Converting a Column to Date Format in Pandas Dataframe
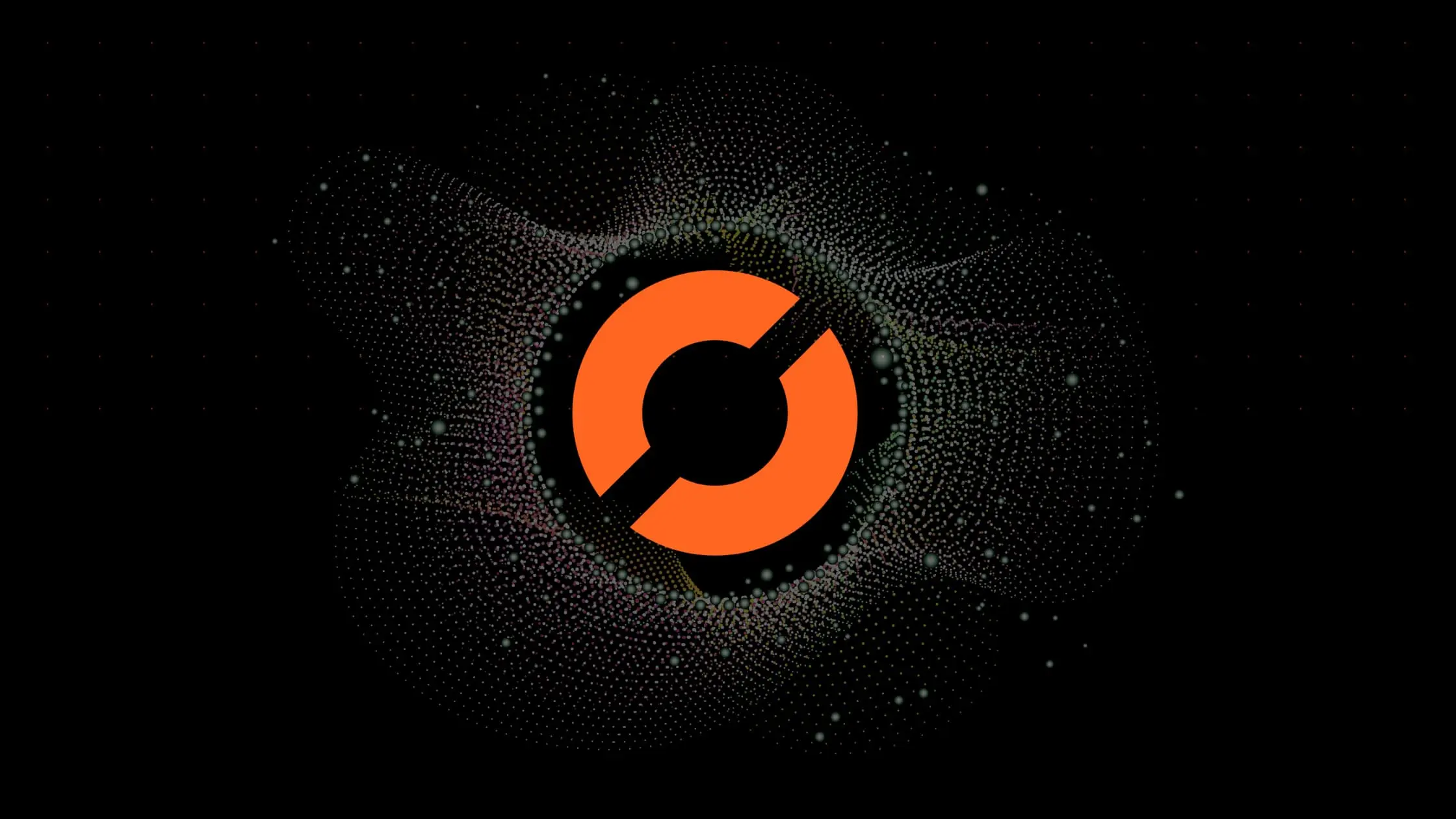
Converting a Column to Date Format in Pandas Dataframe
As a data scientist, working with time-series data is an inevitable part of the job. However, parsing and manipulating dates can be challenging, especially when dealing with data from multiple sources. This is where Pandas, a popular data manipulation library in Python, comes in handy. In this blog post, we will discuss how to convert a column to date format in a Pandas dataframe.
Why do we need to convert a column to date format?
Before we dive into the details of how to convert a column to date format, let us first understand why we need to do so. Dates can be represented in different formats, such as “YYYY-MM-DD”, “MM/DD/YYYY”, “DD-MM-YYYY”, etc. When working with time-series data, it is crucial to ensure that the date format is consistent across all the data sources. Moreover, converting a column to date format allows us to perform various date-related operations, such as date arithmetic, filtering by date range, and aggregation by date.
Prerequisites
Before we begin, make sure that you have Pandas installed on your system. You can install it by running the following command in your terminal:
pip install pandas
Also, make sure that you have a dataset with a date column that needs to be converted to date format.
Method 1: Using the to_datetime() function
The easiest and most straightforward way to convert a column to date format is to use the to_datetime()
function provided by Pandas. The to_datetime()
function can parse various date formats automatically and convert them to a standard datetime format.
The following code snippet demonstrates how to use the to_datetime()
function to convert a column to date format:
import pandas as pd
# Load the dataset
df = pd.read_csv("dataset.csv")
# Convert the date column to date format
df["date"] = pd.to_datetime(df["date"])
In the above code snippet, we first load the dataset using the read_csv()
function provided by Pandas. Next, we use the to_datetime()
function to convert the “date” column to date format. The to_datetime()
function returns a Pandas datetime object, which we assign back to the “date” column of the dataframe.
Method 2: Using the dateutil parser
In some cases, the to_datetime()
function may not be able to parse the date format correctly. For example, if the date format is not in a standard format or contains text, the to_datetime()
function may raise an error. In such cases, we can use the dateutil
parser, which is a third-party library that can parse various date formats.
The following code snippet demonstrates how to use the dateutil
parser to convert a column to date format:
import pandas as pd
from dateutil.parser import parse
# Load the dataset
df = pd.read_csv("dataset.csv")
# Convert the date column to date format using dateutil parser
df["date"] = df["date"].apply(lambda x: parse(x))
In the above code snippet, we first load the dataset using the read_csv()
function provided by Pandas. Next, we use the apply()
function to apply the parse()
function from the dateutil
parser to each row of the “date” column. The parse()
function can parse various date formats and return a datetime object. Finally, we assign the datetime object back to the “date” column of the dataframe.
Conclusion
In this blog post, we discussed how to convert a column to date format in a Pandas dataframe. We demonstrated two methods: using the to_datetime()
function provided by Pandas and using the dateutil
parser, a third-party library. Converting a column to date format is essential when working with time-series data. It ensures that the date format is consistent across all the data sources and allows us to perform various date-related operations. We hope that this blog post will help you in your data science journey.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.