Converting Tensorflow Model to PyTorch Model
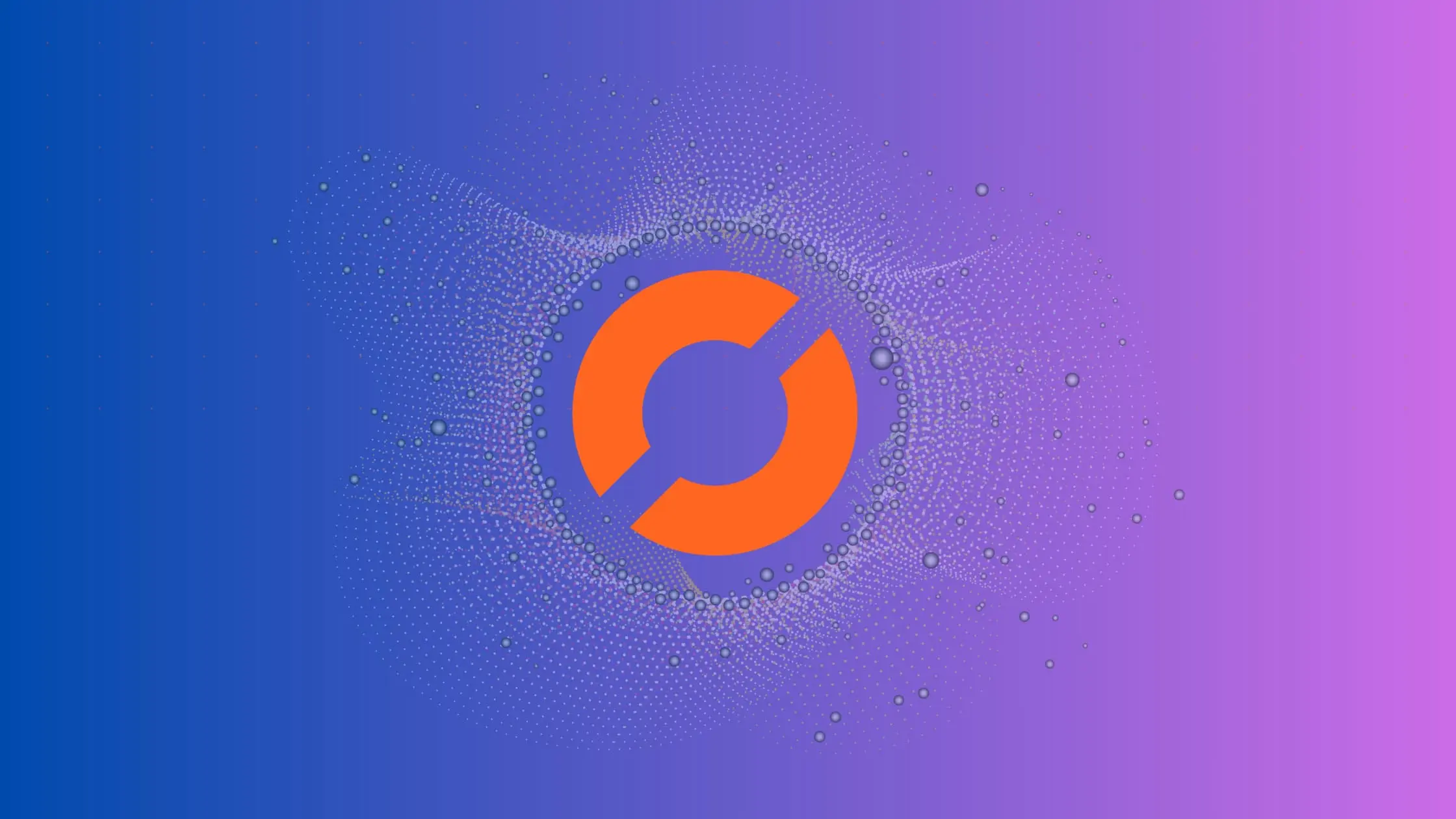
As a data scientist, you may have come across situations where you need to convert a Tensorflow model to a PyTorch model. This could be due to a variety of reasons, such as wanting to take advantage of PyTorch’s dynamic computation graph, or wanting to use PyTorch’s ecosystem of libraries and tools. In this blog post, we will discuss the steps involved in converting a Tensorflow model to a PyTorch model.
