Creating a BLOB from a Base64 string in JavaScript
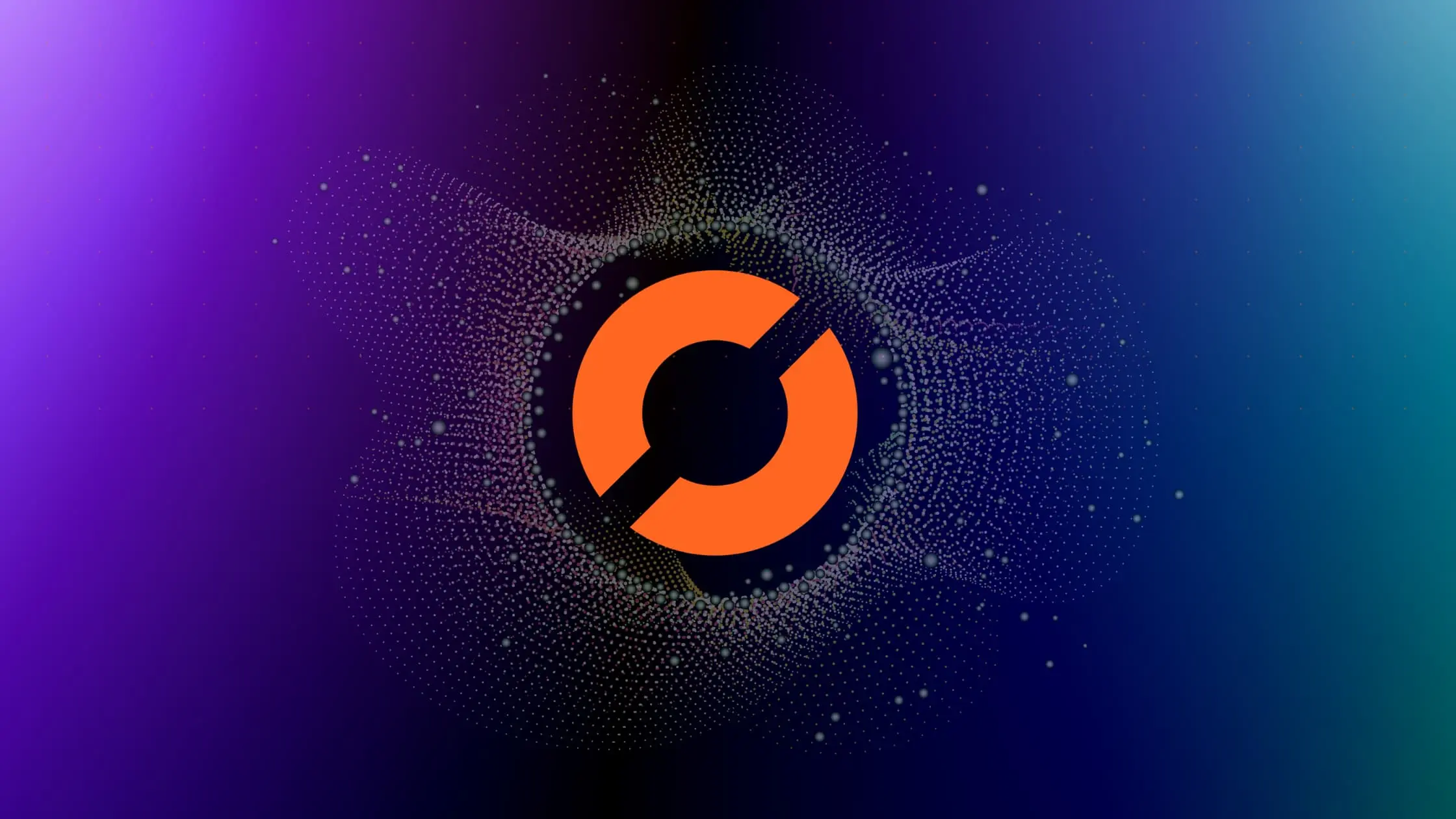
As a software engineer, you may come across a situation where you need to convert a Base64 string to a BLOB in JavaScript. This can be useful in many scenarios, such as when you need to store an image or a file in a database. In this blog post, we will discuss how to create a BLOB from a Base64 string in JavaScript.
Table of Contents
- What is a Base64 string?
- What is a BLOB?
- Converting a Base64 string to a BLOB in JavaScript
- Best Practices
- Common Errors and How to Handle Them
- Example
- Conclusion
What is a Base64 string?
