Conditional Replacement in Pandas A Quick Guide for Data Scientists
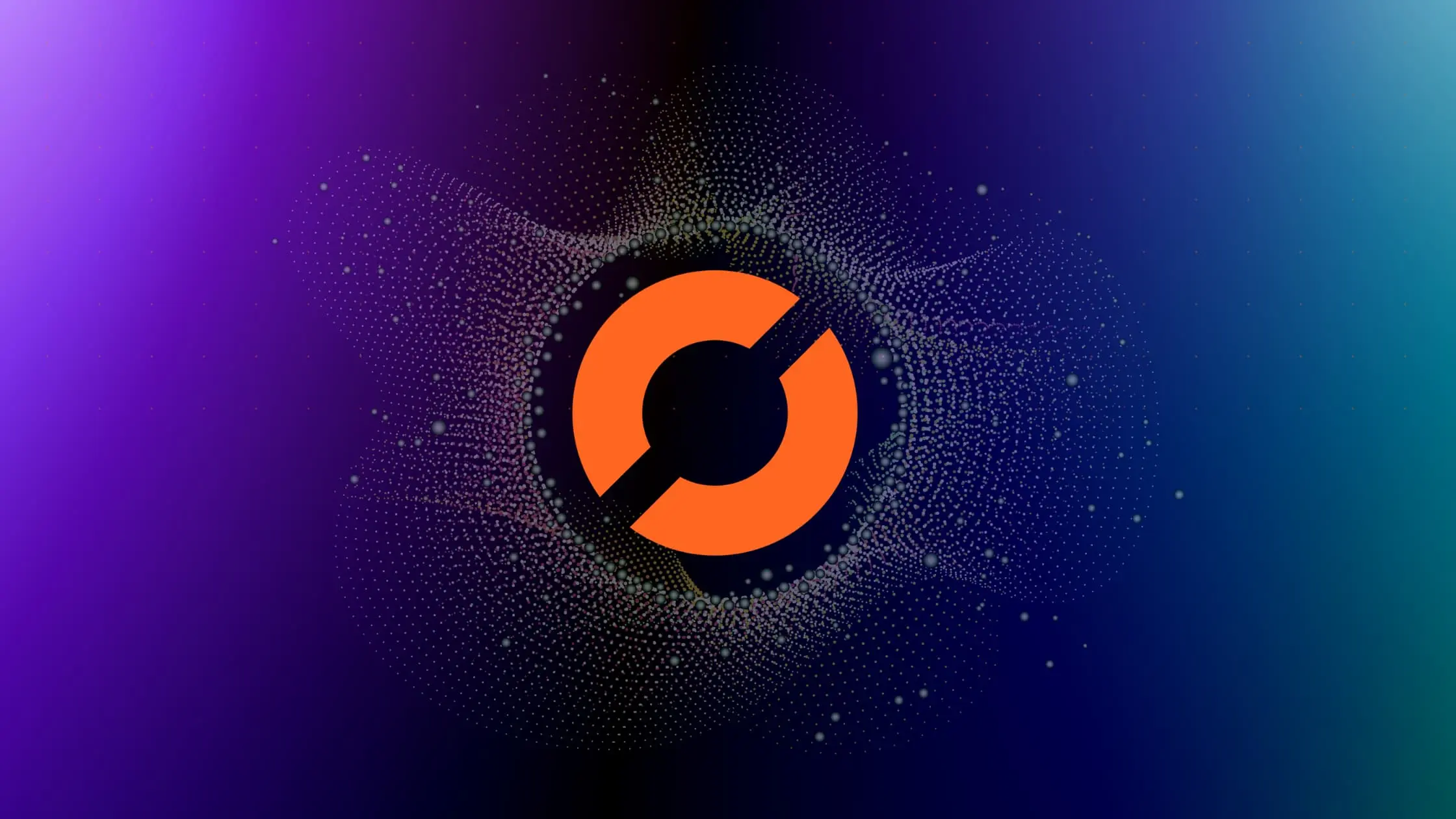
As a data scientist, you’ve probably come across the need to replace values in a pandas DataFrame based on certain conditions. This is a common task when working with real-world datasets, where you may need to clean and preprocess the data before analysis. In this article, we’ll explore how to perform conditional replacement in pandas and provide some examples to demonstrate its usefulness.
Table of Contents
- What is Conditional Replacement?
- How to Perform Conditional Replacement in Pandas
- Best Practices for Conditional Replacement
- Common Errors and How to Handle Them
- Conclusion
What is Conditional Replacement?
