Applying a Function Along a Numpy Array: A Guide for Data Scientists
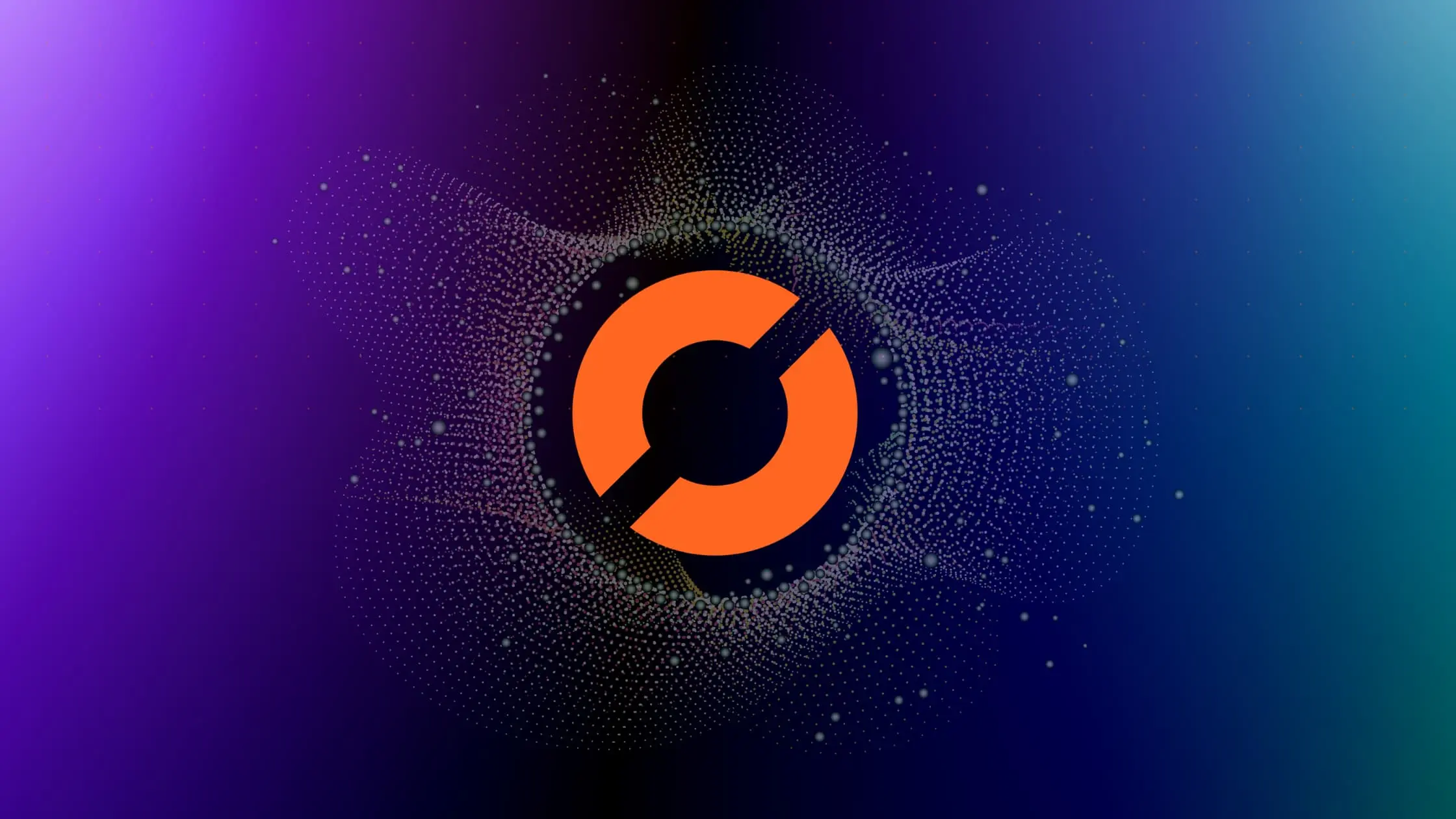
Numpy, a fundamental package for scientific computing in Python, is a powerful tool for data scientists. One of its most useful features is the ability to apply a function along an array. This post will guide you through the process, ensuring you can leverage this feature to optimize your data science projects.
What is Numpy?
Numpy, short for ‘Numerical Python’, is a library for the Python programming language, adding support for large, multi-dimensional arrays and matrices, along with a large collection of high-level mathematical functions to operate on these arrays.
Why Apply a Function Along a Numpy Array?
Applying a function along a Numpy array allows you to perform an operation on each element in the array. This is particularly useful when you need to perform the same operation on multiple data points, such as normalizing data or applying a mathematical transformation.
How to Apply a Function Along a Numpy Array
Let’s dive into the process of applying a function along a Numpy array.
Step 1: Import Numpy
First, you need to import the Numpy library. If you haven’t installed it yet, you can do so using pip:
pip install numpy
Then, import it into your Python script:
import numpy as np
Step 2: Define Your Function
Next, define the function you want to apply to the array. For this example, let’s use a simple function that squares each number:
def square(x):
return x**2
Step 3: Create Your Numpy Array
Now, create the Numpy array you want to apply the function to. Here’s an example:
arr = np.array([1, 2, 3, 4, 5])
Step 4: Apply the Function
Finally, use the np.vectorize()
function to apply your function to the array. This function takes as input the function to be applied and returns a new function that can accept Numpy arrays as input:
vfunc = np.vectorize(square)
result = vfunc(arr)
print(result)
Output:
[ 1 4 9 16 25]
The result
array now contains the square of each number in the original array.
Optimizing the Process
While np.vectorize()
is a convenient way to apply a function to a Numpy array, it’s not the most efficient. For better performance, you can use Numpy’s universal functions (ufuncs), which are implemented in C and are much faster.
Here’s how you can use a ufunc to square each number in an array:
result = np.square(arr)
Output:
[ 1 4 9 16 25]
Conclusion
Applying a function along a Numpy array is a powerful technique that can help you manipulate and analyze your data more effectively. Whether you’re normalizing data, applying mathematical transformations, or performing other operations, this feature of Numpy is sure to be a valuable tool in your data science toolkit.
While np.vectorize()
is a convenient way to apply a function to an array, for better performance, consider using Numpy’s universal functions. They’re faster and more efficient, helping you get the most out of your data science projects.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.