Converting XML to Python DataFrame: A Guide
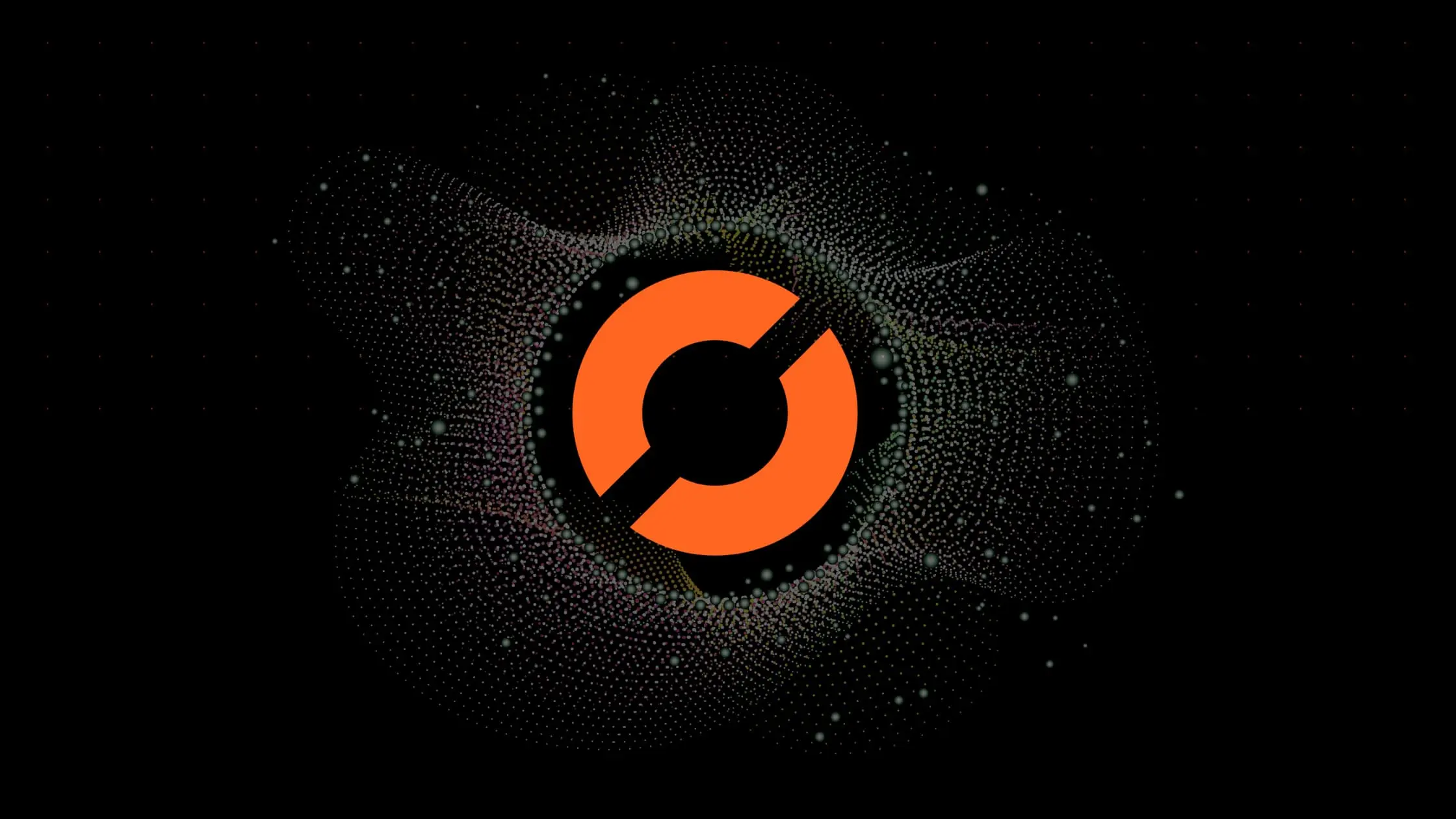
Data scientists often encounter a variety of data formats in their work, one of which is XML. XML, or Extensible Markup Language, is a common data format used for storing and transporting data. However, converting XML data into a Python DataFrame can sometimes be a challenging task. This blog post will guide you through the process of converting XML to a Python DataFrame, making your data analysis tasks easier and more efficient.
Understanding XML and Python DataFrame
Before we delve into the conversion process, let’s briefly understand what XML and Python DataFrame are.
XML (Extensible Markup Language) is a markup language that defines a set of rules for encoding documents in a format that is both human-readable and machine-readable. It is widely used in web services, configuration files, and document storage.
Python DataFrame, on the other hand, is a two-dimensional size-mutable, potentially heterogeneous tabular data structure with labeled axes (rows and columns). It is a primary data structure in pandas, a software library written for data manipulation and analysis in Python.
Why Convert XML to Python DataFrame?
Converting XML data to a Python DataFrame allows data scientists to leverage the powerful data manipulation and analysis capabilities of pandas. With data in a DataFrame, you can perform operations like filtering, sorting, aggregating, merging, and visualization with ease.
Step-by-Step Guide to Convert XML to Python DataFrame
Let’s say we have the following xml
file:
<library>
<book id="1">
<title>Python for Data Science</title>
<author>John Doe</author>
<genre>Data Science</genre>
<price>29.99</price>
</book>
<book id="2">
<title>Machine Learning Basics</title>
<author>Jane Smith</author>
<genre>Machine Learning</genre>
<price>39.99</price>
</book>
</library>
Step 1: Import Necessary Libraries
First, we need to import the necessary libraries. We’ll need pandas
for creating and manipulating DataFrames, and xml.etree.ElementTree
for parsing and creating XML data.
import pandas as pd
import xml.etree.ElementTree as ET
Step 2: Parse the XML File
Next, we parse the XML file using the parse()
function from the ElementTree
module. This function returns an ElementTree
object, which represents the whole XML document.
tree = ET.parse('dataset.xml')
root = tree.getroot()
Step 3: Extract Data
Now, we need to extract the data from the XML file. We can do this by iterating over the XML tree, accessing the tags and text of each element.
# Create a list to store dictionaries representing each book
books_list = []
# Iterate through each <book> element
for book_elem in root.findall('.//book'):
book_dict = {}
book_dict['id'] = book_elem.get('id')
for child_elem in book_elem:
book_dict[child_elem.tag] = child_elem.text
books_list.append(book_dict)
Step 4: Convert to DataFrame
Finally, we can convert the extracted data into a DataFrame using the DataFrame()
function from pandas.
# Create a Pandas DataFrame from the list of dictionaries
df = pd.DataFrame(data)
# Display the DataFrame
print(df)
Output:
id title author genre price
0 1 Python for Data Science John Doe Data Science 29.99
1 2 Machine Learning Basics Jane Smith Machine Learning 39.99
And there you have it! Your XML data is now in a Python DataFrame, ready for analysis.
Conclusion
Converting XML to a Python DataFrame can be a bit tricky, but with the right approach, it becomes a straightforward task. This guide has shown you how to parse an XML file, extract the necessary data, and convert it into a DataFrame using pandas. With this knowledge, you can now easily handle XML data in your data analysis projects.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.