Coloring Cells in Pandas A Guide for Data Scientists
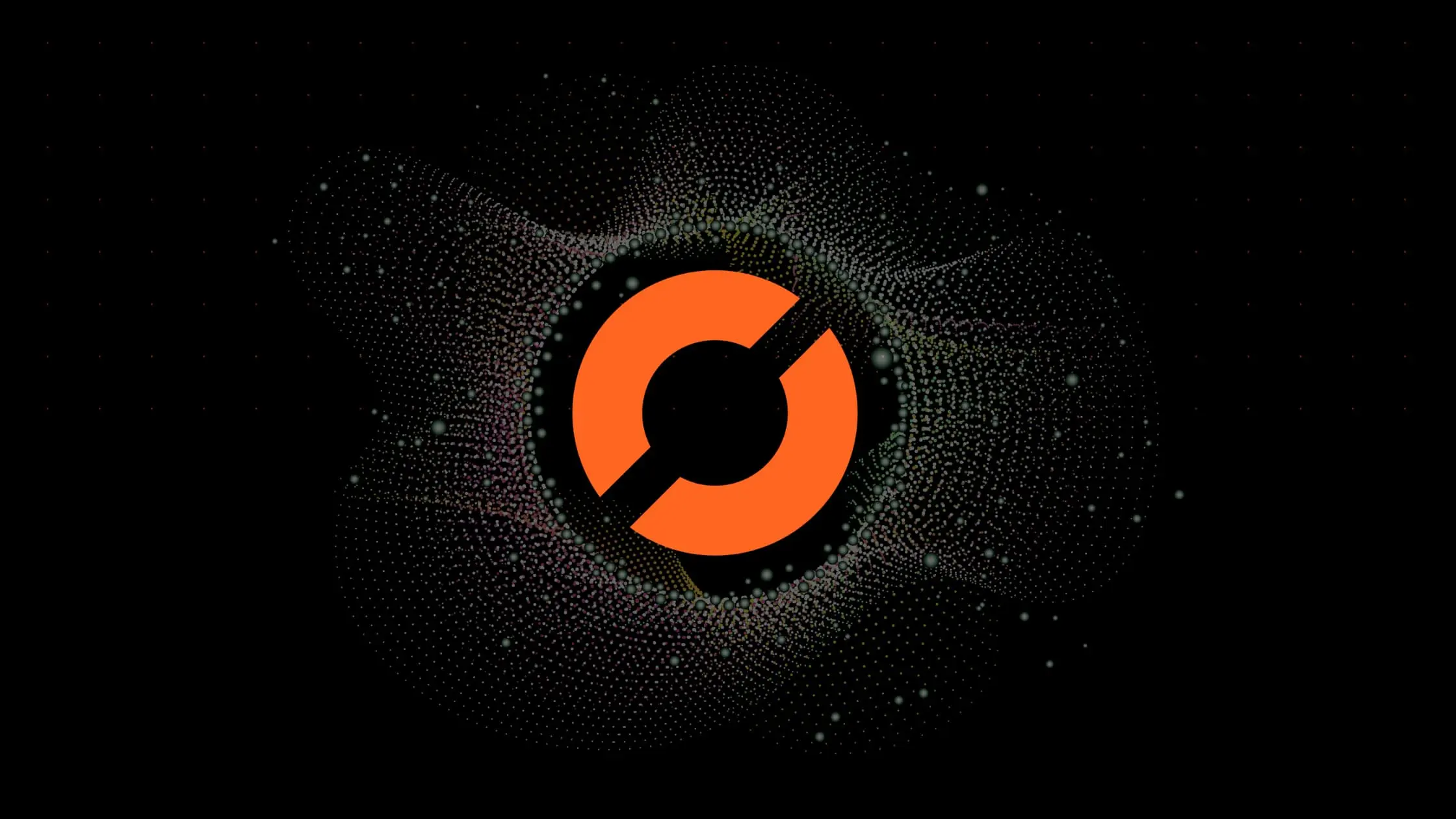
Pandas is a popular data manipulation library in Python that provides powerful tools for data manipulation and analysis. One of the key features of Pandas is the ability to color cells in a DataFrame or Series based on their values. This feature is particularly useful when you need to highlight important information or visualize patterns in your data.
In this post, we will go over the basics of coloring cells in Pandas and demonstrate some examples of how to use it effectively.
Table of Contents
- Introduction
- What is Cell Coloring in Pandas?
- How to Color Cells in Pandas
- Best Practices
- Common Errors
- Conclusion
What is Cell Coloring in Pandas?
Cell coloring in Pandas refers to the process of changing the background color or font color of a cell in a DataFrame or Series based on its value. This can be done using the style
attribute of a Pandas DataFrame or Series.