A Base64 string is a way of representing binary data in ASCII format. It consists of a sequence of characters that represent a sequence of bytes. Each character in the string represents 6 bits of data. The total number of characters in the string is a multiple of 4. Base64 encoding is often used in web applications to transmit images, files, and other binary data.
What is a BLOB?
A BLOB (Binary Large Object) is a type of data that can store binary data such as images, videos, and files. BLOBs are often used in databases to store large amounts of binary data.
Converting a Base64 string to a BLOB in JavaScript
To convert a Base64 string to a BLOB in JavaScript, we can use the atob()
and Blob()
functions.
The atob()
function decodes a Base64 string into a binary data string. It takes a Base64 string as input and returns a string of binary data.
The Blob()
function creates a new BLOB object. It takes an array of binary data as input and returns a new BLOB object.
Here’s an example of how to convert a Base64 string to a BLOB in JavaScript:
const byteCharacters = atob(base64String);
const byteArrays = [];
for (let i = 0; i < byteCharacters.length; i++) {
byteArrays.push(byteCharacters.charCodeAt(i));
}
const byteArray = new Uint8Array(byteArrays);object
This code snippet converts a Base64-encoded string (base64String
) into a Binary Large Object (Blob) in JavaScript. Initially, the atob
function is used to decode the Base64 string into a series of binary characters (byteCharacters
). Subsequently, a loop iterates through each character, obtaining its Unicode value, and populates an array (byteArrays
) with these numeric values. The Uint8Array
constructor is then employed to create an array of 8-bit unsigned integers (byteArray
) from the obtained numeric values. Finally, a Blob is generated using the Blob
constructor, taking the Uint8Array as input and allowing the specification of a content type, resulting in a Blob suitable for various applications, such as saving binary data as files.
Best Practices
Specify Content Type: When creating a BLOB, always specify the content type using the
Blob
constructor. This helps browsers interpret the data correctly.Handle Asynchronous Operations: If decoding or processing the Base64 string involves asynchronous operations, ensure proper error handling and use
async/await
or Promises.Cross-Browser Compatibility: Test your code across different browsers to ensure compatibility. Different browsers may have variations in their implementation of JavaScript functions.
Common Errors and How to Handle Them
Error 1: Invalid Base64 String
One common error is providing an invalid Base64 string. To handle this, check the validity of the string before attempting to decode it.
Error 2: Data URI Mismatch
Ensure that the data URI and the content type specified in the Blob
constructor match. Mismatched types can lead to unexpected behavior.
Error 3: Cross-Origin Security Issues
When working with Base64 strings from external sources, be aware of cross-origin security restrictions. Ensure that your application has the necessary permissions to access external resources.
Example
Let’s create a simple html file that has a function to decode base64 of Hello world
and save it to a text file.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Base64 to Blob and Save as Text File</title>
</head>
<body>
<script>
// Function to convert Base64 to Blob
function base64ToBlob(base64String, contentType = '') {
const byteCharacters = atob(base64String);
const byteArrays = [];
for (let i = 0; i < byteCharacters.length; i++) {
byteArrays.push(byteCharacters.charCodeAt(i));
}
const byteArray = new Uint8Array(byteArrays);
return new Blob([byteArray], { type: contentType });
}
// Function to save Blob as a text file
function saveBlobAsTextFile(blob, fileName) {
const link = document.createElement('a');
link.href = URL.createObjectURL(blob);
link.download = fileName;
link.click();
}
// Example Base64 string of a text file
const base64TextFile = "SGVsbG8gd29ybGQ="; // "Hello world"
// Specify the content type (text/plain for a text file)
const contentType = "text/plain";
// Convert Base64 to Blob
const blob = base64ToBlob(base64TextFile, contentType);
// Save Blob as a text file
saveBlobAsTextFile(blob, "example.txt");
</script>
</body>
</html>
Open this html file on your browser, it will download a text file that has Hello world
inside.
Conclusion
In this blog post, we discussed how to create a BLOB from a Base64 string in JavaScript. We learned that a Base64 string is a way of representing binary data in ASCII format, and a BLOB is a type of data that can store binary data such as images, videos, and files. We also learned that we can use the atob()
and Blob()
functions to convert a Base64 string to a BLOB in JavaScript.
By following the steps outlined in this post, you can easily convert a Base64 string to a BLOB in JavaScript and use it in your web applications. This can be particularly useful when working with databases that store binary data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.