Binning a Column with Python Pandas
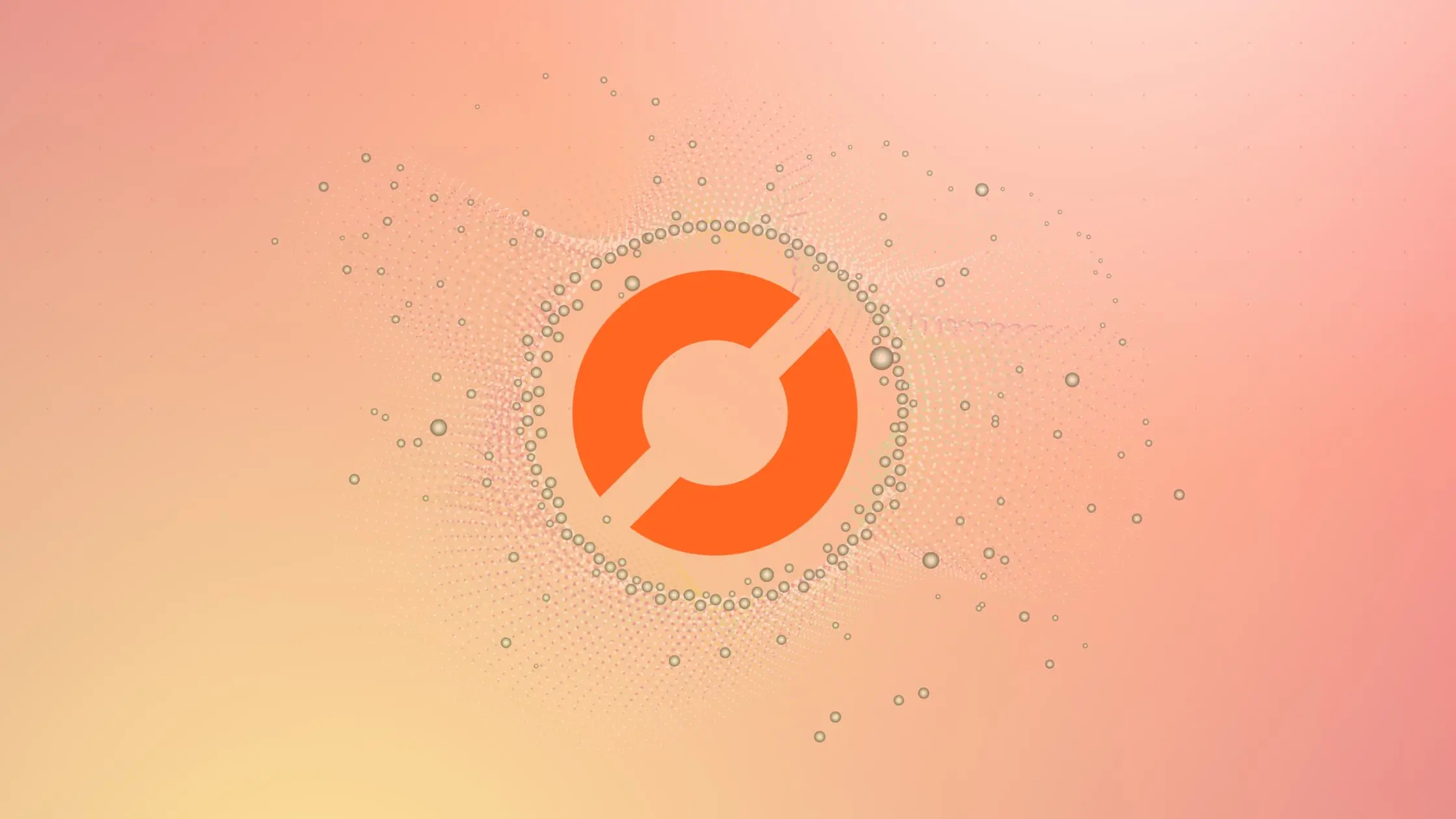
If you work with data, you might have come across a scenario where you need to group a continuous variable into a set of discrete intervals. This process is called binning, and it can help you simplify your analysis and gain insights from the data.
