Append DataFrames with Different Column Names in Pandas
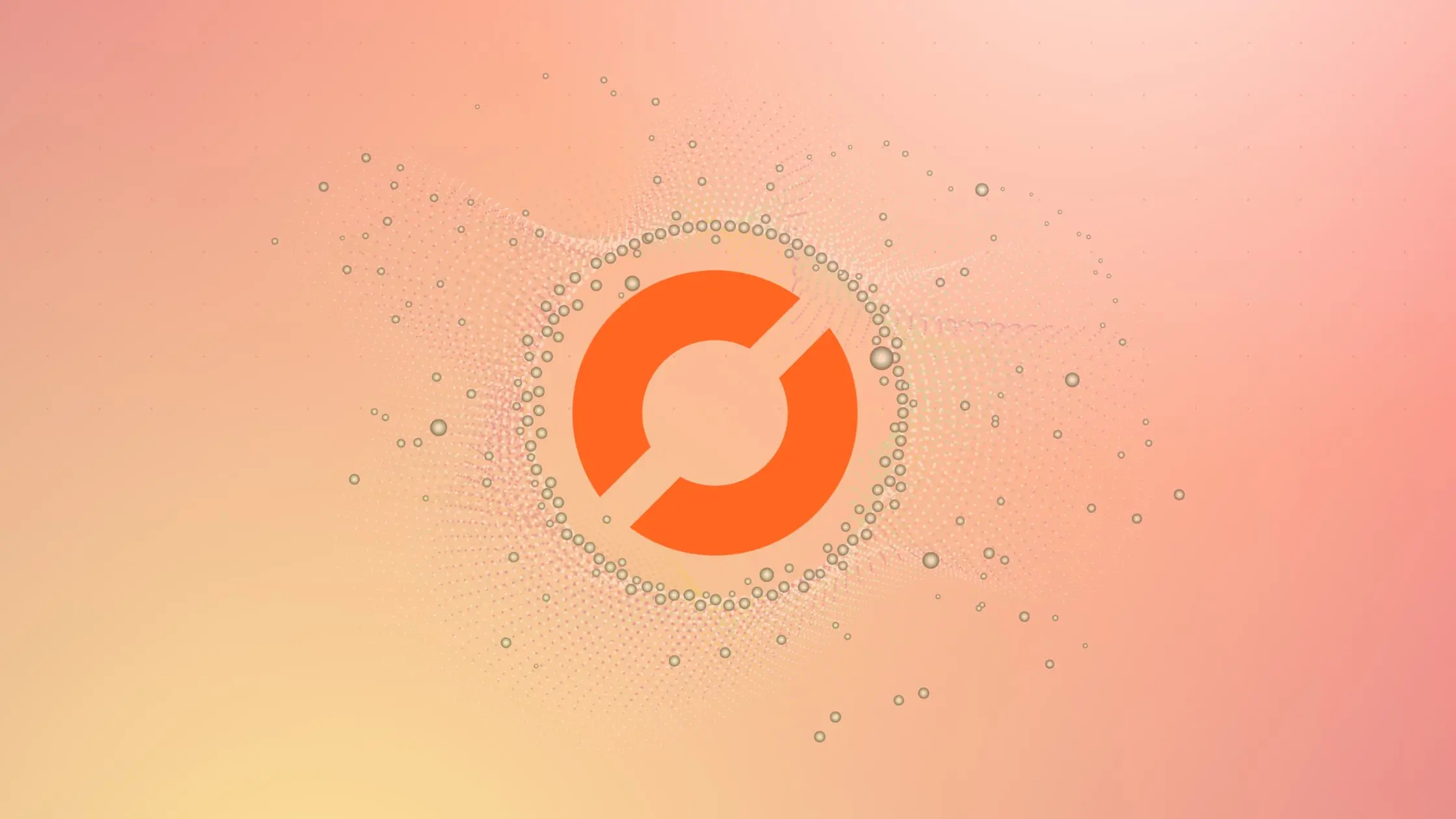
Pandas is a powerful data manipulation library in Python that provides flexible and efficient data structures. One common operation in data analysis is appending or combining dataframes. However, what if the dataframes have different column names? In this blog post, we’ll explore how to append dataframes with different column names in Pandas.
Table of Contents
- Introduction
- Appending DataFrames with Different Column Names
- Common Errors and Troubleshooting
- Conclusion
Introduction to Appending DataFrames
Appending dataframes is a common operation in data analysis. It involves combining two or more dataframes vertically, i.e., adding rows from one dataframe to another. In Pandas, you can use the append()
function to append dataframes.
df1.append(df2)
However, this operation assumes that the dataframes have the same column names. If the column names are different, the append()
function will result in NaN values for the columns that do not exist in the original dataframe.
Appending DataFrames with Different Column Names
Let’s say we have two dataframes with different column names:
import pandas as pd
df1 = pd.DataFrame({
'A': ['A0', 'A1', 'A2', 'A3'],
'B': ['B0', 'B1', 'B2', 'B3'],
'C': ['C0', 'C1', 'C2', 'C3'],
'D': ['D0', 'D1', 'D2', 'D3'],
})
df2 = pd.DataFrame({
'E': ['E4', 'E5', 'E6', 'E7'],
'F': ['F4', 'F5', 'F6', 'F7'],
'G': ['G4', 'G5', 'G6', 'G7'],
'H': ['H4', 'H5', 'H6', 'H7'],
})
If we try to append df2
to df1
using the append()
function, we’ll get NaN values for the columns that do not exist in df1
.
result = df1.append(df2)
print(result)
Output:
A B C D E F G H
0 A0 B0 C0 D0 NaN NaN NaN NaN
1 A1 B1 C1 D1 NaN NaN NaN NaN
2 A2 B2 C2 D2 NaN NaN NaN NaN
3 A3 B3 C3 D3 NaN NaN NaN NaN
0 NaN NaN NaN NaN E4 F4 G4 H4
1 NaN NaN NaN NaN E5 F5 G5 H5
2 NaN NaN NaN NaN E6 F6 G6 H6
3 NaN NaN NaN NaN E7 F7 G7 H7
To append dataframes with different column names, we need to rename the columns of the second dataframe to match the column names of the first dataframe. We can use the rename()
function in Pandas to rename the columns.
df2 = df2.rename(columns={'E': 'A', 'F': 'B', 'G': 'C', 'H': 'D'})
result = df1.append(df2)
print(result)
Output:
A B C D
0 A0 B0 C0 D0
1 A1 B1 C1 D1
2 A2 B2 C2 D2
3 A3 B3 C3 D3
0 E4 F4 G4 H4
1 E5 F5 G5 H5
2 E6 F6 G6 H6
3 E7 F7 G7 H7
Now, df2
has the same column names as df1
, and we can append df2
to df1
without any NaN values.
Common Errors and Troubleshooting
When appending DataFrames with different column names, you may encounter errors. The most common ones include:
- ValueError: Raised when columns are not aligned during the append operation.
- TypeError: Occurs if the DataFrames have incompatible data types in corresponding columns.
To troubleshoot, ensure that the ignore_index parameter is set to True to reindex the resulting DataFrame.
Conclusion
Appending dataframes with different column names in Pandas requires renaming the columns of the second dataframe to match the column names of the first dataframe. This operation is essential in data analysis when you need to combine data from different sources with different column names.
Remember, data manipulation is a crucial part of data analysis, and understanding how to append dataframes with different column names in Pandas can help you handle complex data manipulation tasks more efficiently.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.