Applying Functions to Each Element in a 2D Numpy Array: A Guide
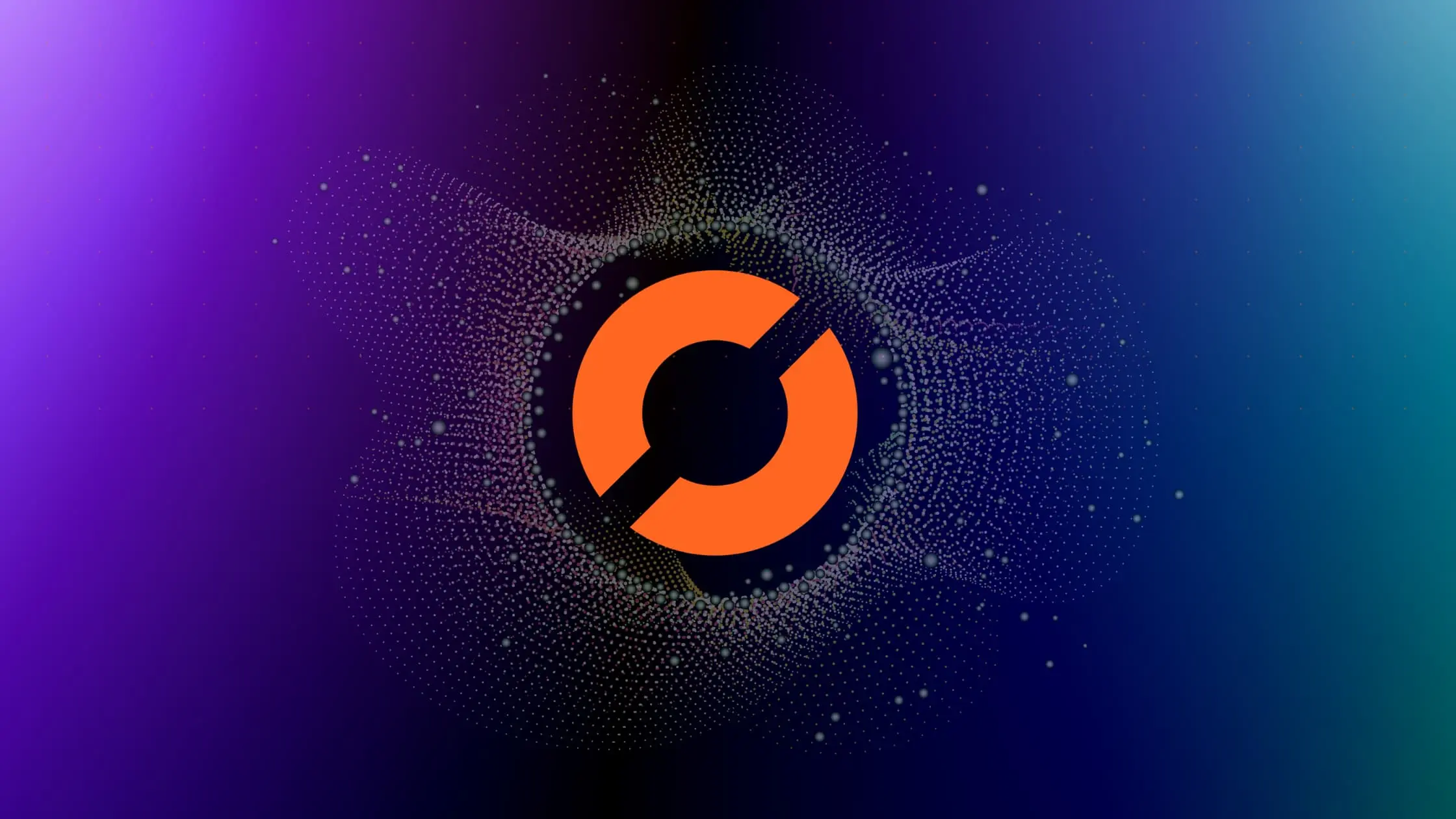
Numpy, a fundamental package for scientific computing in Python, is a powerful tool for data scientists. It provides a high-performance multidimensional array object and tools for working with these arrays. In this blog post, we’ll explore how to apply a function or map values to each element in a 2D Numpy array, a common task in data science.
Table of Contents
- Why Use Numpy?