The style
attribute is a powerful tool that allows you to apply various formatting options to the cells in your DataFrame or Series. This includes changing the background color, font color, font size, and font style, among other things.
How to Color Cells in Pandas
To color cells in Pandas, you first need to create a DataFrame or Series. For this example, we will create a simple DataFrame containing the scores of five students in three different subjects:
import pandas as pd
data = {'Math': [80, 90, 70, 60, 85],
'Science': [85, 75, 90, 65, 80],
'English': [70, 80, 75, 90, 85]}
df = pd.DataFrame(data, index=['Alice', 'Bob', 'Charlie', 'David', 'Eve'])
This will create a DataFrame that looks like this:
Math Science English
Alice 80 85 70
Bob 90 75 80
Charlie 70 90 75
David 60 65 90
Eve 85 80 85
Now that we have a DataFrame, we can use the style
attribute to apply cell coloring based on the values in the DataFrame.
Basic Cell Coloring
The simplest way to color cells in Pandas is to use the background-color
property of the style
attribute. This allows you to change the background color of the cells based on their values.
For example, to highlight all the cells in the DataFrame that have a value greater than 80, you can use the following code:
def highlight_greater_than_80(val):
"""
Takes a scalar and returns a string with
the css property 'background-color: yellow' for
values greater than 80, black otherwise.
"""
color = 'yellow' if val > 80 else 'black'
return f'background-color: {color}'
df.style.apply(lambda x: x.map(highlight_greater_than_80), axis=None)
This will highlight all the cells that have a value greater than 80 in yellow, as shown below:
Advanced Cell Coloring
In addition to basic cell coloring, Pandas also provides several advanced options for cell coloring. These include:
Gradient coloring: This allows you to color cells based on a gradient scale, such as from red to green or from light to dark.
Bar charts: This allows you to create bar charts inside the cells of a DataFrame based on their values.
Heatmaps: This allows you to create heatmaps based on the values in the DataFrame.
Here is an example of how to use gradient coloring in Pandas:
def gradient_color(val):
"""
Takes a scalar and returns a string with
the css property 'background-color: red' for
values less than 70, green for values greater
than 90, and a gradient in between for the
values in between.
"""
r = int(255 * (1 - (val - 70) / (90 - 70)))
g = int(255 * ((val - 70) / (90 - 70)))
b = 0
return f'background-color: rgb({r},{g},{b})'
df.style.apply(lambda x: x.map(gradient_color), axis=None)
This will color the cells in the DataFrame based on a gradient, with values less than 70 in red, values greater than 90 in green, and a gradient in between for the values in between.
Conditional Formatting
Another powerful feature of cell coloring in Pandas is conditional formatting. This allows you to apply different formatting options to cells based on their values.
For example, you can highlight the maximum value in each row like this:
def highlight_max(s):
"""
Takes a Series s and returns a Series with
the css property 'background-color: yellow'
for the maximum value in each row.
"""
is_max = s == s.max()
return ['background-color: yellow' if v else '' for v in is_max]
df.style.apply(highlight_max, axis=1)
This will highlight the maximum value in each row in yellow.
Best Practices
Useful Visualization: Ensure that cell coloring adds meaningful value to your analysis or presentation. Don’t use it excessively or inappropriately, as it might distract from the actual insights in your data.
Consistent Color Schemes: If you are using color to convey specific meanings, maintain a consistent color scheme across your visualizations. This helps in creating a cohesive and understandable representation of the data.
Documentation: Clearly document the color-coding conventions you use, especially if your code is meant to be shared or if others will be interpreting your visualizations. This documentation can be in the form of comments in the code or a separate document.
Consider Accessibility: Be mindful of color choices for users with color vision deficiencies. Ensure that your color choices are accessible and that information is not solely conveyed through color.
Common Errors:
Data Type Mismatch: Ensure that the data types in your DataFrame or Series are compatible with the conditions specified in your coloring functions. Mismatched data types may result in errors or undesired outcomes.
Overlapping Styling: Avoid overlapping styles that might conflict with each other. If multiple styling functions are applied, make sure they complement each other to provide a coherent visualization.
Neglecting Edge Cases: Consider edge cases when defining your coloring functions. For instance, if your data includes NaN values, account for them in your functions to prevent errors or unexpected behavior.
Performance Considerations: Be cautious with large datasets, as extensive use of cell coloring can impact performance. Test your code with different sizes of datasets to ensure that it remains responsive.
Limited Browser Compatibility: Keep in mind that some advanced styling options may not be supported in all environments or browsers. Test your visualizations across different platforms to ensure consistent rendering.
Conclusion
In this post, we have shown you how to color cells in Pandas based on their values. This is a powerful tool that allows you to highlight important information or visualize patterns in your data.
We have covered the basics of cell coloring in Pandas, including how to use basic cell coloring and advanced options such as gradient coloring and conditional formatting.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.