In this post, we will explore how to bin a column using Python Pandas, a popular data manipulation library. We will cover what binning is, why it is useful, and how to implement it using Pandas.
Table of Contents
What is Binning?
Binning is the process of dividing a continuous variable into a set of intervals or bins. For example, suppose you have a column of ages in a dataset. Binning can help you group these ages into categories such as “0-10”, “11-20”, “21-30”, and so on. This grouping can simplify your analysis and help you identify patterns and trends in the data.
Binning is often used in data preprocessing and feature engineering. It can also be useful in data visualization, where it can help you create more informative and insightful plots.
Why is Binning Useful?
Binning can be useful for several reasons:
Simplification: Binning can help you simplify a continuous variable by reducing the number of unique values. This can make the data easier to analyze and interpret.
Categorical Encoding: Binning can be used to encode a continuous variable as a categorical variable. This can be useful for machine learning models that require categorical input.
Outlier Detection: Binning can help you identify outliers by grouping extreme values into separate bins.
How to Bin a Column with Pandas
To bin a column using Pandas, we can use the cut()
function. The cut()
function takes a continuous variable and a set of bin edges and returns a categorical variable representing the bin intervals.
Here’s an example of how to bin a column using Pandas:
import pandas as pd
# create a sample dataframe
data = {'age': [18, 25, 30, 35, 40, 45, 50, 55, 60, 65]}
df = pd.DataFrame(data)
# define the bin edges
bins = [0, 20, 40, 60, 80]
# bin the age column
df['age_bin'] = pd.cut(df['age'], bins)
print(df)
Output:
age age_bin
0 18 (0, 20]
1 25 (20, 40]
2 30 (20, 40]
3 35 (20, 40]
4 40 (20, 40]
5 45 (40, 60]
6 50 (40, 60]
7 55 (40, 60]
8 60 (40, 60]
9 65 (60, 80]
In this example, we created a sample dataframe with a column of ages. We then defined the bin edges as [0, 20, 40, 60, 80]
, which creates four bins: 0-20
, 20-40
, 40-60
, and 60-80
. Finally, we applied the cut()
function to the age column and stored the result in a new column called age_bin
.
The output shows the original age values and their corresponding bins. As you can see, the cut()
function has grouped the ages into the appropriate intervals.
Customizing Binning with Pandas
The cut()
function provides several options that allow you to customize the binning process. Here are a few examples:
Specify Bin Labels
By default, the cut()
function returns a categorical variable with labels corresponding to the bin edges. However, you can specify custom labels using the labels
parameter:
bins = [0, 20, 40, 60, 80]
labels = ['young', 'middle-aged', 'old', 'very-old']
df['age_bin'] = pd.cut(df['age'], bins, labels=labels)
print(df)
Output:
age age_bin
0 18 young
1 25 middle-aged
2 30 middle-aged
3 35 middle-aged
4 40 middle-aged
5 45 old
6 50 old
7 55 old
8 60 old
9 65 very-old
In this example, we specified custom labels for each bin using the labels
parameter. The resulting categorical variable now has more descriptive labels.
Bin by Quantile
Instead of specifying bin edges manually, you can also bin a column by quantile using the qcut()
function. The qcut()
function takes a continuous variable and a number of quantiles and returns a categorical variable representing the quantile intervals.
df['age_bin'] = pd.qcut(df['age'], q=4, labels=False)
print(df)
Output:
age age_bin
0 18 0
1 25 0
2 30 1
3 35 1
4 40 2
5 45 2
6 50 3
7 55 3
8 60 3
9 65 3
In this example, we used the qcut()
function to bin the age column into four quantiles. The resulting categorical variable now represents the quantile intervals, with values ranging from 0 to 3.
Conclusion
Binning is a useful technique for grouping continuous variables into discrete intervals. It can help simplify analysis and gain insights from the data. In this post, we explored how to bin a column using Python Pandas, a popular data manipulation library. We covered what binning is, why it is useful, and how to implement it using Pandas. We also looked at some options for customizing the binning process, such as specifying custom labels and binning by quantile.
By mastering this technique, you can improve your data preprocessing and feature engineering skills, and create more informative and insightful visualizations.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.