- Creating a 2D Numpy Array
- Applying Functions to Each Element in a 2D Numpy Array
- Common Errors and Solutions
- Conclusion
Why Use Numpy?
Numpy arrays are more efficient than Python lists when it comes to numerical operations. They provide a host of functions that allow for mathematical manipulation of arrays, making it a go-to tool for data scientists.
Creating a 2D Numpy Array
Before we dive into applying functions, let’s first create a 2D Numpy array. Here’s how you can do it:
import numpy as np
# Create a 2D array
array_2d = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
print(array_2d)
Output:
[[1 2 3]
[4 5 6]
[7 8 9]]
Applying Functions to Each Element in a 2D Numpy Array
There are several ways to apply a function or map values to each element in a 2D Numpy array. We’ll explore three methods: using np.vectorize()
, np.apply_along_axis()
, and list comprehension.
Method 1: Using np.vectorize()
np.vectorize()
is a class that generalizes a function to handle input arrays. It’s essentially a for loop over the elements and supports broadcasting and multiple input arrays.
# Define a function
def add_five(x):
return x + 5
# Vectorize the function
vectorized_add_five = np.vectorize(add_five)
# Apply the function to the 2D array
new_array = vectorized_add_five(array_2d)
print(new_array)
Output:
[[ 6 7 8]
[ 9 10 11]
[12 13 14]]
Method 2: Using np.apply_along_axis()
np.apply_along_axis()
applies a function to 1-D slices along the given axis. This method is more suitable for more complex functions that cannot be vectorized.
# Define a function
def multiply_by_two(x):
return x * 2
# Apply the function to the 2D array
new_array = np.apply_along_axis(multiply_by_two, 0, array_2d)
print(new_array)
Output:
[[ 2 4 6]
[ 8 10 12]
[14 16 18]]
Method 3: Using List Comprehension
While not as efficient as the Numpy methods, list comprehension is a Pythonic way to apply a function to each element in a 2D array.
# Define a function
def subtract_three(x):
return x - 3
# Apply the function to the 2D array
new_array = np.array([[subtract_three(i) for i in row] for row in array_2d])
print(new_array)
Output:
[[-2 -1 0]
[ 1 2 3]
[ 4 5 6]]
Common Errors and Solutions
Incorrect Array Shape: Attempting to perform operations on arrays of mismatched shapes. Ensure that the arrays involved in operations have compatible shapes. Use
array.shape
to check dimensions and reshape arrays if necessary.Function Compatibility: Using functions that are not compatible with array operations. Ensure that the functions used are designed to work with Numpy arrays. If necessary, modify the function or use Numpy’s built-in functions.
Broadcasting Issues: Misunderstanding how broadcasting works, leading to unexpected results. Familiarize yourself with Numpy’s broadcasting rules. Always verify the shapes of arrays before performing operations that rely on broadcasting.
Memory Overhead with np.vectorize(): Using
np.vectorize()
excessively, leading to high memory usage. Understand thatnp.vectorize()
is not a performance optimization tool. Use it only when necessary, and prefer built-in Numpy operations.Axis Confusion in np.apply_along_axis(): Misinterpreting the axis parameter in
np.apply_along_axis()
. Clearly understand the axis parameter. Axis 0 typically refers to columns, and axis 1 to rows, in a 2D array.Type Errors: Function operations leading to type mismatches or unexpected type conversions. Ensure consistent data types across operations. Use array.dtype to check and
array.astype()
to convert types if necessary.Inefficient List Comprehension: Overusing list comprehension for large arrays, leading to inefficiency. Use list comprehension sparingly, especially for large datasets. Prefer Numpy’s vectorized operations for better performance.
Misuse of apply_along_axis for Simple Operations: Using
np.apply_along_axis()
for operations that can be vectorized. Before usingnp.apply_along_axis()
, check if a vectorized Numpy function exists for your task.
Conclusion
Applying functions to each element in a 2D Numpy array is a common task in data science. Whether you’re performing simple arithmetic operations or applying more complex functions, Numpy provides efficient ways to accomplish this task. Remember to choose the method that best suits your needs and the complexity of your function.
In this blog post, we’ve explored three methods: np.vectorize()
, np.apply_along_axis()
, and list comprehension. Each has its strengths and weaknesses, but all are powerful tools in the data scientist’s toolkit.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.