Converting Pandas DataFrame to JSON Object Column: A Guide
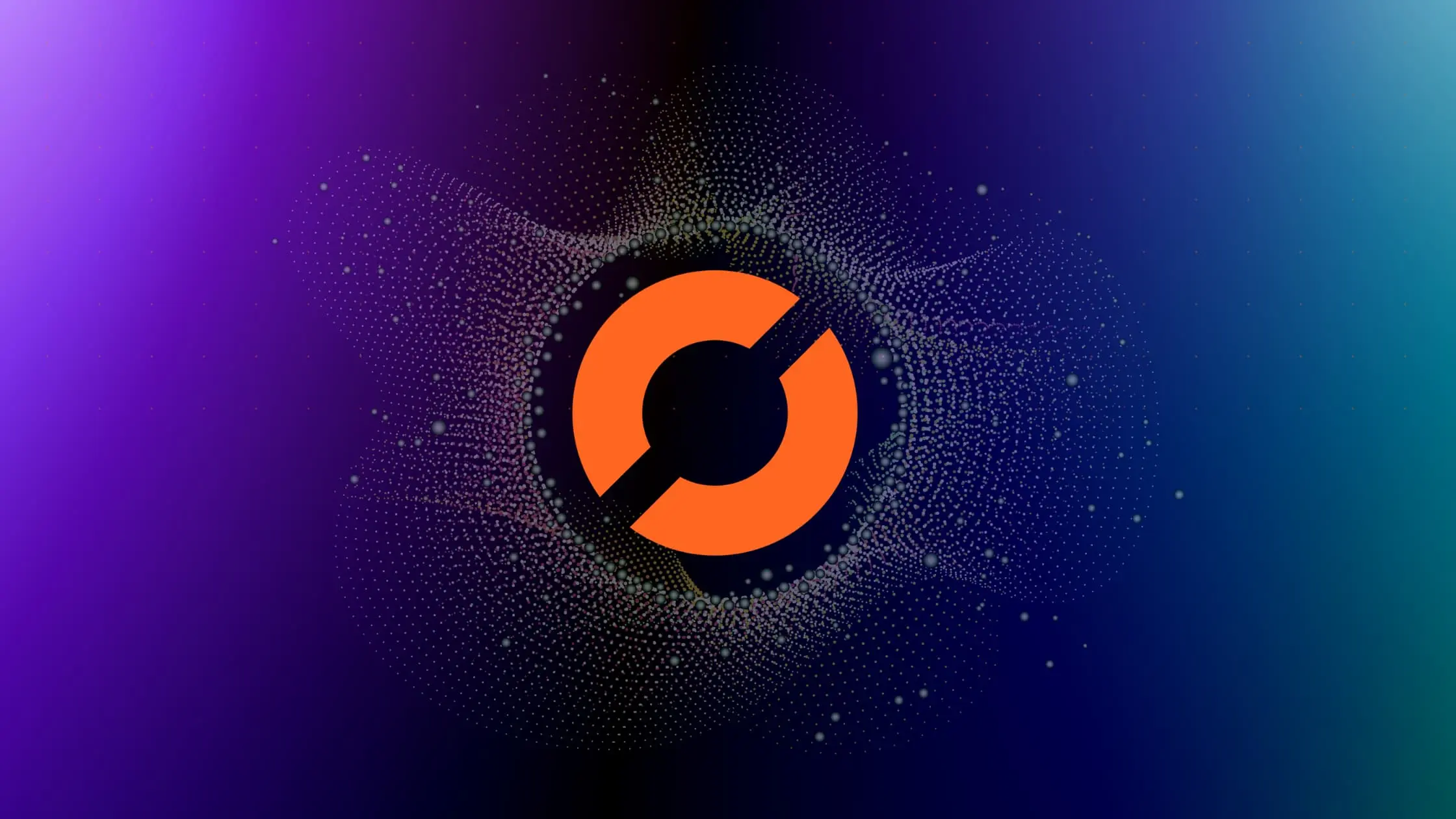
Data scientists often encounter the need to convert a Pandas DataFrame to a JSON object column. This conversion is crucial when dealing with complex data structures that are not easily represented in a tabular format. This blog post will guide you through the process, step by step.
Table of Contents
- Why Convert Pandas DataFrame to JSON Object Column?
- Step-by-Step Guide to Converting DataFrame to JSON Object Column
- Best Practices for Converting DataFrame to JSON
- Conclusion
Why Convert Pandas DataFrame to JSON Object Column?
Before we dive into the how, let’s understand the why. JSON (JavaScript Object Notation) is a lightweight data-interchange format that is easy for humans to read and write and easy for machines to parse and generate. It is often used when data is sent from a server to a web page.
Pandas is a powerful data manipulation library in Python. However, when dealing with nested data or data that doesn’t fit neatly into a table, JSON can be a more suitable format. By converting a DataFrame to a JSON object column, you can handle complex data structures more efficiently.
Step-by-Step Guide to Converting DataFrame to JSON Object Column
Step 1: Import Necessary Libraries
First, we need to import the necessary libraries. We will need Pandas for data manipulation and json for handling JSON data.
import pandas as pd
import json
Step 2: Create a DataFrame
Next, let’s create a simple DataFrame for demonstration purposes.
data = {'Name': ['John', 'Anna', 'Peter'],
'Age': [28, 24, 22],
'Occupation': ['Engineer', 'Doctor', 'Student']}
df = pd.DataFrame(data)
Step 3: Convert DataFrame to JSON
Now, we can convert the DataFrame to a JSON object. We use the to_json()
function, which converts the DataFrame to a JSON string. We will also use the orient='records'
parameter to create a list of records in the JSON string.
json_str = df.to_json(orient='records')
Step 4: Convert JSON String to JSON Object
The to_json()
function returns a JSON string. To convert this string to a JSON object, we use the json.loads()
function.
json_obj = json.loads(json_str)
Step 5: Add JSON Object as a Column in DataFrame
Finally, we can add the JSON object as a new column in the DataFrame. We use the apply()
function to apply a function across the DataFrame’s rows.
df['JSON_Object'] = df.apply(lambda row: json.dumps(row.to_dict()), axis=1)
print(df)
And that’s it! You have successfully converted a Pandas DataFrame to a JSON object column.
Output:
Name Age Occupation JSON_Object
0 John 28 Engineer {"Name": "John", "Age": 28, "Occupation": "Engineer"}
1 Anna 24 Doctor {"Name": "Anna", "Age": 24, "Occupation": "Doctor"}
2 Peter 22 Student {"Name": "Peter", "Age": 22, "Occupation": "Student"}
Best Practices for Converting DataFrame to JSON
Before diving into the methods, it’s essential to follow some best practices:
- Ensure your DataFrame is well-structured with appropriate column names.
- Handle missing or null values appropriately to avoid unexpected results.
Conclusion
Converting a Pandas DataFrame to a JSON object column can be a powerful tool when dealing with complex data structures. This guide has shown you how to perform this conversion step by step. Remember, the key is to understand your data and choose the right tools for the job.
If you found this guide helpful, please share it with your fellow data scientists. And stay tuned for more practical guides on data manipulation and analysis.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.