Conditional replacement is the process of replacing values in a DataFrame based on certain conditions. For example, you may want to replace all negative values in a column with zero, or replace all occurrences of a particular string with another string. This can be done using pandas' replace
method, which allows you to specify the value to replace and the replacement value based on a condition.
How to Perform Conditional Replacement in Pandas
To perform conditional replacement in pandas, you can use the replace
method on a DataFrame or a Series object. The replace
method takes two arguments: the value to replace and the replacement value. You can also specify a condition using a boolean expression or a callable function.
Here’s the basic syntax:
df.replace(to_replace, value=None, inplace=False, limit=None, regex=False, method='pad')
Let’s break down each argument:
to_replace
: The value or values to replace. This can be a scalar value, a list of values, a dictionary of values, a regular expression, or a callable function.value
: The replacement value or values. This can be a scalar value, a list of values, or a dictionary of values.inplace
: Whether to modify the DataFrame in place or return a new DataFrame with the replacements.limit
: The maximum number of replacements to make.regex
: Whether to interpretto_replace
andvalue
as regular expressions.method
: The method to use when replacing values. The default is'pad'
, which fills forward any missing values.
Let’s see some examples to understand how to use this method.
Example 1: Replace Negative Values with Zero
Suppose we have a DataFrame with some negative values in a column, and we want to replace them with zero. Here’s how we can do it:
import pandas as pd
import numpy as np
df = pd.DataFrame({'A': [1, 2, -3, 4, -5]})
print("before\n")
print(df)
df['A'] = np.where(df['A'] < 0, 0, df['A'])
print("\nafter\n")
print(df)
Output:
before
A
0 1
1 2
2 -3
3 4
4 -5
after
A
0 1
1 2
2 0
3 4
4 0
In this example, we use the NumPy where
function to replace the negative values with zero. The where
function takes a boolean condition and two values, and returns the second value where the condition is true and the first value where it’s false. In this case, we check if the value in column 'A'
is less than zero, and replace it with zero if it is.
Example 2: Replace String Values with Another String
Suppose we have a DataFrame with some string values in a column, and we want to replace them with another string. Here’s how we can do it:
df = pd.DataFrame({'A': ['foo', 'bar', 'baz']})
print("before\n")
print(df)
df['A'].replace({'foo': 'qux', 'bar': 'quux'}, inplace=True)
print("\nafter\n")
print(df)
In this example, we use a dictionary to specify the replacements. The keys of the dictionary are the values to replace, and the values are the replacement values. We set the inplace
parameter to True
to modify the DataFrame in place.
Output:
before
A
0 foo
1 bar
2 baz
after
A
0 qux
1 quux
2 baz
Example 3: Replace Values Based on a Function
Suppose we have a DataFrame with some values in a column, and we want to replace them based on a function. Here’s how we can do it:
df = pd.DataFrame({'A': [1, 2, 3, 4, 5]})
print("before\n")
print(df)
def replace_func(x):
if x % 2 == 0:
return x * 2
else:
return x
df['A'] = df['A'].apply(replace_func)
print("\nafter\n")
print(df)
In this example, we define a function replace_func
that takes a value x
and returns a replacement value based on a condition. We use the apply
method to apply this function to each value in column 'A'
.
Output:
before
A
0 1
1 2
2 3
3 4
4 5
after
A
0 1
1 4
2 3
3 8
4 5
Best Practices for Conditional Replacement
To ensure efficient and readable code, consider the following best practices:
Use Vectorized Operations for Large Datasets
Leverage vectorized operations like numpy.where
for improved performance with large datasets.
Leverage Method Chaining for Readability
Use method chaining to enhance code readability, making it easier to understand and maintain.
Consider Performance Implications
Evaluate the performance characteristics of each method and choose the one that aligns with the specific requirements of your analysis.
Common Errors and How to Handle Them
Despite the versatility of Pandas, data scientists often encounter common errors when performing conditional replacement. Let’s address some of these issues and their solutions:
Mismatched Dimensions
Error: "ValueError: shape mismatch"
Solution: Ensure that the dimensions of the arrays or DataFrames involved in conditional replacement operations match appropriately.
Incorrect Data Types
Error: "TypeError: '>' not supported between instances of 'str' and 'int'"
Solution: Validate and convert data types as needed to ensure compatibility with the specified conditions.
Unintended Side Effects
Error: Unexpected modifications to unrelated columns or rows.
Solution: Double-check the conditions and indices to avoid unintended side effects. Use caution when chaining multiple operations.
Conclusion
Conditional replacement is a useful technique in data cleaning and preprocessing. In this article, we’ve explored how to perform conditional replacement in pandas using the replace
method, and provided some examples to demonstrate its usefulness. By mastering this technique, you can make your data analysis more efficient and accurate.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.