Adding New Rows to PySpark DataFrame: A Guide
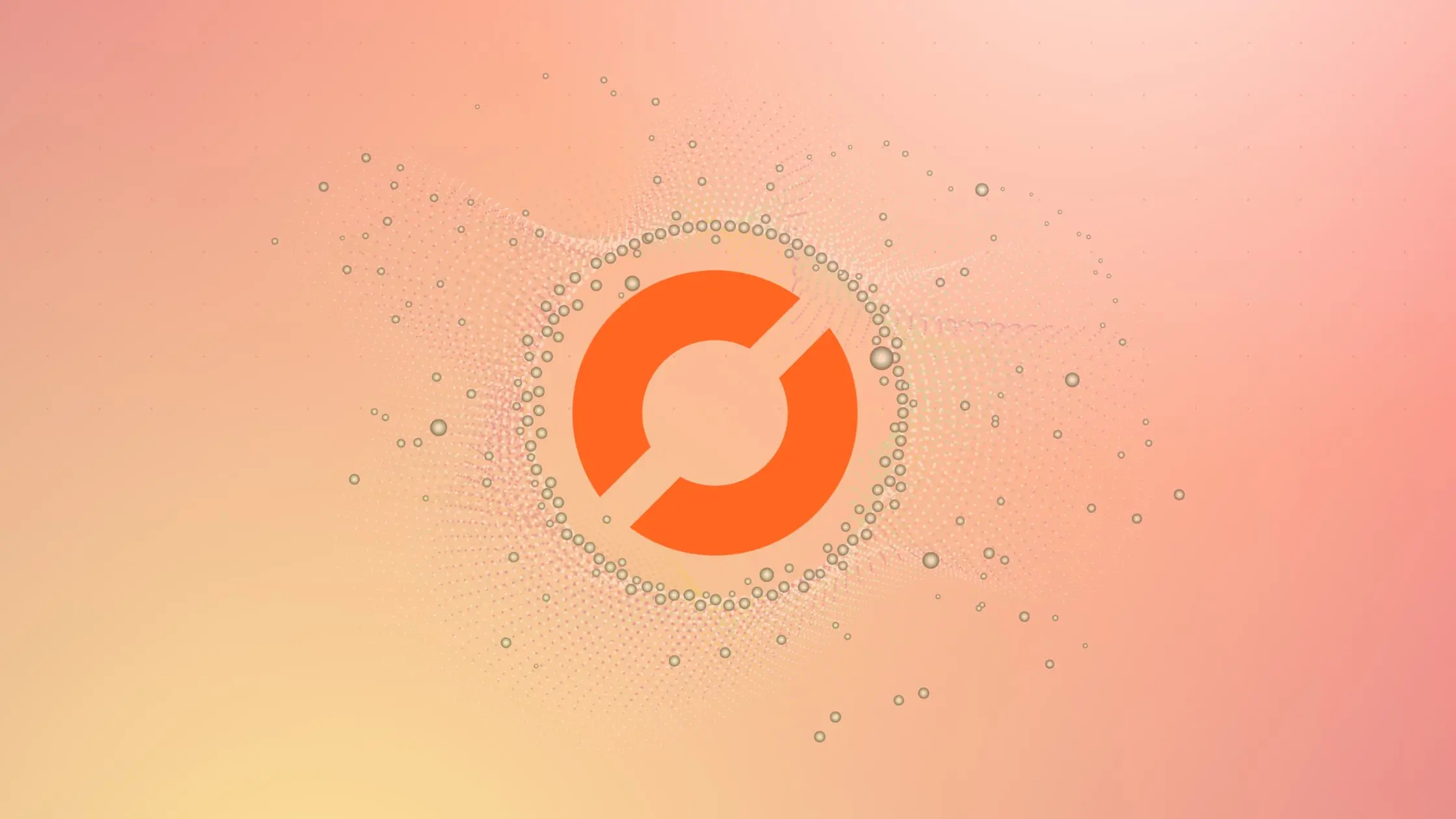
Data manipulation is a crucial aspect of data science. In this blog post, we’ll delve into how to add new rows to a PySpark DataFrame, a common operation that data scientists often need to perform. PySpark, the Python library for Apache Spark, is a powerful tool for large-scale data processing.
Introduction to PySpark DataFrame
PySpark DataFrame is a distributed collection of data organized into named columns. It’s conceptually equivalent to a table in a relational database or a data frame in Python, but with optimizations for speed and functionality under the hood.
Why Add Rows to a DataFrame?
There are numerous reasons why you might want to add new rows to a DataFrame. For instance, you might have new data that you want to append to an existing DataFrame, or you might want to add calculated results as new rows.
Adding Rows to a DataFrame
Let’s dive into the process of adding new rows to a PySpark DataFrame.
Step 1: Import Necessary Libraries
First, we need to import the necessary libraries.
from pyspark.sql import SparkSession
from pyspark.sql import Row
Step 2: Create a SparkSession
Next, we create a SparkSession, which is the entry point to any functionality in Spark.
spark = SparkSession.builder.appName('AddRows').getOrCreate()
Step 3: Create a DataFrame
For this example, let’s create a simple DataFrame.
data = [('James', 'Sales', 3000),
('Michael', 'Sales', 4600),
('Robert', 'Sales', 4100)]
columns = ["Employee", "Department", "Salary"]
df = spark.createDataFrame(data, columns)
df.show()
Output:
+--------+----------+------+
|Employee|Department|Salary|
+--------+----------+------+
| James| Sales| 3000|
| Michael| Sales| 4600|
| Robert| Sales| 4100|
+--------+----------+------+
Step 4: Create a New Row
Now, we’ll create a new row that we want to add to the DataFrame.
new_row = spark.createDataFrame([('Maria', 'Marketing', 4000)], columns)
Step 5: Append the New Row
Finally, we append the new row to the existing DataFrame using the union
method.
df = df.union(new_row)
df.show()
Output:
+--------+----------+------+
|Employee|Department|Salary|
+--------+----------+------+
| James| Sales| 3000|
| Michael| Sales| 4600|
| Robert| Sales| 4100|
| Maria| Marketing| 4000|
+--------+----------+------+
Conclusion
Adding new rows to a PySpark DataFrame is a straightforward process, but it’s a fundamental skill for data scientists working with large-scale data. By mastering this operation, you can manipulate data more effectively and efficiently in PySpark.
PySpark is a powerful tool for data processing, and understanding how to manipulate DataFrames is crucial for data analysis.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.