Changing Specific Values in a Numpy Array: A Guide
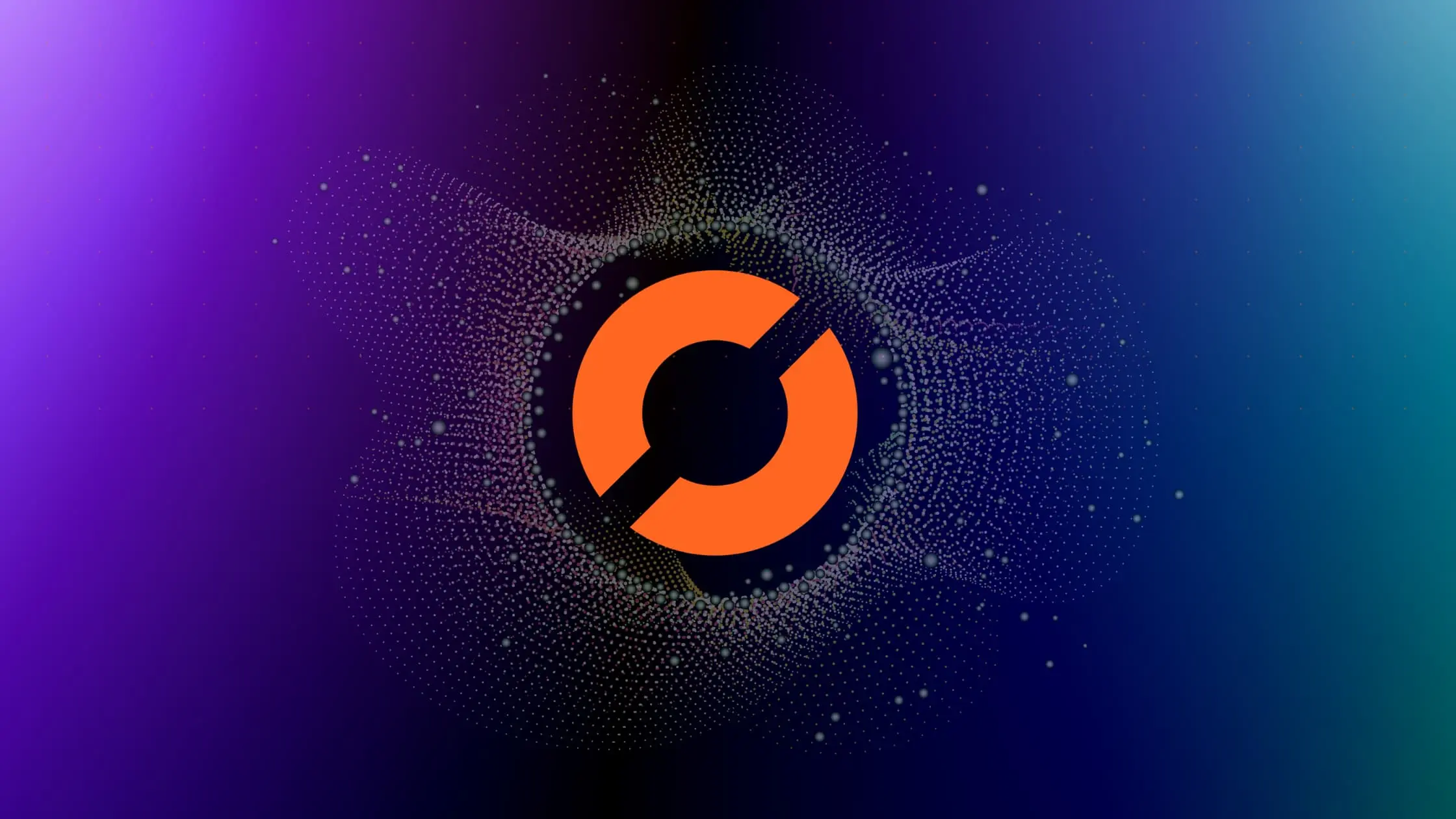
Numpy is a powerful library in Python that provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays. In this blog post, we’ll delve into a specific use case: changing values between two specific values in a Numpy array. This is a common task in data preprocessing, and understanding how to do it efficiently can save you a lot of time.
What You’ll Learn
By the end of this post, you’ll be able to:
- Understand the basics of Numpy arrays
- Identify and change specific values in a Numpy array
- Use Boolean indexing to change values between two specific values
Prerequisites
To follow along, you should have:
- Basic knowledge of Python
- Familiarity with Numpy library
- An installed version of Python and Numpy
Understanding Numpy Arrays
Numpy arrays are the core of the Numpy library. They are similar to lists in Python, but provide more efficient storage and data operations as the size of the data grows. Numpy arrays can be created using the numpy.array()
function.
import numpy as np
# Create a Numpy array
arr = np.array([1, 2, 3, 4, 5])
print(arr)
Identifying and Changing Specific Values
To change a specific value in a Numpy array, you can use indexing. This is similar to how you would change a value in a Python list.
# Change the second value in the array
arr[1] = 10
print(arr)
Output:
[ 1 10 3 4 5]
Changing Values Between Two Specific Values
To change values between two specific values in a Numpy array, you can use Boolean indexing. This is a type of indexing that allows you to select elements in an array using conditions.
# Change all values between 2 and 4 to 0
arr[(arr > 2) & (arr < 4)] = 0
print(arr)
In the above code, (arr > 2) & (arr < 4)
creates a Boolean array of the same shape as arr
, where each element is True
if the corresponding element in arr
is between 2 and 4, and False
otherwise. The =
operator then changes all True
elements to 0.
Output:
[1 2 0 4 5]
Common Errors and Solutions
1. Error: Incorrect Syntax in Boolean Indexing
# Incorrect Boolean indexing syntax
arr[arr > 2 and arr < 4] = 0
Solution: Use parentheses to ensure correct order of operations.
# Correct Boolean indexing syntax
arr[(arr > 2) & (arr < 4)] = 0
2. Error: Changing Values in a Non-NumPy Array
# Attempting to use NumPy operations on a regular Python list
python_list = [1, 2, 3, 4, 5]
python_list[(python_list > 2) & (python_list < 4)] = 0
Solution: Convert the Python list to a NumPy array before applying NumPy operations.
# Convert Python list to NumPy array
np_array = np.array(python_list)
np_array[(np_array > 2) & (np_array < 4)] = 0
Best Practices
1. Ensure Proper Data Type
Always ensure that the data type of your NumPy array is appropriate for the operations you are performing. Incorrect data types can lead to unexpected results or errors.
2. Validate Conditions
Before applying Boolean indexing, double-check your conditions to avoid unintended modifications. Print the Boolean array first to verify that it selects the desired elements.
3. Create a Copy for Reference
Create a copy of your original array before making changes to easily reference the initial state if needed.
# Create a copy of the original array
original_arr = np.copy(arr)
4. Use Vectorized Operations
Leverage NumPy’s vectorized operations for efficiency and readability. These operations are optimized for large datasets and can significantly improve performance.
# Use vectorized operation to change values between 2 and 4 to 0
arr[(arr > 2) & (arr < 4)] = 0
Conclusion
Changing values between two specific values in a Numpy array is a common task in data preprocessing. By using Boolean indexing, you can do this efficiently and in a way that is easy to understand. Remember to always be careful when changing values in an array, as it can alter your data in ways that may not be immediately obvious.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.