Streamlining Data Preparation: How to Set Column Names in a Pandas DataFrame from the First Row
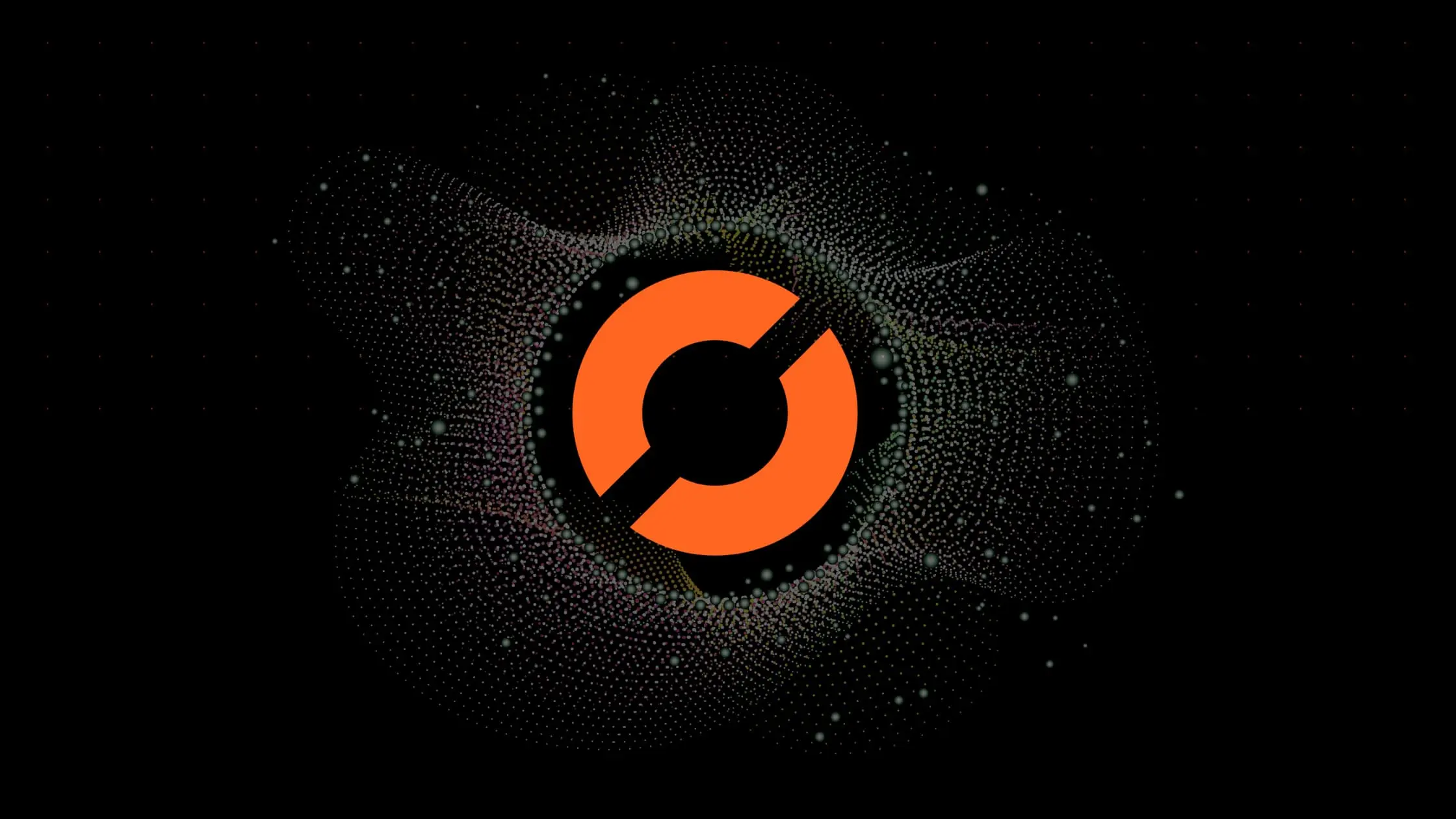
Introduction
Pandas is a powerful Python library for data manipulation and analysis. It provides flexible data structures that make it easy to work with structured (tabular, multidimensional, potentially heterogeneous) and time series data. One of the most common tasks when working with pandas is to convert the first row of a DataFrame to column names. This can be useful when your data doesn’t come with a header row, or when you want to change the column names to something more meaningful.
Step 1: Importing the Necessary Libraries
First, we need to import the pandas library. If you haven’t installed it yet, you can do so using pip:
pip install pandas
Then, import the library in your Python script:
import pandas as pd
Step 2: Loading the Data
Next, we need to load the data into a pandas DataFrame. You can do this from a variety of sources, such as a CSV file, an Excel file, or a SQL database. For this example, let’s assume we’re loading data from a CSV file:
df = pd.read_csv('data.csv')
The dataframe would look like this:
0 Product Sales
1 Apple 15
2 Banana 22
3 Cherry 8
Step 3: Converting the First Row to Column Names
Now, let’s convert the first row of the DataFrame to column names. We can do this using the .iloc
and .columns
properties of the DataFrame:
df.columns = df.iloc[0]
df = df[1:]
The .iloc
property is used to access the DataFrame by integer-location based indexing, allowing us to select the first row. We then assign this row to the .columns
property, which sets the column names of the DataFrame.
Step 4: Resetting the Index
After converting the first row to column names, the index of the DataFrame will be off by one. To fix this, we can use the .reset_index
method:
df = df.reset_index(drop=True)
Once you run this code, ‘df’ will have a neat, reset index:
Product Sales
0 Apple 15
1 Banana 22
2 Cherry 8
The drop=True
argument is used to avoid the old index being added as a new column in the DataFrame.
Conclusion
And that’s it! You’ve successfully converted the first row of a pandas DataFrame to column names. This is a simple but powerful technique that can make your data easier to work with.
Remember, pandas is a versatile library with many more features to explore. Whether you’re a beginner or an experienced data scientist, there’s always more to learn. So keep exploring, keep experimenting, and keep pushing the boundaries of what’s possible with data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.