Table of Contents
- Why Convert?
- Step-by-Step Converting Tensorflow Model to PyTorch Model
- Best Practices for Model Conversion
- Handling Common Errors
- Conclusion
Why Convert?
Framework Preferences
Teams or individuals may have a preference for one framework over another due to factors such as ease of use, community support, or specific features. Converting a model allows practitioners to leverage the capabilities of PyTorch while preserving the knowledge and work invested in a Tensorflow model.
Research and Collaboration
In collaborative environments, different teams or collaborators may be working with different frameworks. Converting models ensures seamless collaboration and knowledge sharing across diverse technical stacks.
Deployment Considerations
The deployment ecosystem may favor one framework over another. Converting a Tensorflow model to PyTorch might be necessary to align with the deployment infrastructure or to take advantage of specific deployment tools and optimizations.
Library Ecosystem
The availability of specific libraries or tools in one framework but not in another could be a driving factor. Converting allows practitioners to tap into the rich ecosystem of PyTorch libraries while maintaining the core model architecture.
Let’s go through each of these steps in detail.
Step-by-Step Converting Tensorflow Model to PyTorch Model
Setting Up the Environment
Before we delve into the conversion process, let’s ensure our environment is set up correctly. Install the necessary packages using:
pip install tensorflow torch
Training a Tensorflow Model
To keep this blog concise, we won’t go into the details of model training. However, ensure you have a trained Tensorflow model before proceeding.
Saving and Loading Tensorflow Model
Save your trained Tensorflow model using:
import tensorflow as tf
model = ... # Your Tensorflow model
model.save("tf_model.h5")
Load the model back for conversion:
loaded_model = tf.keras.models.load_model("tf_model.h5")
Converting to PyTorch Model
Installing Necessary Libraries
Install the tf2onnx
library for converting Tensorflow models to ONNX format:
pip install tf2onnx
Loading Tensorflow Model
import tf2onnx
# Convert the model to ONNX format
onnx_model, _ = tf2onnx.convert.from_keras(loaded_model)
Converting to PyTorch Model
import onnx
from onnx2pytorch import ConvertModel
# Load ONNX model
onnx_model = onnx.load_model("tf_model.onnx")
# Convert ONNX model to PyTorch
pytorch_model = ConvertModel(onnx_model)
Best Practices for Model Conversion
- Ensure both Tensorflow and PyTorch versions are up-to-date.
- Double-check layer compatibility between frameworks.
- Test the converted model with sample data to verify correctness.
Handling Common Errors
Error 1: Shape Mismatch
If you encounter shape mismatches during conversion, double-check layer configurations and input shapes. Use reshape operations or adjust layer parameters accordingly.
Error 2: Unsupported Operations
Some operations may not have direct equivalents in PyTorch. Identify these operations and implement custom layers or find alternative PyTorch functions.
Error 3: Data Format Differences
Tensor data format (NHWC vs. NCHW) may differ between TensorFlow and PyTorch. Adjust data formats as needed to prevent runtime errors.
Conclusion
Converting a Tensorflow model to a PyTorch model can be a useful technique for data scientists who want to take advantage of PyTorch’s dynamic computation graph or use its ecosystem of libraries and tools. In this blog post, we discussed the steps involved in converting a Tensorflow model to a PyTorch model, which include exporting the Tensorflow model to a format that can be imported into PyTorch, loading the exported model into PyTorch, converting the model’s weights and structure to PyTorch format, and saving the PyTorch model. By following these steps, data scientists can easily convert their Tensorflow models to PyTorch models and take advantage of the benefits that PyTorch has to offer.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.