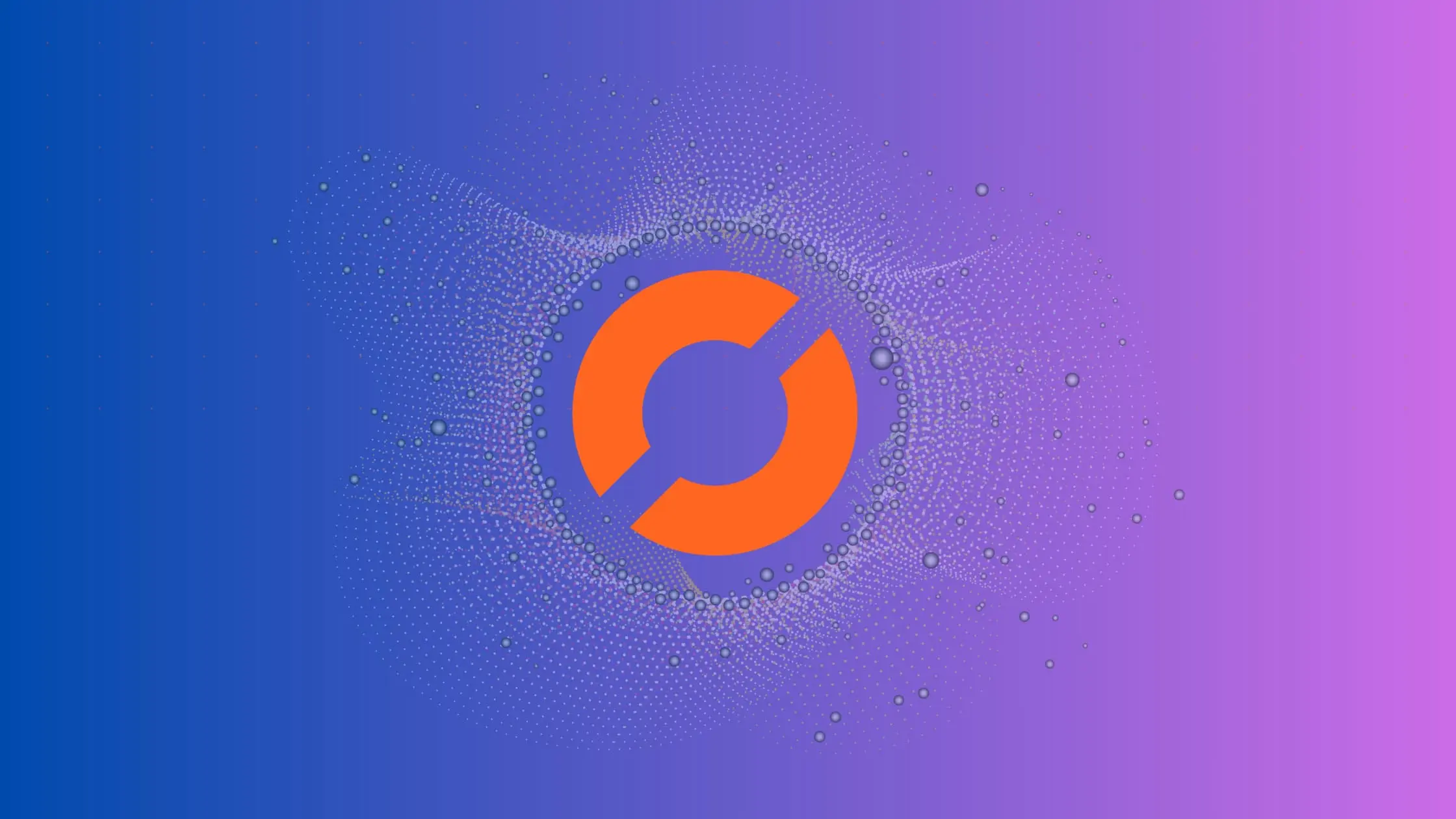
As a data scientist or software engineer, you have probably encountered a situation where you need to copy columns from one DataFrame to another. This is a common task when working with data, and pandas provides several ways to accomplish it. In this article, we will explore the fastest way to copy columns from one DataFrame to another using pandas.
Table of Contents
- What Is Pandas?
- How to Copy Columns from One DataFrame to Another
- Other Methods for Copying Columns in Pandas
- Conclusion
What Is Pandas?
Pandas is a powerful Python library for data manipulation and analysis. It provides data structures for efficiently storing and manipulating large datasets, as well as tools for data cleaning, transformation, and analysis. Pandas is widely used in data science and machine learning applications, and is an essential tool for any data scientist or software engineer working with data.
How to Copy Columns from One DataFrame to Another
There are several ways to copy columns from one DataFrame to another using pandas, but some methods are faster than others. In general, the fastest way to copy columns is to use the .loc
accessor, which allows you to access and modify specific rows and columns in a DataFrame.
Here is an example of how to copy columns from one DataFrame to another using .loc
:
import pandas as pd
# Create a sample DataFrame
df1 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]})
# Create a new DataFrame with only columns A and C from df1
df2 = pd.DataFrame()
df2.loc[:, 'A'] = df1.loc[:, 'A']
df2.loc[:, 'C'] = df1.loc[:, 'C']
print(df2)
Output:
A C
0 1 7
1 2 8
2 3 9
In this example, we first create a sample DataFrame df1
with three columns A, B, and C. We then create a new empty DataFrame df2
and use .loc
to copy only columns A and C from df1
to df2
.
By using .loc
to select specific columns, we avoid copying unnecessary data and improve the performance of our code. This method is particularly useful when working with large datasets, where efficiency is critical.
Other Methods for Copying Columns in Pandas
While using .loc
is the fastest way to copy columns in pandas, there are other methods that can be used depending on the specific requirements of your task.
Using the [] Operator
One common way to copy columns in pandas is to use the []
operator. This allows you to select specific columns by name or index, and copy them to a new DataFrame. Here is an example:
# Copy columns A and C from df1 to df2 using the [] operator
df2 = df1[['A', 'C']].copy()
Output:
A C
0 1 7
1 2 8
2 3 9
This method is simple and easy to understand, but can be slower than using .loc
when working with large datasets.
Using the copy() Method
Another way to copy columns in pandas is to use the copy()
method. This method creates a deep copy of the DataFrame, including all columns and rows. Here is an example:
# Create a deep copy of df1
df2 = df1.copy()
This method is useful when you need to create a completely separate copy of a DataFrame, but can be slower than using .loc
when you only need to copy specific columns.
Output:
A B C
0 1 4 7
1 2 5 8
2 3 6 9
Conclusion
In this article, we explored the fastest way to copy columns from one DataFrame to another using pandas. By using the .loc
accessor, we can select specific columns and avoid copying unnecessary data, improving the performance of our code. While other methods like the []
operator and the copy()
method can also be used, they may be slower when working with large datasets.
As a data scientist or software engineer, it is important to understand the different methods for copying columns in pandas and choose the one that best fits your specific requirements. By using the right method, you can improve the efficiency of your code and make the most of pandas' powerful data manipulation capabilities.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.