Convert Pandas Column to DateTime A Guide
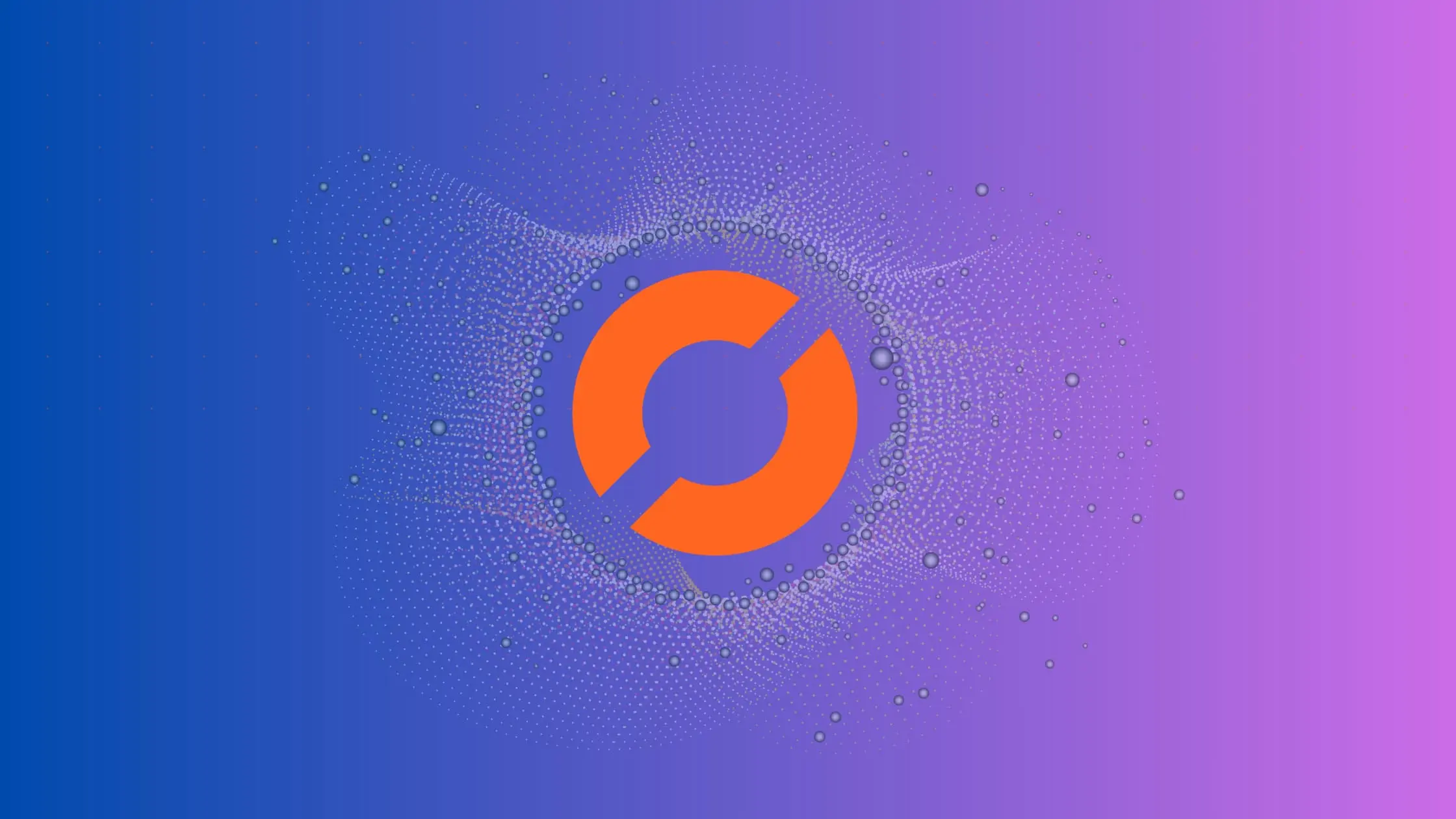
As a data scientist or software engineer, you are likely to work with data in different formats, including text, numerical, and datetime data. In this article, we will focus on datetime data and how to convert pandas columns to datetime format.
Table of Contents
- Introduction 1.1 What is Pandas?
- Understanding Datetime in Pandas
- Converting Pandas Columns to Datetime
3.1 Using
to_datetime()
Function 3.2 Handling Datetime Formats - Conclusion
What is Pandas?
Pandas is a popular data manipulation library in Python used to analyze, manipulate, and transform data. It provides data structures for efficient data manipulation and analysis, including the Series and DataFrame objects. Pandas is widely used in data science, machine learning, and other related fields.
Understanding Datetime in Pandas
Datetime data is a fundamental data type used to represent dates and times. In Pandas, datetime data is represented using the datetime64
data type, which provides high precision and efficient storage of datetime data.
Datetime data is often represented in different formats, including ISO 8601 format, which is a standard format used to represent datetime data. Pandas provides a range of functions to convert datetime data to different formats, including to_datetime()
, strftime()
, and date_range()
.
Converting Pandas Columns to Datetime
To convert a pandas column to datetime format, we need to use the to_datetime()
function, which is a powerful function that can convert a wide range of datetime formats to the datetime64
data type.
The to_datetime()
function takes a pandas column as input and returns a new column with datetime values. The function can also handle missing or invalid datetime values by setting them to NaT
, which represents missing or invalid datetime data.
Here is an example of how to convert a pandas column to datetime format using the to_datetime()
function:
import pandas as pd
# create a sample dataframe with date column
data = {'date': ['2021-01-01', '2021-01-02', '2021-01-03']}
df = pd.DataFrame(data)
# convert date column to datetime format
df['date'] = pd.to_datetime(df['date'])
# print the dataframe
print(df)
Output:
date
0 2021-01-01
1 2021-01-02
2 2021-01-03
In this example, we create a sample dataframe with a date column and convert the date column to datetime format using the to_datetime()
function. We then print the resulting dataframe, which shows the date column in datetime format.
Handling Datetime Formats
The to_datetime()
function can handle a wide range of datetime formats, including ISO 8601 format, which is a standard format used to represent datetime data. However, it is essential to understand the datetime format of your data to ensure that the to_datetime()
function can parse the datetime values correctly.
If the datetime format of your data is not recognized by the to_datetime()
function, you can specify the datetime format using the format
parameter. The format
parameter takes a string that specifies the datetime format of your data.
Here is an example of how to convert a pandas column to datetime format with a custom datetime format using the format
parameter:
import pandas as pd
# create a sample dataframe with date column
data = {'date': ['01-01-2021', '02-01-2021', '03-01-2021']}
df = pd.DataFrame(data)
# convert date column to datetime format with custom format
df['date'] = pd.to_datetime(df['date'], format='%m-%d-%Y')
# print the dataframe
print(df)
Output:
date
0 2021-01-01
1 2021-02-01
2 2021-03-01
In this example, we create a sample dataframe with a date column and convert the date column to datetime format with a custom format using the format
parameter. We then print the resulting dataframe, which shows the date column in datetime format with the custom format specified.
Conclusion
Datetime data is an essential data type used to represent dates and times, and converting pandas columns to datetime format is a common task in data manipulation and analysis. In this article, we have explored the to_datetime()
function, which is a powerful function that can convert a wide range of datetime formats to the datetime64
data type. We have also discussed how to handle datetime formats using the format
parameter.
By understanding how to convert pandas columns to datetime format, you can effectively manipulate and analyze datetime data in your data science and software engineering projects.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.