Counting Rows in PySpark DataFrames: A Guide
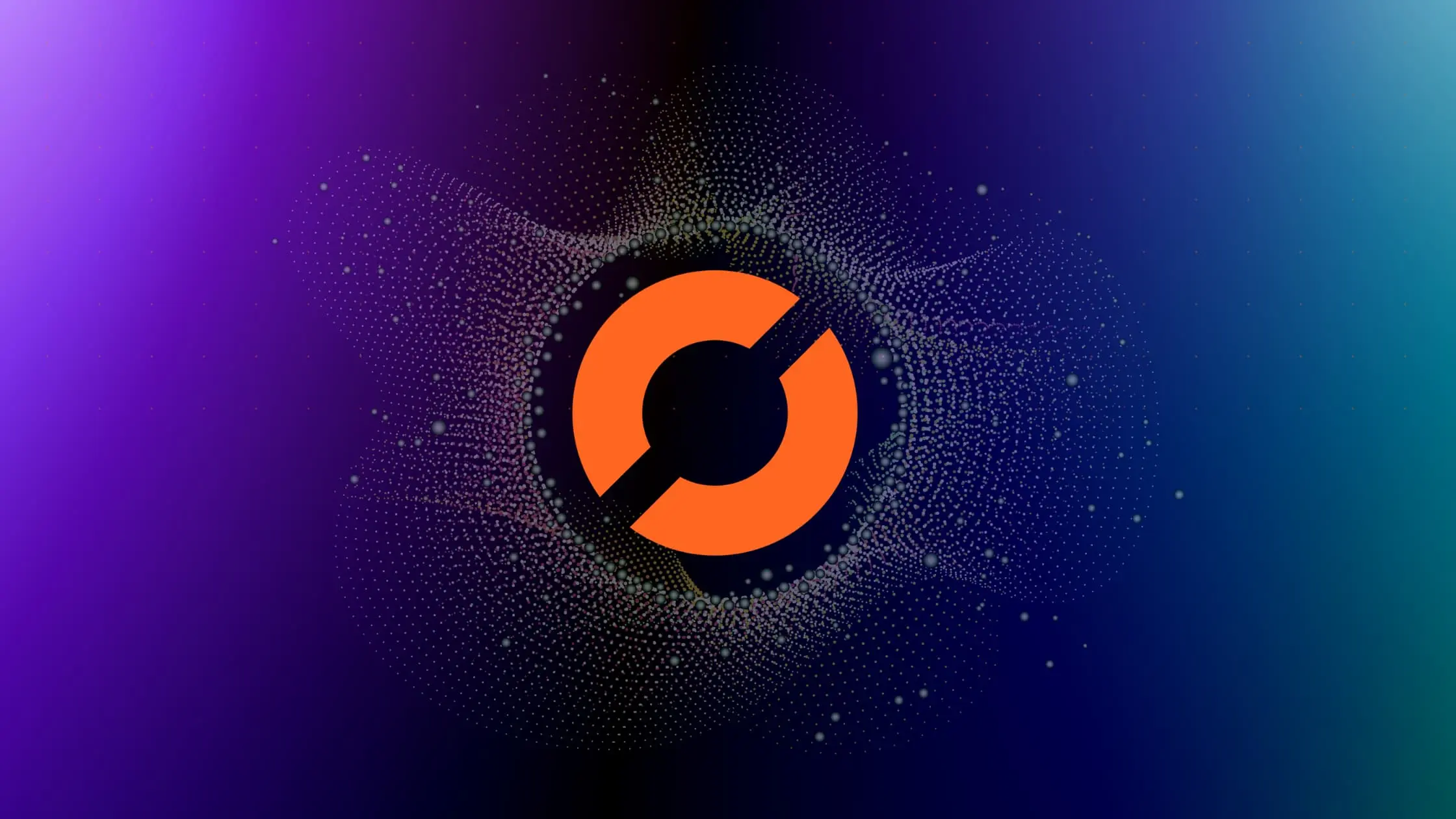
Counting Rows in PySpark DataFrames: A Guide
Data science is a field that’s constantly evolving, with new tools and techniques being introduced regularly. One such tool that has gained popularity in recent years is Apache Spark, and more specifically, its Python library, PySpark. In this blog post, we’ll delve into one of the fundamental operations in PySpark: counting rows in a DataFrame.
What is PySpark?
Before we dive into the specifics, let’s briefly discuss what PySpark is. PySpark is the Python library for Apache Spark, an open-source, distributed computing system used for big data processing and analytics. PySpark allows data scientists to write Spark applications using Python APIs, making it a popular choice for handling large datasets.
Why Count Rows in PySpark DataFrames?
Counting rows in a DataFrame is a common operation in data analysis. It helps in understanding the size of the dataset, identifying missing values, and performing exploratory data analysis. In PySpark, there are several ways to count rows, each with its own advantages and use cases.
Counting Rows Using the count() Function
The simplest way to count rows in a PySpark DataFrame is by using the count()
function. Here’s how you can do it:
from pyspark.sql import SparkSession
# Initialize SparkSession
spark = SparkSession.builder.appName('count_rows').getOrCreate()
# Load DataFrame
df = spark.read.csv('data.csv', header=True, inferSchema=True)
# Count rows
row_count = df.count()
print(f'The DataFrame has {row_count} rows.')
print(f'-'*30)
df.show()
Output:
The DataFrame has 6 rows.
------------------------------
+----+----+----+----+
|col1|col2|col3|col4|
+----+----+----+----+
| x| 15| a| 20|
| y| 16| b| 18|
| x| 17| c| 16|
| y| 18| d| 14|
| x| 19| e| 12|
| x| 20| f| 10|
+----+----+----+----+
The count()
function returns the total number of rows in the DataFrame. It’s straightforward and easy to use, but it performs a full scan of the data, which can be time-consuming for large datasets.
Counting Rows Using SQL Queries
If you’re comfortable with SQL, you can also use SQL queries to count rows in a PySpark DataFrame. Here’s an example:
# Register DataFrame as a SQL temporary view
df.createOrReplaceTempView('data')
# Count rows using SQL query
row_count = spark.sql('SELECT COUNT(*) FROM data').collect()[0][0]
print(f'The DataFrame has {row_count} rows.')
Output
The DataFrame has 6 rows.
This method is useful if you’re already using SQL queries in your data analysis, as it allows you to keep your code consistent.
Counting Rows Using the rdd
Attribute
Another way to count rows in a PySpark DataFrame is by using the rdd
attribute and the count()
function. Here’s how:
# Count rows using rdd attribute
row_count = df.rdd.count()
print(f'The DataFrame has {row_count} rows.')
Output
The DataFrame has 6 rows.
This method converts the DataFrame to an RDD (Resilient Distributed Dataset), then counts the number of elements in the RDD. It’s a bit more complex than the previous methods, but it can be useful in certain situations.
Conclusion
Counting rows in a PySpark DataFrame is a fundamental operation in data analysis. Whether you’re using the count()
function, SQL queries, or the rdd
attribute, PySpark provides several ways to count rows, each with its own advantages and use cases.
The method you choose should depend on your specific needs and the size of your dataset. For small datasets, the count()
function is usually sufficient. For larger datasets, you might want to consider using SQL queries or the rdd
attribute to improve performance.
We hope this guide has helped you understand how to count rows in PySpark DataFrames. Stay tuned for more PySpark tutorials and tips!
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.